DynamoDB - Cập nhật các mục
Cập nhật một mục trong DynamoDB chủ yếu bao gồm việc chỉ định khóa chính đầy đủ và tên bảng cho mục đó. Nó yêu cầu một giá trị mới cho mỗi thuộc tính bạn sửa đổi. Hoạt động sử dụngUpdateItem, sửa đổi các mục hiện có hoặc tạo chúng khi phát hiện ra một mục bị thiếu.
Trong các bản cập nhật, bạn có thể muốn theo dõi các thay đổi bằng cách hiển thị các giá trị ban đầu và mới, trước và sau các thao tác. UpdateItem sử dụngReturnValues để đạt được điều này.
Note - Hoạt động không báo cáo mức tiêu thụ đơn vị công suất, nhưng bạn có thể sử dụng ReturnConsumedCapacity tham số.
Sử dụng bảng điều khiển GUI, Java hoặc bất kỳ công cụ nào khác để thực hiện tác vụ này.
Làm thế nào để cập nhật các mục bằng công cụ GUI?
Điều hướng đến bảng điều khiển. Trong ngăn điều hướng ở bên trái, hãy chọnTables. Chọn bảng cần thiết, sau đó chọnItems chuyển hướng.
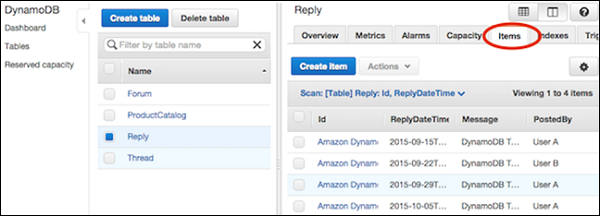
Chọn mục mong muốn để cập nhật và chọn Actions | Edit.
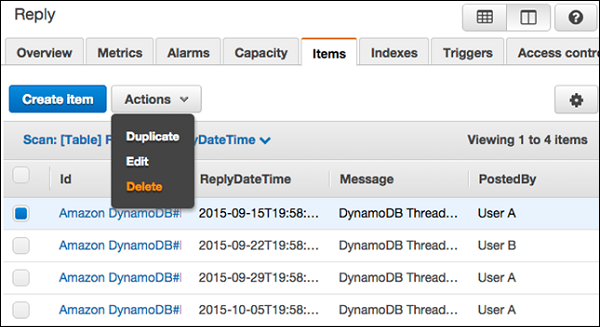
Sửa đổi bất kỳ thuộc tính hoặc giá trị nào cần thiết trong Edit Item cửa sổ.
Cập nhật các mục bằng Java
Sử dụng Java trong các hoạt động cập nhật mục yêu cầu tạo một cá thể lớp Bảng và gọi updateItemphương pháp. Sau đó, bạn chỉ định khóa chính của mặt hàng và cung cấpUpdateExpression chi tiết sửa đổi thuộc tính.
Sau đây là một ví dụ về điều tương tự -
DynamoDB dynamoDB = new DynamoDB(new AmazonDynamoDBClient(
new ProfileCredentialsProvider()));
Table table = dynamoDB.getTable("ProductList");
Map<String, String> expressionAttributeNames = new HashMap<String, String>();
expressionAttributeNames.put("#M", "Make");
expressionAttributeNames.put("#P", "Price
expressionAttributeNames.put("#N", "ID");
Map<String, Object> expressionAttributeValues = new HashMap<String, Object>();
expressionAttributeValues.put(":val1",
new HashSet<String>(Arrays.asList("Make1","Make2")));
expressionAttributeValues.put(":val2", 1); //Price
UpdateItemOutcome outcome = table.updateItem(
"internalID", // key attribute name
111, // key attribute value
"add #M :val1 set #P = #P - :val2 remove #N", // UpdateExpression
expressionAttributeNames,
expressionAttributeValues);
Các updateItem method cũng cho phép xác định các điều kiện, có thể thấy trong ví dụ sau:
Table table = dynamoDB.getTable("ProductList");
Map<String, String> expressionAttributeNames = new HashMap<String, String>();
expressionAttributeNames.put("#P", "Price");
Map<String, Object> expressionAttributeValues = new HashMap<String, Object>();
expressionAttributeValues.put(":val1", 44); // change Price to 44
expressionAttributeValues.put(":val2", 15); // only if currently 15
UpdateItemOutcome outcome = table.updateItem (new PrimaryKey("internalID",111),
"set #P = :val1", // Update
"#P = :val2", // Condition
expressionAttributeNames,
expressionAttributeValues);
Cập nhật mặt hàng bằng bộ đếm
DynamoDB cho phép bộ đếm nguyên tử, có nghĩa là sử dụng UpdateItem để tăng / giảm các giá trị thuộc tính mà không ảnh hưởng đến các yêu cầu khác; hơn nữa, các quầy luôn cập nhật.
