Highcharts GWT - Sintaxe de configuração
Neste capítulo, mostraremos a configuração necessária para desenhar um gráfico usando a API Highcharts no GWT.
Etapa 1: Criar aplicativo GWT
Siga as etapas a seguir para atualizar o aplicativo GWT que criamos no GWT - capítulo Criar aplicativo -
Degrau | Descrição |
---|---|
1 | Crie um projeto com o nome HelloWorld em um pacote com.tutorialspoint conforme explicado no capítulo GWT - Criar aplicativo . |
2 | Modifique HelloWorld.gwt.xml , HelloWorld.html e HelloWorld.java conforme explicado abaixo. Mantenha o resto dos arquivos inalterados. |
3 | Compile e execute o aplicativo para verificar o resultado da lógica implementada. |
A seguir está o conteúdo do descritor do módulo modificado src/com.tutorialspoint/HelloWorld.gwt.xml.
<?xml version = "1.0" encoding = "UTF-8"?>
<module rename-to = 'helloworld'>
<inherits name = 'com.google.gwt.user.User'/>
<inherits name = 'com.google.gwt.user.theme.clean.Clean'/>
<entry-point class = 'com.tutorialspoint.client.HelloWorld'/>
<inherits name="org.moxieapps.gwt.highcharts.Highcharts"/>
<source path = 'client'/>
<source path = 'shared'/>
</module>
A seguir está o conteúdo do arquivo host HTML modificado war/HelloWorld.html.
<html>
<head>
<title>GWT Highcharts Showcase</title>
<link rel = "stylesheet" href = "HelloWorld.css"/>
<script language = "javascript" src = "helloworld/helloworld.nocache.js">
<script src = "https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js" />
<script src = "https://code.highcharts.com/highcharts.js" />
</script>
</head>
<body>
</body>
</html>
Veremos o HelloWorld.java atualizado no final, após entender as configurações.
Etapa 2: Criar configurações
Criar gráfico
Configure o tipo, título e subtítulo do gráfico.
Chart chart = new Chart()
.setType(Type.SPLINE)
.setChartTitleText("Monthly Average Temperature")
.setChartSubtitleText("Source: WorldClimate.com");
xAxis
Configure o ticker a ser exibido no eixo X.
XAxis xAxis = chart.getXAxis();
xAxis.setCategories("Jan", "Feb", "Mar", "Apr", "May", "Jun",
"Jul", "Aug", "Sep", "Oct", "Nov", "Dec");
yAxis
Configure o título, plote as linhas a serem exibidas no eixo Y.
YAxis yAxis = chart.getYAxis();
yAxis.setAxisTitleText("Temperature °C");
yAxis.createPlotLine()
.setValue(0)
.setWidth(1)
.setColor("#808080");
dica
Configure a dica de ferramenta. Coloque o sufixo a ser adicionado após o valor (eixo y).
ToolTip toolTip = new ToolTip();
toolTip.setValueSuffix("°C");
chart.setToolTip(toolTip);
lenda
Configure a legenda a ser exibida no lado direito do gráfico junto com outras propriedades.
legend.setLayout(Legend.Layout.VERTICAL)
.setAlign(Legend.Align.RIGHT)
.setVerticalAlign(Legend.VerticalAlign.TOP)
.setX(-10)
.setY(100)
.setBorderWidth(0);
chart.setLegend(legend);
Series
Configure os dados a serem exibidos no gráfico. Série é uma matriz em que cada elemento dessa matriz representa uma única linha no gráfico.
chart.addSeries(chart.createSeries()
.setName("Tokyo")
.setPoints(new Number[] {
7.0, 6.9, 9.5, 14.5, 18.2, 21.5, 25.2,
26.5, 23.3, 18.3, 13.9, 9.6
})
);
chart.addSeries(chart.createSeries()
.setName("New York")
.setPoints(new Number[] {
-0.2, 0.8, 5.7, 11.3, 17.0, 22.0, 24.8,
24.1, 20.1, 14.1, 8.6, 2.5
})
);
chart.addSeries(chart.createSeries()
.setName("Berlin")
.setPoints(new Number[] {
-0.9, 0.6, 3.5, 8.4, 13.5, 17.0, 18.6,
17.9, 14.3, 9.0, 3.9, 1.0
})
);
chart.addSeries(chart.createSeries()
.setName("London")
.setPoints(new Number[] {
3.9, 4.2, 5.7, 8.5, 11.9, 15.2, 17.0,
16.6, 14.2, 10.3, 6.6, 4.8
})
);
Etapa 3: adicione o gráfico ao painel principal.
