Java RMI - aplikacja bazodanowa
W poprzednim rozdziale stworzyliśmy przykładową aplikację RMI, w której klient wywołuje metodę wyświetlającą okno GUI (JavaFX).
W tym rozdziale weźmiemy przykład, aby zobaczyć, jak program kliencki może pobrać rekordy tabeli w bazie danych MySQL znajdującej się na serwerze.
Załóżmy, że mamy tabelę o nazwie student_data w bazie danych details jak pokazano niżej.
+----+--------+--------+------------+---------------------+
| ID | NAME | BRANCH | PERCENTAGE | EMAIL |
+----+--------+--------+------------+---------------------+
| 1 | Ram | IT | 85 | [email protected] |
| 2 | Rahim | EEE | 95 | [email protected] |
| 3 | Robert | ECE | 90 | [email protected] |
+----+--------+--------+------------+---------------------+
Załóżmy, że nazwa użytkownika to myuser a jego hasło to password.
Tworzenie klasy studenckiej
Stwórz Student klasa z setter i getter metody, jak pokazano poniżej.
public class Student implements java.io.Serializable {
private int id, percent;
private String name, branch, email;
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getBranch() {
return branch;
}
public int getPercent() {
return percent;
}
public String getEmail() {
return email;
}
public void setID(int id) {
this.id = id;
}
public void setName(String name) {
this.name = name;
}
public void setBranch(String branch) {
this.branch = branch;
}
public void setPercent(int percent) {
this.percent = percent;
}
public void setEmail(String email) {
this.email = email;
}
}
Definiowanie interfejsu zdalnego
Zdefiniuj interfejs zdalny. Tutaj definiujemy zdalny interfejs o nazwieHello z metodą o nazwie getStudents ()w tym. Ta metoda zwraca listę zawierającą obiekt klasyStudent.
import java.rmi.Remote;
import java.rmi.RemoteException;
import java.util.*;
// Creating Remote interface for our application
public interface Hello extends Remote {
public List<Student> getStudents() throws Exception;
}
Opracowanie klasy implementacyjnej
Utwórz klasę i zaimplementuj powyższy utworzony interface.
Tutaj wdrażamy getStudents() metoda Remote interface. Po wywołaniu tej metody pobiera rekordy tabeli o nazwiestudent_data. Ustawia te wartości na klasę Student przy użyciu jej metod ustawiających, dodaje je do obiektu listy i zwraca tę listę.
import java.sql.*;
import java.util.*;
// Implementing the remote interface
public class ImplExample implements Hello {
// Implementing the interface method
public List<Student> getStudents() throws Exception {
List<Student> list = new ArrayList<Student>();
// JDBC driver name and database URL
String JDBC_DRIVER = "com.mysql.jdbc.Driver";
String DB_URL = "jdbc:mysql://localhost:3306/details";
// Database credentials
String USER = "myuser";
String PASS = "password";
Connection conn = null;
Statement stmt = null;
//Register JDBC driver
Class.forName("com.mysql.jdbc.Driver");
//Open a connection
System.out.println("Connecting to a selected database...");
conn = DriverManager.getConnection(DB_URL, USER, PASS);
System.out.println("Connected database successfully...");
//Execute a query
System.out.println("Creating statement...");
stmt = conn.createStatement();
String sql = "SELECT * FROM student_data";
ResultSet rs = stmt.executeQuery(sql);
//Extract data from result set
while(rs.next()) {
// Retrieve by column name
int id = rs.getInt("id");
String name = rs.getString("name");
String branch = rs.getString("branch");
int percent = rs.getInt("percentage");
String email = rs.getString("email");
// Setting the values
Student student = new Student();
student.setID(id);
student.setName(name);
student.setBranch(branch);
student.setPercent(percent);
student.setEmail(email);
list.add(student);
}
rs.close();
return list;
}
}
Program serwera
Program serwera RMI powinien implementować zdalny interfejs lub rozszerzać klasę implementacji. Tutaj powinniśmy utworzyć zdalny obiekt i powiązać go z plikiemRMI registry.
