Spring Boot - Enviando e-mail
Usando o serviço da web Spring Boot RESTful, você pode enviar um e-mail com o Gmail Transport Layer Security. Neste capítulo, vamos entender em detalhes como usar esse recurso.
Primeiro, precisamos adicionar a dependência Spring Boot Starter Mail em seu arquivo de configuração de compilação.
Os usuários do Maven podem adicionar a seguinte dependência ao arquivo pom.xml.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
Os usuários do Gradle podem adicionar a seguinte dependência ao seu arquivo build.gradle.
compile('org.springframework.boot:spring-boot-starter-mail')
O código do arquivo de classe do aplicativo Spring Boot principal é fornecido abaixo -
package com.tutorialspoint.emailapp;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class EmailappApplication {
public static void main(String[] args) {
SpringApplication.run(EmailappApplication.class, args);
}
}
Você pode escrever uma API Rest simples para enviar por e-mail no arquivo de classe Rest Controller, conforme mostrado.
package com.tutorialspoint.emailapp;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class EmailController {
@RequestMapping(value = "/sendemail")
public String sendEmail() {
return "Email sent successfully";
}
}
Você pode escrever um método para enviar o e-mail com anexo. Defina as propriedades mail.smtp e use PasswordAuthentication.
private void sendmail() throws AddressException, MessagingException, IOException {
Properties props = new Properties();
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", "smtp.gmail.com");
props.put("mail.smtp.port", "587");
Session session = Session.getInstance(props, new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("[email protected]", "<your password>");
}
});
Message msg = new MimeMessage(session);
msg.setFrom(new InternetAddress("[email protected]", false));
msg.setRecipients(Message.RecipientType.TO, InternetAddress.parse("[email protected]"));
msg.setSubject("Tutorials point email");
msg.setContent("Tutorials point email", "text/html");
msg.setSentDate(new Date());
MimeBodyPart messageBodyPart = new MimeBodyPart();
messageBodyPart.setContent("Tutorials point email", "text/html");
Multipart multipart = new MimeMultipart();
multipart.addBodyPart(messageBodyPart);
MimeBodyPart attachPart = new MimeBodyPart();
attachPart.attachFile("/var/tmp/image19.png");
multipart.addBodyPart(attachPart);
msg.setContent(multipart);
Transport.send(msg);
}
Agora, chame o método sendmail () acima da API Rest como mostrado -
@RequestMapping(value = "/sendemail")
public String sendEmail() throws AddressException, MessagingException, IOException {
sendmail();
return "Email sent successfully";
}
Note - LIGUE para permitir aplicativos menos seguros nas configurações da sua conta do Gmail antes de enviar um e-mail.
O arquivo de configuração de compilação completo é fornecido abaixo.
Maven – pom.xml
<?xml version = "1.0" encoding = "UTF-8"?>
<project xmlns = "http://maven.apache.org/POM/4.0.0"
xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation = "http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.tutorialspoint</groupId>
<artifactId>emailapp</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>emailapp</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.9.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Gradle – build.gradle
buildscript {
ext {
springBootVersion = '1.5.9.RELEASE'
}
repositories {
mavenCentral()
}
dependencies {
classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}")
}
}
apply plugin: 'java'
apply plugin: 'eclipse'
apply plugin: 'org.springframework.boot'
group = 'com.tutorialspoint'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
dependencies {
compile('org.springframework.boot:spring-boot-starter-web')
compile('org.springframework.boot:spring-boot-starter-mail')
testCompile('org.springframework.boot:spring-boot-starter-test')
}
Agora, você pode criar um arquivo JAR executável e executar o aplicativo Spring Boot usando os comandos Maven ou Gradle mostrados abaixo -
Para Maven, você pode usar o comando conforme mostrado -
mvn clean install
Após “BUILD SUCCESS”, você pode encontrar o arquivo JAR no diretório de destino.
Para Gradle, você pode usar o comando conforme mostrado -
gradle clean build
Depois de “BUILD SUCCESSFUL”, você pode encontrar o arquivo JAR no diretório build / libs.
Agora, execute o arquivo JAR usando o comando fornecido abaixo -
java –jar <JARFILE>
Você pode ver que o aplicativo foi iniciado na porta 8080 do Tomcat.

Agora acesse a seguinte URL do seu navegador e você receberá um e-mail.
http://localhost:8080/sendemail
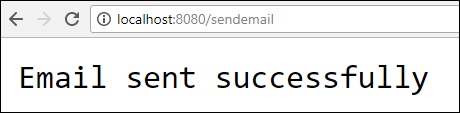