Estamos adicionando o gráfico ao painel raiz.
RootPanel.get().add(chart);
Exemplo
Considere o seguinte exemplo para entender melhor a sintaxe de configuração -
HelloWorld.java
package com.tutorialspoint.client;
import org.moxieapps.gwt.highcharts.client.Chart;
import org.moxieapps.gwt.highcharts.client.Legend;
import org.moxieapps.gwt.highcharts.client.Series.Type;
import org.moxieapps.gwt.highcharts.client.ToolTip;
import org.moxieapps.gwt.highcharts.client.XAxis;
import org.moxieapps.gwt.highcharts.client.YAxis;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.user.client.ui.RootPanel;
public class HelloWorld implements EntryPoint {
public void onModuleLoad() {
Chart chart = new Chart()
.setType(Type.SPLINE)
.setChartTitleText("Monthly Average Temperature")
.setChartSubtitleText("Source: WorldClimate.com");
XAxis xAxis = chart.getXAxis();
xAxis.setCategories("Jan", "Feb", "Mar", "Apr", "May", "Jun",
"Jul", "Aug", "Sep", "Oct", "Nov", "Dec");
YAxis yAxis = chart.getYAxis();
yAxis.setAxisTitleText("Temperature °C");
yAxis.createPlotLine()
.setValue(0)
.setWidth(1)
.setColor("#808080");
ToolTip toolTip = new ToolTip();
toolTip.setValueSuffix("°C");
chart.setToolTip(toolTip);
Legend legend = new Legend();
legend.setLayout(Legend.Layout.VERTICAL)
.setAlign(Legend.Align.RIGHT)
.setVerticalAlign(Legend.VerticalAlign.TOP)
.setX(-10)
.setY(100)
.setBorderWidth(0);
chart.setLegend(legend);
chart.addSeries(chart.createSeries()
.setName("Tokyo")
.setPoints(new Number[] {
7.0, 6.9, 9.5, 14.5, 18.2, 21.5, 25.2,
26.5, 23.3, 18.3, 13.9, 9.6
})
);
chart.addSeries(chart.createSeries()
.setName("New York")
.setPoints(new Number[] {
-0.2, 0.8, 5.7, 11.3, 17.0, 22.0, 24.8,
24.1, 20.1, 14.1, 8.6, 2.5
})
);
chart.addSeries(chart.createSeries()
.setName("Berlin")
.setPoints(new Number[] {
-0.9, 0.6, 3.5, 8.4, 13.5, 17.0, 18.6,
17.9, 14.3, 9.0, 3.9, 1.0
})
);
chart.addSeries(chart.createSeries()
.setName("London")
.setPoints(new Number[] {
3.9, 4.2, 5.7, 8.5, 11.9, 15.2, 17.0,
16.6, 14.2, 10.3, 6.6, 4.8
})
);
RootPanel.get().add(chart);
}
}
Resultado
Verifique o resultado.
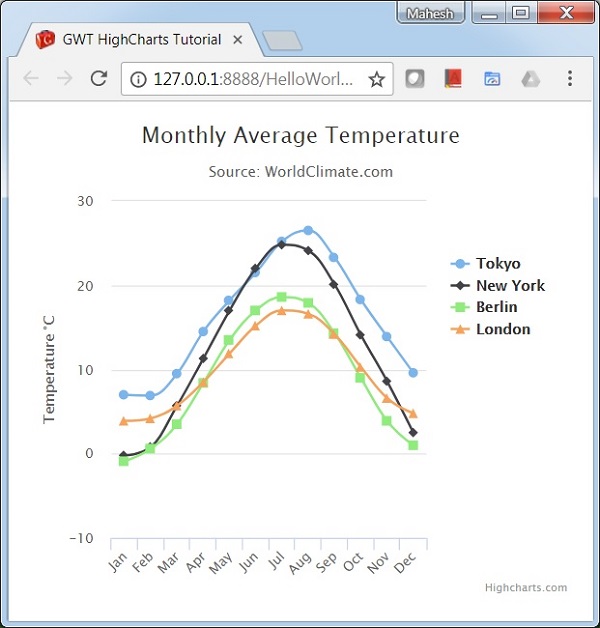