TypeORM - Thao tác truy vấn
Thao tác dữ liệu được sử dụng để quản lý và xem dữ liệu. Phần này giải thích về cách truy cập các truy vấn cơ sở dữ liệu như chèn, cập nhật, chọn và xóa các truy vấn bằng QueryBuilder. Chúng ta hãy đi qua từng chi tiết một.
Xây dựng truy vấn chèn
Hãy để chúng tôi tạo một thực thể Khách hàng như sau:
Khách hàng.ts
import {Entity, PrimaryGeneratedColumn, Column} from "typeorm";
@Entity()
export class Customer {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
@Column()
age: number;
}
Hãy thêm các thay đổi sau trong index.ts như sau:
index.ts
import "reflect-metadata";
import {createConnection} from "typeorm";
import {Customer} from "./entity/Customer";
import {getConnection} from "typeorm";
createConnection().then(async connection => {
await getConnection().createQueryBuilder() .insert()
.into(Customer)
.values([ { name: "Adam",age:11},
{ name: "David",age:12} ]) .execute();
}).catch(error => console.log(error));
Bây giờ, hãy khởi động ứng dụng của bạn bằng lệnh dưới đây -
npm start
Đầu ra
Bạn có thể thấy kết quả sau trên màn hình của mình:

Bây giờ mở máy chủ mysql của bạn, bảng được chèn với hai trường như hình dưới đây -
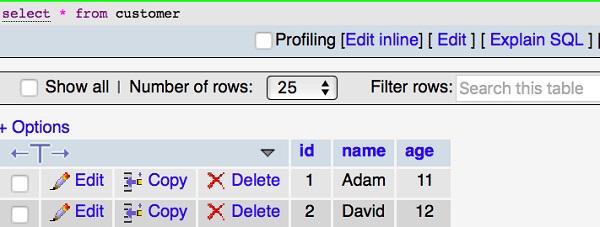
Xây dựng truy vấn cập nhật
Phần cuối cùng, chúng tôi đã chèn hai hàng dữ liệu. Hãy kiểm tra cách hoạt động của truy vấn cập nhật. Thêm các thay đổi sau trong index.ts như sau:
import "reflect-metadata";
import {createConnection} from "typeorm";
import {Customer} from "./entity/Customer";
import {getConnection} from "typeorm";
createConnection().then(async connection => {
await getConnection()
.createQueryBuilder() .update(Customer)
.set({ name: "Michael" }) .where("id = :id", { id: 1 }) .execute();
console.log("data updated");
}).catch(error => console.log(error));
Bây giờ, hãy khởi động ứng dụng của bạn bằng lệnh dưới đây -
npm start
Bạn có thể thấy kết quả sau trên màn hình của mình:

Bảng Mysql được sửa đổi như hình dưới đây -
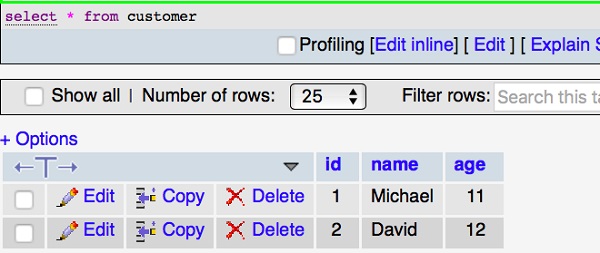
Tạo truy vấn chọn
selecttruy vấn được sử dụng để hiển thị các bản ghi từ bảng. Hãy thêm đoạn mã sau vàoindex.ts như sau -
index.ts
import "reflect-metadata";
import {createConnection} from "typeorm";
import {Customer} from "./entity/Customer";
createConnection().then(async connection => {
console.log("Display records from Customer table...");
const cus = new Customer();
console.log("Loading customers from the database...");
const customers = await connection.manager.find(Customer); console.log("Loaded users: ", customers);
}).catch(error => console.log(error));
Bạn có thể thấy kết quả sau trên màn hình của mình:

biểu hiện ở đâu
Hãy để chúng tôi thêm biểu thức where trong truy vấn để lọc khách hàng. Mã mẫu như sau:
import "reflect-metadata";
import {createConnection} from "typeorm";
import {Customer} from "./entity/Customer";
import {getConnection} from "typeorm";
createConnection().then(async connection => {
const customer = await getConnection() .createQueryBuilder() .select("cus")
.from(Customer, "cus") .where("cus.id = :id", { id: 1 }) .getOne();
console.log(customer);
})
.catch(error => console.log(error));
Chương trình trên sẽ trả về các bản ghi id đầu tiên. Bạn có thể thấy kết quả sau trên màn hình của mình,

Tương tự, bạn cũng có thể thử các biểu thức khác.
Tạo truy vấn xóa
Phần cuối, chúng tôi đã chèn, cập nhật và chọn lọc dữ liệu. Hãy kiểm tra cách hoạt động của truy vấn xóa. Thêm các thay đổi sau trong index.ts như sau:
import "reflect-metadata";
import {createConnection} from "typeorm";
import {Customer} from "./entity/Customer";
import {getConnection} from "typeorm";
createConnection().then(async connection => {
await getConnection() .createQueryBuilder()
.delete()
.from(Customer)
.where("id = :id", { id: 1 }) .execute();
console.log("data deleted"); }).catch(error => console.log(error));
Bạn có thể thấy kết quả sau trên màn hình của mình:

Và bảng mysql của bạn được sửa đổi như sau:
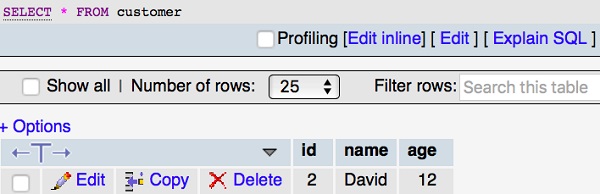