WCF - Criando Serviço WCF
Criar um serviço WCF é uma tarefa simples usando o Microsoft Visual Studio 2012. Abaixo está o método passo a passo para criar um serviço WCF junto com toda a codificação necessária, para entender o conceito de uma maneira melhor.
- Inicie o Visual Studio 2012.
- Clique no novo projeto e, em seguida, na guia Visual C #, selecione a opção WCF.

É criado um serviço WCF que executa operações aritméticas básicas como adição, subtração, multiplicação e divisão. O código principal está em dois arquivos diferentes - uma interface e uma classe.
Um WCF contém uma ou mais interfaces e suas classes implementadas.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.Text;
namespace WcfServiceLibrary1 {
// NOTE: You can use the "Rename" command on the "Refactor" menu to
// change the interface name "IService1" in both code and config file
// together.
[ServiceContract]
Public interface IService1 {
[OperationContract]
int sum(int num1, int num2);
[OperationContract]
int Subtract(int num1, int num2);
[OperationContract]
int Multiply(int num1, int num2);
[OperationContract]
int Divide(int num1, int num2);
}
// Use a data contract as illustrated in the sample below to add
// composite types to service operations.
[DataContract]
Public class CompositeType {
Bool boolValue = true;
String stringValue = "Hello ";
[DataMember]
Public bool BoolValue {
get { return boolValue; }
set { boolValue = value; }
}
[DataMember]
Public string StringValue {
get { return stringValue; }
set { stringValue = value; }
}
}
}
O código por trás de sua classe é fornecido abaixo.
using System;
usingSystem.Collections.Generic;
usingSystem.Linq;
usingSystem.Runtime.Serialization;
usingSystem.ServiceModel;
usingSystem.Text;
namespace WcfServiceLibrary1 {
// NOTE: You can use the "Rename" command on the "Refactor" menu to
// change the class name "Service1" in both code and config file
// together.
publicclassService1 :IService1 {
// This Function Returns summation of two integer numbers
publicint sum(int num1, int num2) {
return num1 + num2;
}
// This function returns subtraction of two numbers.
// If num1 is smaller than number two then this function returns 0
publicint Subtract(int num1, int num2) {
if (num1 > num2) {
return num1 - num2;
}
else {
return 0;
}
}
// This function returns multiplication of two integer numbers.
publicint Multiply(int num1, int num2) {
return num1 * num2;
}
// This function returns integer value of two integer number.
// If num2 is 0 then this function returns 1.
publicint Divide(int num1, int num2) {
if (num2 != 0) {
return (num1 / num2);
} else {
return 1;
}
}
}
}
Para executar este serviço, clique no botão Iniciar no Visual Studio.
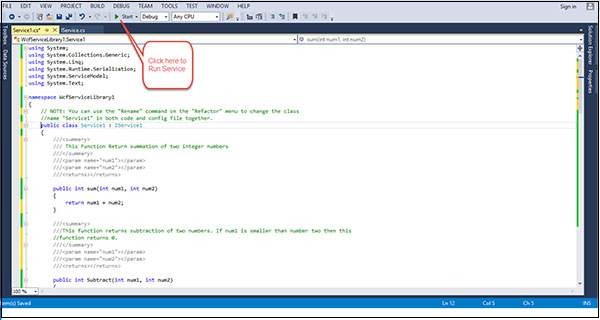
Enquanto executamos este serviço, a seguinte tela aparece.

Ao clicar no método de soma, a página seguinte é aberta. Aqui, você pode inserir quaisquer dois números inteiros e clicar no botão Chamar. O serviço retornará a soma desses dois números.

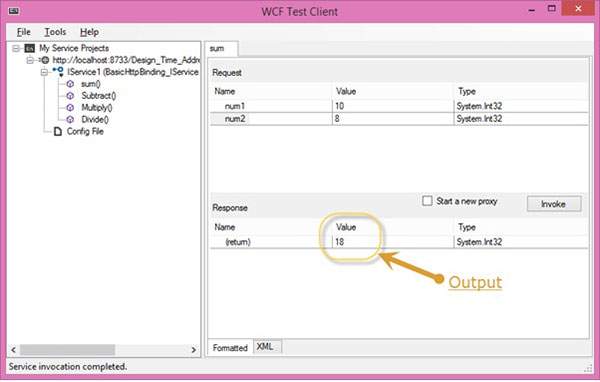
Como a soma, podemos realizar todas as outras operações aritméticas listadas no menu. E aqui estão as fotos para eles.
A página a seguir aparece ao clicar no método Subtrair. Insira os números inteiros, clique no botão Chamar e obtenha a saída conforme mostrado aqui -

A página a seguir aparece ao clicar no método Multiply. Insira os números inteiros, clique no botão Chamar e obtenha a saída conforme mostrado aqui -
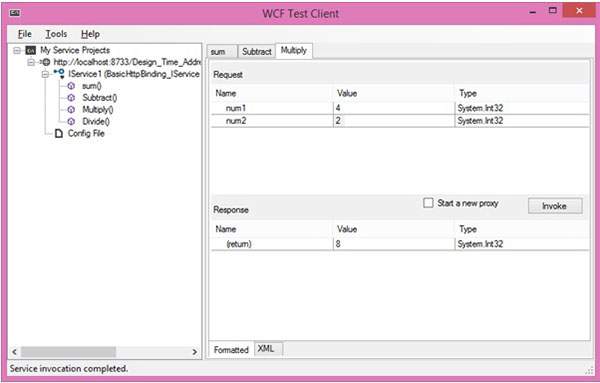
A página a seguir aparece ao clicar no método Divide. Insira os números inteiros, clique no botão Chamar e obtenha a saída conforme mostrado aqui -

Depois que o serviço é chamado, você pode alternar entre eles diretamente aqui.
