WCF - tworzenie usługi WCF
Tworzenie usługi WCF jest prostym zadaniem przy użyciu programu Microsoft Visual Studio 2012. Poniżej podano metodę krok po kroku tworzenia usługi WCF wraz z całym wymaganym kodowaniem, aby lepiej zrozumieć koncepcję.
- Uruchom program Visual Studio 2012.
- Kliknij nowy projekt, a następnie na karcie Visual C # wybierz opcję WCF.

Tworzona jest usługa WCF, która wykonuje podstawowe operacje arytmetyczne, takie jak dodawanie, odejmowanie, mnożenie i dzielenie. Główny kod znajduje się w dwóch różnych plikach - jednym interfejsie i jednej klasie.
WCF zawiera co najmniej jeden interfejs i jego zaimplementowane klasy.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.Text;
namespace WcfServiceLibrary1 {
// NOTE: You can use the "Rename" command on the "Refactor" menu to
// change the interface name "IService1" in both code and config file
// together.
[ServiceContract]
Public interface IService1 {
[OperationContract]
int sum(int num1, int num2);
[OperationContract]
int Subtract(int num1, int num2);
[OperationContract]
int Multiply(int num1, int num2);
[OperationContract]
int Divide(int num1, int num2);
}
// Use a data contract as illustrated in the sample below to add
// composite types to service operations.
[DataContract]
Public class CompositeType {
Bool boolValue = true;
String stringValue = "Hello ";
[DataMember]
Public bool BoolValue {
get { return boolValue; }
set { boolValue = value; }
}
[DataMember]
Public string StringValue {
get { return stringValue; }
set { stringValue = value; }
}
}
}
Kod odpowiadający jego klasie jest podany poniżej.
using System;
usingSystem.Collections.Generic;
usingSystem.Linq;
usingSystem.Runtime.Serialization;
usingSystem.ServiceModel;
usingSystem.Text;
namespace WcfServiceLibrary1 {
// NOTE: You can use the "Rename" command on the "Refactor" menu to
// change the class name "Service1" in both code and config file
// together.
publicclassService1 :IService1 {
// This Function Returns summation of two integer numbers
publicint sum(int num1, int num2) {
return num1 + num2;
}
// This function returns subtraction of two numbers.
// If num1 is smaller than number two then this function returns 0
publicint Subtract(int num1, int num2) {
if (num1 > num2) {
return num1 - num2;
}
else {
return 0;
}
}
// This function returns multiplication of two integer numbers.
publicint Multiply(int num1, int num2) {
return num1 * num2;
}
// This function returns integer value of two integer number.
// If num2 is 0 then this function returns 1.
publicint Divide(int num1, int num2) {
if (num2 != 0) {
return (num1 / num2);
} else {
return 1;
}
}
}
}
Aby uruchomić tę usługę, kliknij przycisk Start w programie Visual Studio.
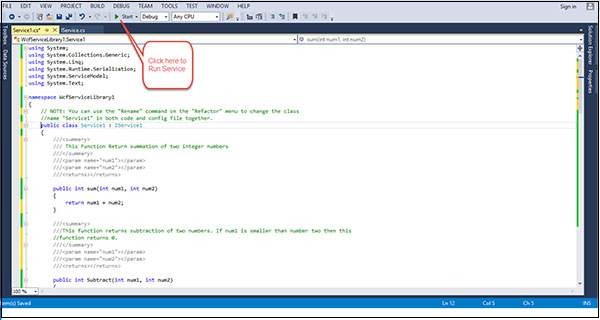
Gdy uruchamiamy tę usługę, pojawia się następujący ekran.

Po kliknięciu metody sumowania otwiera się następująca strona. Tutaj możesz wprowadzić dowolne dwie liczby całkowite i kliknąć przycisk Invoke. Usługa zwróci sumę tych dwóch liczb.

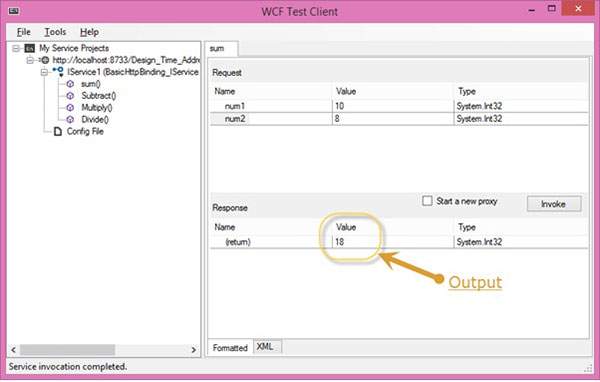
Podobnie jak sumowanie, możemy wykonać wszystkie inne operacje arytmetyczne, które są wymienione w menu. A oto dla nich zatrzaski.
Po kliknięciu metody Subtract pojawi się następująca strona. Wprowadź liczby całkowite, kliknij przycisk Wywołaj i uzyskaj dane wyjściowe, jak pokazano tutaj -

Po kliknięciu metody Multiply pojawi się następująca strona. Wprowadź liczby całkowite, kliknij przycisk Wywołaj i uzyskaj dane wyjściowe, jak pokazano tutaj -
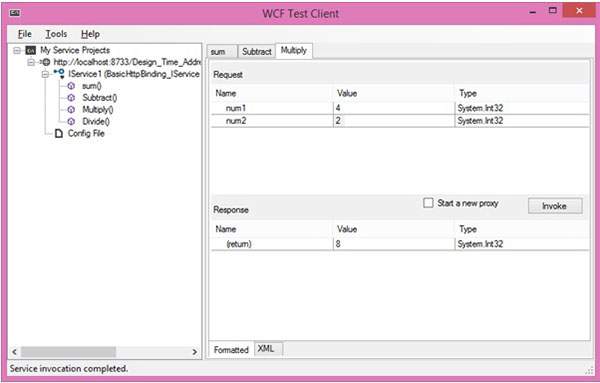
Po kliknięciu metody Divide pojawi się następująca strona. Wprowadź liczby całkowite, kliknij przycisk Wywołaj i uzyskaj dane wyjściowe, jak pokazano tutaj -

Po wywołaniu usługi możesz przełączać się między nimi bezpośrednio z tego miejsca.