Poniżej znajduje się program serwera tej aplikacji. Tutaj rozszerzymy wyżej utworzoną klasę, utworzymy zdalny obiekt i zarejestrujemy go w rejestrze RMI pod nazwą wiązaniahello.
import java.rmi.registry.Registry;
import java.rmi.registry.LocateRegistry;
import java.rmi.RemoteException;
import java.rmi.server.UnicastRemoteObject;
public class Server extends ImplExample {
public Server() {}
public static void main(String args[]) {
try {
// Instantiating the implementation class
ImplExample obj = new ImplExample();
// Exporting the object of implementation class (
here we are exporting the remote object to the stub)
Hello stub = (Hello) UnicastRemoteObject.exportObject(obj, 0);
// Binding the remote object (stub) in the registry
Registry registry = LocateRegistry.getRegistry();
registry.bind("Hello", stub);
System.err.println("Server ready");
} catch (Exception e) {
System.err.println("Server exception: " + e.toString());
e.printStackTrace();
}
}
}
Program klienta
Poniżej znajduje się program kliencki tej aplikacji. Tutaj pobieramy zdalny obiekt i wywołujemy nazwaną metodęgetStudents(). Pobiera rekordy tabeli z obiektu listy i wyświetla je.
import java.rmi.registry.LocateRegistry;
import java.rmi.registry.Registry;
import java.util.*;
public class Client {
private Client() {}
public static void main(String[] args)throws Exception {
try {
// Getting the registry
Registry registry = LocateRegistry.getRegistry(null);
// Looking up the registry for the remote object
Hello stub = (Hello) registry.lookup("Hello");
// Calling the remote method using the obtained object
List<Student> list = (List)stub.getStudents();
for (Student s:list)v {
// System.out.println("bc "+s.getBranch());
System.out.println("ID: " + s.getId());
System.out.println("name: " + s.getName());
System.out.println("branch: " + s.getBranch());
System.out.println("percent: " + s.getPercent());
System.out.println("email: " + s.getEmail());
}
// System.out.println(list);
} catch (Exception e) {
System.err.println("Client exception: " + e.toString());
e.printStackTrace();
}
}
}
Kroki do uruchomienia przykładu
Poniżej przedstawiono kroki, aby uruchomić nasz przykład RMI.
Step 1 - Otwórz folder, w którym zapisałeś wszystkie programy i skompiluj wszystkie pliki Java, jak pokazano poniżej.
Javac *.java
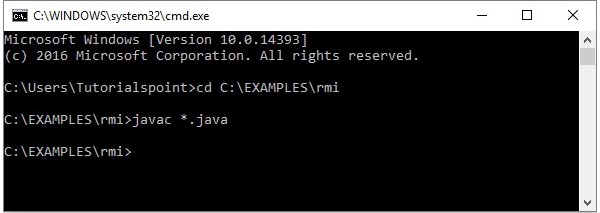
Step 2 - Uruchom rmi rejestru za pomocą następującego polecenia.
start rmiregistry

To rozpocznie rmi rejestru w osobnym oknie, jak pokazano poniżej.
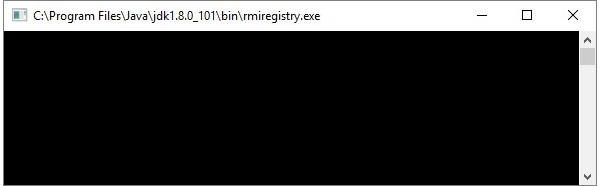
Step 3 - Uruchom plik klasy serwera, jak pokazano poniżej.
Java Server
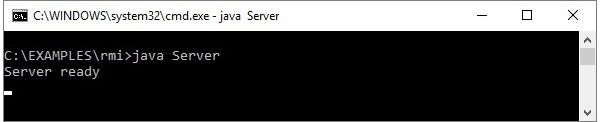
Step 4 - Uruchom plik klasy klienta, jak pokazano poniżej.
java Client
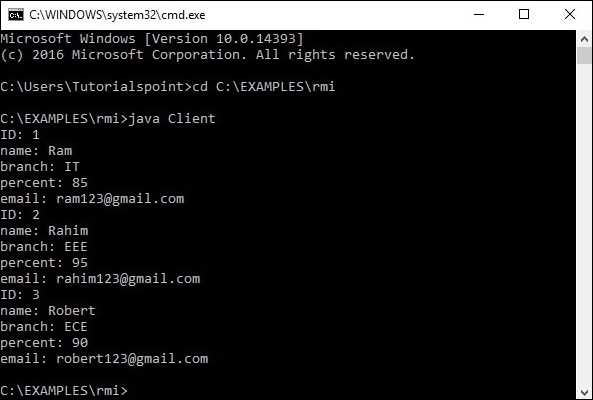