FuelPHP-완전한 작업 예
이 장에서는 FuelPHP에서 완전한 MVC 기반 BookStore 애플리케이션을 만드는 방법을 배웁니다.
1 단계 : 프로젝트 만들기
다음 명령을 사용하여 FuelPHP에서 "BookStore"라는 새 프로젝트를 만듭니다.
oil create bookstore
2 단계 : 레이아웃 만들기
응용 프로그램에 대한 새 레이아웃을 만듭니다. fuel / app / views / layout.php 위치에 layout.php 파일을 생성하십시오. 코드는 다음과 같습니다.
fuel / app / views / layout.php
<!DOCTYPE html>
<html lang = "en">
<head>
<meta charset = "utf-8">
<meta http-equiv = "X-UA-Compatible" content = "IE = edge">
<meta name = "viewport" content = "width = device-width, initial-scale = 1">
<title><?php echo $title; ?></title>
<!-- Bootstrap core CSS -->
<link href = "/assets/css/bootstrap.min.css" rel = "stylesheet">
<script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js">
</script>
<script src = "/assets/js/bootstrap.min.js"></script>
</head>
<body>
<nav class = "navbar navbar-inverse navbar-fixed-top">
<div class = "container">
<div class = "navbar-header">
<button type = "button" class = "navbar-toggle collapsed"
datatoggle = "collapse" data-target = "#navbar"
aria-expanded = "false" ariacontrols = "navbar">
<span class= "sr-only">Toggle navigation</span>
<span class = "icon-bar"></span>
<span class = "icon-bar"></span>
<span class = "icon-bar"></span>
</button>
<a class = "navbar-brand" href = "#">FuelPHP Sample</a>
</div>
<div id = "navbar" class = "collapse navbar-collapse">
<ul class = "nav navbar-nav">
<li class = "active"><a href = "/book/index">Home</a></li>
<li><a href = "/book/add">Add book</a></li>
</ul>
</div><!--/.nav-collapse -->
</div>
</nav>
<div class = "container">
<div class = "starter-template" style = "padding: 50px 0 0 0;">
<?php echo $content; ?>
</div>
</div><!-- /.container -->
</body>
</html>
여기서는 부트 스트랩 템플릿을 사용하고 있습니다. FuelPHP는 부트 스트랩 템플릿에 대한 최고 수준의 지원을 제공합니다. 제목과 내용이라는 두 가지 변수를 만들었습니다. title은 현재 페이지의 제목을 지정하는 데 사용되며 콘텐츠는 현재 페이지의 세부 정보를 지정하는 데 사용됩니다.
3 단계 : 컨트롤러 생성
새 컨트롤러 인 Controller_Book을 만들어 책을 표시, 추가, 편집 및 삭제합니다. 새 파일 fuel / app / classes / controller / book.php를 만들고 다음 코드를 배치합니다.
fuel / app / classes / controller / book.php
<?php
class Controller_Book extends Controller_Template {
public $template = 'layout';
public function action_index() {
// Create the view object
$view = View::forge('book/index');
// set the template variables
$this->template->title = "Book index page";
$this->template->content = $view;
}
}
여기에서는 템플릿 컨트롤러를 상속하여 북 컨트롤러를 만들고 기본 템플릿을 fuel / app / views / layout.php로 설정했습니다.
4 단계 : 인덱스보기 만들기
폴더를 만들고 fuel / app / views 폴더 아래의보기 디렉토리에 예약하십시오. 그런 다음 책 폴더 안에 index.php 파일을 만들고 다음 코드를 추가하십시오.
fuel / app / views / index.php
<h3>index page</h3>
지금까지 기본적인 북 컨트롤러를 만들었습니다.
5 단계 : 기본 경로 수정
기본 경로를 업데이트하여 애플리케이션의 홈 페이지를 예약 컨트롤러로 설정합니다. 기본 라우팅 구성 파일 인 fuel / app / config / routes.php를 열고 다음과 같이 변경하십시오.
fuel / app / config / routes.php
<?php
return array (
'_root_' => 'book/index', // The default route
'_404_' => 'welcome/404', // The main 404 route
'hello(/:name)?' => array('welcome/hello', 'name' => 'hello'),
);
이제 URL을 요청하면 http : // localhost : 8080 /는 다음과 같이 책 컨트롤러의 색인 페이지를 반환합니다.