Sau đây là một ví dụ giải thích cách nó có thể được thực hiện.
Note- Mẫu sau có thể giả sử là nguồn dữ liệu đã tạo trước đó. Trước khi cố gắng thực thi, hãy thu thập các thư viện hỗ trợ và tạo các nguồn dữ liệu cần thiết (các bảng có các đặc điểm bắt buộc hoặc các nguồn tham chiếu khác).
Mẫu này cũng sử dụng Eclipse IDE, tệp thông tin đăng nhập AWS và Bộ công cụ AWS trong Dự án Java AWS của Eclipse.
package com.amazonaws.codesamples.document;
import java.io.IOException;
import java.util.Arrays;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import com.amazonaws.auth.profile.ProfileCredentialsProvider;
import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClient;
import com.amazonaws.services.dynamodbv2.document.DeleteItemOutcome;
import com.amazonaws.services.dynamodbv2.document.DynamoDB;
import com.amazonaws.services.dynamodbv2.document.Item;
import com.amazonaws.services.dynamodbv2.document.Table;
import com.amazonaws.services.dynamodbv2.document.UpdateItemOutcome;
import com.amazonaws.services.dynamodbv2.document.spec.DeleteItemSpec;
import com.amazonaws.services.dynamodbv2.document.spec.UpdateItemSpec;
import com.amazonaws.services.dynamodbv2.document.utils.NameMap;
import com.amazonaws.services.dynamodbv2.document.utils.ValueMap;
import com.amazonaws.services.dynamodbv2.model.ReturnValue;
public class UpdateItemOpSample {
static DynamoDB dynamoDB = new DynamoDB(new AmazonDynamoDBClient(
new ProfileCredentialsProvider()));
static String tblName = "ProductList";
public static void main(String[] args) throws IOException {
createItems();
retrieveItem();
// Execute updates
updateMultipleAttributes();
updateAddNewAttribute();
updateExistingAttributeConditionally();
// Item deletion
deleteItem();
}
private static void createItems() {
Table table = dynamoDB.getTable(tblName);
try {
Item item = new Item()
.withPrimaryKey("ID", 303)
.withString("Nomenclature", "Polymer Blaster 4000")
.withStringSet( "Manufacturers",
new HashSet<String>(Arrays.asList("XYZ Inc.", "LMNOP Inc.")))
.withNumber("Price", 50000)
.withBoolean("InProduction", true)
.withString("Category", "Laser Cutter");
table.putItem(item);
item = new Item()
.withPrimaryKey("ID", 313)
.withString("Nomenclature", "Agitatatron 2000")
.withStringSet( "Manufacturers",
new HashSet<String>(Arrays.asList("XYZ Inc,", "CDE Inc.")))
.withNumber("Price", 40000)
.withBoolean("InProduction", true)
.withString("Category", "Agitator");
table.putItem(item);
} catch (Exception e) {
System.err.println("Cannot create items.");
System.err.println(e.getMessage());
}
}
private static void updateAddNewAttribute() {
Table table = dynamoDB.getTable(tableName);
try {
Map<String, String> expressionAttributeNames = new HashMap<String, String>();
expressionAttributeNames.put("#na", "NewAttribute");
UpdateItemSpec updateItemSpec = new UpdateItemSpec()
.withPrimaryKey("ID", 303)
.withUpdateExpression("set #na = :val1")
.withNameMap(new NameMap()
.with("#na", "NewAttribute"))
.withValueMap(new ValueMap()
.withString(":val1", "A value"))
.withReturnValues(ReturnValue.ALL_NEW);
UpdateItemOutcome outcome = table.updateItem(updateItemSpec);
// Confirm
System.out.println("Displaying updated item...");
System.out.println(outcome.getItem().toJSONPretty());
} catch (Exception e) {
System.err.println("Cannot add an attribute in " + tableName);
System.err.println(e.getMessage());
}
}
}