6 단계 : 데이터베이스 생성
다음 명령을 사용하여 MySQL 서버에 새 데이터베이스를 만듭니다.
create database tutorialspoint_bookdb
그런 다음 다음 명령을 사용하여 데이터베이스 내부에 테이블을 만듭니다.
CREATE TABLE book (
id INT PRIMARY KEY AUTO_INCREMENT,
title VARCHAR(80) NOT NULL,
author VARCHAR(80) NOT NULL,
price DECIMAL(10, 2) NOT NULL
);
다음 SQL 문을 사용하여 일부 샘플 레코드를 테이블에 삽입합니다.
INSERT
INTO
book(title,
author,
price)
VALUES(
'The C Programming Language',
'Dennie Ritchie',
25.00
),(
'The C++ Programming Language',
'Bjarne Stroustrup',
80.00
),(
'C Primer Plus (5th Edition)',
'Stephen Prata',
45.00
),('Modern PHP', 'Josh Lockhart', 10.00),(
'Learning PHP, MySQL & JavaScript, 4th Edition',
'Robin Nixon',
30.00
)
7 단계 : 데이터베이스 구성
fuel / app / config에있는 데이터베이스 구성 파일 db.php를 사용하여 데이터베이스를 구성하십시오.
fuel / app / config / db.php
<?php
return array (
'development' => array (
'type' => 'mysqli',
'connection' => array (
'hostname' => 'localhost',
'port' => '3306',
'database' => 'tutorialspoint_bookdb',
'username' => 'root',
'password' => 'password',
'persistent' => false,
'compress' => false,
),
'identifier' => '`',
'table_prefix' => '',
'charset' => 'utf8',
'enable_cache' => true,
'profiling' => false,
'readonly' => false,
),
'production' => array (
'type' => 'mysqli',
'connection' => array (
'hostname' => 'localhost',
'port' => '3306',
'database' => 'tutorialspoint_bookdb',
'username' => 'root',
'password' => 'password',
'persistent' => false,
'compress' => false,
),
'identifier' => '`',
'table_prefix' => '',
'charset' => 'utf8',
'enable_cache' => true,
'profiling' => false,
'readonly' => false,
),
);
8 단계 : Orm 패키지 포함
ORM 패키지를 포함하도록 기본 구성 파일을 업데이트하십시오. "fuel / app / config /"에 있습니다.
fuel / app / config / config.php
'always_load' => array (
'packages' => array (
'orm'
),
),
9 단계 : 모델 생성
“fuel / app / classes / model”에있는 book.php에서 책 모델을 만듭니다. 다음과 같이 정의됩니다-
fuel / app / classes / model / book.php
<?php
class Model_Book extends Orm\Model {
protected static $_connection = 'production';
protected static $_table_name = 'book';
protected static $_primary_key = array('id');
protected static $_properties = array (
'id',
'title' => array (
'data_type' => 'varchar',
'label' => 'Book title',
'validation' => array (
'required',
'min_length' => array(3),
'max_length' => array(80)
),
'form' => array (
'type' => 'text'
),
),
'author' => array (
'data_type' => 'varchar',
'label' => 'Book author',
'validation' => array (
'required',
),
'form' => array (
'type' => 'text'
),
),
'price' => array (
'data_type' => 'decimal',
'label' => 'Book price',
'validation' => array (
'required',
),
'form' => array (
'type' => 'text'
),
),
);
protected static $_observers = array('Orm\\Observer_Validation' => array (
'events' => array('before_save')
));
}
여기에서는 모델의 속성으로 데이터베이스 세부 정보를 지정했습니다. 유효성 검사 세부 정보도 있습니다.
10 단계 : 책 표시
책 컨트롤러에서 색인 조치를 업데이트하여 데이터베이스에서 사용 가능한 책을 나열하십시오.
fuel / app / classes / controller / book.php
<?php
class Controller_Book extends Controller_Template {
public $template = 'layout';
public function action_index() {
// Create the view object
$view = View::forge('book/index');
// fetch the book from database and set it to the view
$books = Model_Book::find('all');
$view->set('books', $books);
// set the template variables
$this->template->title = "Book index page";
$this->template->content = $view;
}
}
여기에서 우리는 orm 데이터베이스에서 도서 세부 정보를 가져온 다음 도서 세부 정보를 뷰에 전달했습니다.
11 단계 : 인덱스보기 업데이트
“fuel / app / views / book”에있는 view 파일 index.php를 업데이트합니다. 전체 업데이트 코드는 다음과 같습니다.
fuel / app / views / book / index.php
<table class = "table">
<thead>
<tr>
<th>#</th>
<th>Title</th>
<th>Author</th>
<th>Price</th>
<th></th>
</tr>
</thead>
<tbody>
<?php
foreach($books as $book) {
?>
<tr>
<td><?php echo $book['id']; ?></td>
<td><?php echo $book['title']; ?></td>
<td><?php echo $book['author']; ?></td>
<td><?php echo $book['price']; ?></td>
<td>
<a href = "/book/edit/<?php echo $book['id']; ?>">Edit</a>
<a href = "/book/delete/<?php echo $book['id']; ?>">Delete</a>
</td>
</tr>
<?php
}
?>
</tbody>
</table>
<ul>
</ul>
이제 URL을 요청하면 http : // localhost : 8080 /는 다음과 같이 페이지를 표시합니다.

12 단계 : 책을 추가하는 작업 만들기
서점에 새 책을 추가하는 기능을 만듭니다. 다음과 같이 북 컨트롤러에 새 액션 action_add를 생성합니다.
public function action_add() {
// create a new fieldset and add book model
$fieldset = Fieldset::forge('book')->add_model('Model_Book');
// get form from fieldset
$form = $fieldset->form();
// add submit button to the form
$form->add('Submit', '', array('type' => 'submit', 'value' => 'Submit'));
// build the form and set the current page as action
$formHtml = $fieldset->build(Uri::create('book/add'));
$view = View::forge('book/add');
$view->set('form', $formHtml, false);
if (Input::param() != array()) {
try {
$book = Model_Book::forge();
$book->title = Input::param('title');
$book->author = Input::param('author');
$book->price = Input::param('price');
$book->save();
Response::redirect('book');
} catch (Orm\ValidationFailed $e) {
$view->set('errors', $e->getMessage(), false);
}
}
$this->template->title = "Book add page";
$this->template->content = $view; }
여기에서는 다음 두 가지 프로세스가 수행됩니다.
Fieldset 메서드와 Book 모델을 사용하여 책을 추가하는 책 양식을 작성합니다.
사용자가 도서 정보를 입력하고 양식을 제출할 때 도서 양식을 처리합니다. 제출 된 데이터의 Input :: param () 메서드를 확인하여 찾을 수 있습니다. 양식 처리에는 다음 단계가 포함됩니다.
책 정보를 수집하십시오.
도서 정보를 확인합니다. 저장 방법 전에 호출 할 유효성 검사를 이미 설정했습니다. 유효성 검사가 실패하면 Orm \ ValidationFailed 예외가 발생합니다.
도서 정보를 데이터베이스에 저장합니다.
성공하면 사용자를 색인 페이지로 리디렉션합니다. 그렇지 않으면 양식을 다시 표시하십시오.
양식을 표시하고 동일한 작업으로 양식을 처리하면서 두 가지를 모두 수행합니다. 사용자가 처음으로 작업을 호출하면 양식이 표시됩니다. 사용자가 도서 정보를 입력하고 데이터를 제출하면 양식이 처리됩니다.
13 단계 : 책 추가 조치를위한보기 작성
책 추가 조치에 대한보기를 작성하십시오. 새 파일 fuel / app / views / book / add.php를 만들고 다음 코드를 입력합니다.
<style>
#form table {
width: 90%;
}
#form table tr {
width: 90%
}
#form table tr td {
width: 50%
}
#form input[type = text], select {
width: 100%;
padding: 12px 20px;
margin: 8px 0;
display: inline-block;
border: 1px solid #ccc;
border-radius: 4px;
box-sizing: border-box;
}
#form input[type = submit] {
width: 100%;
background-color: #3c3c3c;
color: white;
padding: 14px 20px;
margin: 8px 0;
border: none;
border-radius: 4px;
cursor: pointer;
}
#form div {
border-radius: 5px;
background-color: #f2f2f2;
padding: 20px;
}
</style>
<div id = "form">
<h2>Book form</h2>
<?php
if(isset($errors)) {
echo $errors;
}
echo $form;
?>
</div>
여기서는 action 메소드에서 생성 된 폼만 보여주고 있습니다. 또한 오류가있는 경우 표시합니다.
14 단계 : 책 추가 조치 확인
URL http : // localhost : 8080 / book / add를 요청하거나 책 탐색 추가 링크를 클릭하면 다음과 같은 양식이 표시됩니다.
형태

데이터가있는 양식

도서 정보를 입력하고 페이지를 제출하면 도서 정보가 데이터베이스에 저장되고 다음과 같이 페이지가 색인 페이지로 리디렉션됩니다.
새로 추가 된 도서가있는 도서 목록
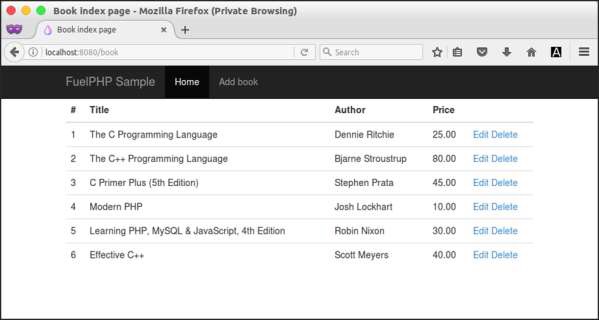
15 단계 : 책을 편집하기위한 조치 작성
기존 도서 정보를 편집하고 업데이트하는 기능을 만듭니다. 다음과 같이 책 컨트롤러에서 새 액션 action_edit를 만듭니다.
public function action_edit($id = false) {
if(!($book = Model_Book::find($id))) {
throw new HttpNotFoundException();
}
// create a new fieldset and add book model
$fieldset = Fieldset::forge('book')->add_model('Model_Book');
$fieldset->populate($book);
// get form from fieldset
$form = $fieldset->form();
// add submit button to the form
$form->add('Submit', '', array('type' => 'submit', 'value' => 'Submit'));
// build the form and set the current page as action
$formHtml = $fieldset->build(Uri::create('book/edit/' . $id));
$view = View::forge('book/add');
$view->set('form', $formHtml, false);
if (Input::param() != array()) {
try {
$book->title = Input::param('title');
$book->author = Input::param('author');
$book->price = Input::param('price');
$book->save();
Response::redirect('book');
} catch (Orm\ValidationFailed $e) {
$view->set('errors', $e->getMessage(), false);
}
}
$this->template->title = "Book edit page";
$this->template->content = $view;
}
페이지를 처리하기 전에 요청 된 책을 ID로 검색한다는 점을 제외하면 추가 조치와 유사합니다. 데이터베이스에서 도서 정보가 발견되면 계속 진행하여 양식에 도서 정보를 표시합니다. 그렇지 않으면 파일을 찾을 수 없음 예외가 발생하고 종료됩니다.
16 단계 : 편집 작업에 대한보기 만들기
책 편집 조치에 대한보기를 작성하십시오. 여기에서는 추가 작업에 사용 된 것과 동일한보기를 사용합니다.
17 단계 : 책 편집 작업 확인.
도서 목록 페이지에서 책의 편집 링크를 클릭하면 다음과 같은 해당 도서 양식이 표시됩니다.
도서 세부 정보가있는 양식

18 단계 : 책 삭제 조치 작성
서점에서 책을 삭제하는 기능을 만듭니다. 다음과 같이 북 컨트롤러에서 새 액션 action_delete를 생성합니다.
public function action_delete($id = null) {
if ( ! ($book = Model_Book::find($id))) {
throw new HttpNotFoundException();
} else {
$book->delete();
}
Response::redirect('book');
}
여기에서는 제공된 도서 ID를 사용하여 데이터베이스에 도서가 있는지 확인합니다. 책이 발견되면 삭제되고 색인 페이지로 리디렉션됩니다. 그렇지 않으면 페이지를 찾을 수없는 정보가 표시됩니다.
19 단계 : 삭제 작업 확인
도서 목록 페이지에서 삭제 링크를 클릭하여 삭제 작업을 확인하십시오. 요청 된 책을 삭제 한 다음 다시 색인 페이지로 리디렉션됩니다.
마지막으로 책 정보를 추가, 편집, 삭제 및 나열하는 모든 기능이 생성됩니다.
FuelPHP는 다른 MVC 기반 PHP 프레임 워크에 비해 간단하고 유연하며 확장 가능하며 쉽게 구성 할 수 있습니다. 최신 MVC 프레임 워크의 모든 기능을 제공합니다. 그대로 사용하거나 필요에 맞게 완전히 변경할 수 있습니다. 무엇보다도 웹 개발을위한 훌륭한 선택입니다.