CherryPy - แอปพลิเคชันที่ใช้งานได้
แอปพลิเคชันสแต็กแบบเต็มช่วยอำนวยความสะดวกในการสร้างแอปพลิเคชั่นใหม่ผ่านคำสั่งหรือการเรียกใช้ไฟล์
พิจารณาแอปพลิเคชัน Python เช่น web2py framework โครงการ / แอปพลิเคชันทั้งหมดถูกสร้างขึ้นในแง่ของกรอบ MVC ในทำนองเดียวกัน CherryPy ช่วยให้ผู้ใช้สามารถตั้งค่าและกำหนดค่าโครงร่างของโค้ดได้ตามความต้องการ
ในบทนี้เราจะเรียนรู้รายละเอียดเกี่ยวกับการสร้างแอปพลิเคชั่น CherryPy และดำเนินการ
ระบบไฟล์
ระบบไฟล์ของแอปพลิเคชันจะแสดงในภาพหน้าจอต่อไปนี้ -
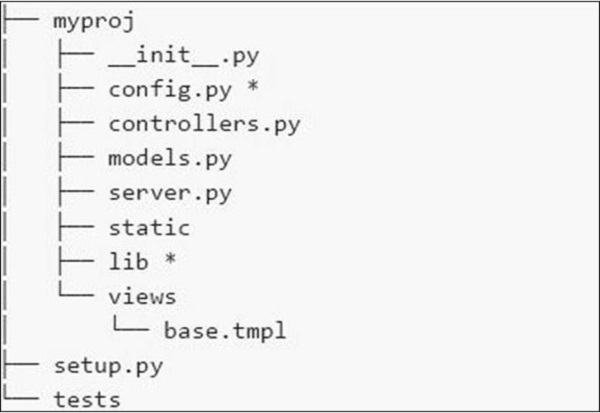
นี่คือคำอธิบายสั้น ๆ ของไฟล์ต่างๆที่เรามีในระบบไฟล์ -
config.py- ทุกแอปพลิเคชันต้องการไฟล์กำหนดค่าและวิธีโหลด ฟังก์ชันนี้สามารถกำหนดได้ใน config.py
controllers.py- MVC เป็นรูปแบบการออกแบบยอดนิยมตามมาด้วยผู้ใช้ controllers.py คือที่วัตถุทั้งหมดที่มีการดำเนินการที่จะถูกติดตั้งอยู่บนcherrypy.tree
models.py - ไฟล์นี้โต้ตอบกับฐานข้อมูลโดยตรงสำหรับบริการบางอย่างหรือสำหรับจัดเก็บข้อมูลถาวร
server.py - ไฟล์นี้โต้ตอบกับเว็บเซิร์ฟเวอร์ที่พร้อมใช้งานจริงซึ่งทำงานได้อย่างเหมาะสมกับพร็อกซีโหลดบาลานซ์
Static - รวมไฟล์ CSS และรูปภาพทั้งหมด
Views - รวมไฟล์เทมเพลตทั้งหมดสำหรับแอปพลิเคชันที่กำหนด
ตัวอย่าง
ให้เราเรียนรู้รายละเอียดขั้นตอนในการสร้างแอปพลิเคชัน CherryPy
Step 1 - สร้างแอปพลิเคชันที่ควรมีแอปพลิเคชัน
Step 2- ภายในไดเร็กทอรีให้สร้างแพ็คเกจ python ที่ตรงกับโปรเจ็กต์ สร้างไดเร็กทอรี gedit และรวมไฟล์ _init_.py ไว้ในไฟล์เดียวกัน
Step 3 - ภายในแพ็คเกจประกอบด้วยไฟล์ controllers.py พร้อมเนื้อหาดังต่อไปนี้ -
#!/usr/bin/env python
import cherrypy
class Root(object):
def __init__(self, data):
self.data = data
@cherrypy.expose
def index(self):
return 'Hi! Welcome to your application'
def main(filename):
data = {} # will be replaced with proper functionality later
# configuration file
cherrypy.config.update({
'tools.encode.on': True, 'tools.encode.encoding': 'utf-8',
'tools.decode.on': True,
'tools.trailing_slash.on': True,
'tools.staticdir.root': os.path.abspath(os.path.dirname(__file__)),
})
cherrypy.quickstart(Root(data), '/', {
'/media': {
'tools.staticdir.on': True,
'tools.staticdir.dir': 'static'
}
})
if __name__ == '__main__':
main(sys.argv[1])
Step 4- พิจารณาแอปพลิเคชันที่ผู้ใช้ป้อนค่าผ่านแบบฟอร์ม รวมสองแบบฟอร์ม - index.html และ submit.html ในแอปพลิเคชัน
Step 5 - ในรหัสด้านบนสำหรับคอนโทรลเลอร์เรามี index()ซึ่งเป็นฟังก์ชันเริ่มต้นและโหลดก่อนหากมีการเรียกตัวควบคุมเฉพาะ
Step 6 - การใช้งานไฟล์ index() วิธีการสามารถเปลี่ยนแปลงได้ดังต่อไปนี้ -
@cherrypy.expose
def index(self):
tmpl = loader.load('index.html')
return tmpl.generate(title='Sample').render('html', doctype='html')
Step 7- สิ่งนี้จะโหลด index.html เมื่อเริ่มต้นแอปพลิเคชันที่กำหนดและนำไปยังสตรีมเอาต์พุตที่กำหนด ไฟล์ index.html มีดังนี้ -
index.html
<!DOCTYPE html >
<html>
<head>
<title>Sample</title>
</head>
<body class = "index">
<div id = "header">
<h1>Sample Application</h1>
</div>
<p>Welcome!</p>
<div id = "footer">
<hr>
</div>
</body>
</html>
Step 8 - สิ่งสำคัญคือต้องเพิ่มวิธีการในคลาสรูทใน controller.py หากคุณต้องการสร้างฟอร์มที่ยอมรับค่าเช่นชื่อและชื่อเรื่อง
@cherrypy.expose
def submit(self, cancel = False, **value):
if cherrypy.request.method == 'POST':
if cancel:
raise cherrypy.HTTPRedirect('/') # to cancel the action
link = Link(**value)
self.data[link.id] = link
raise cherrypy.HTTPRedirect('/')
tmp = loader.load('submit.html')
streamValue = tmp.generate()
return streamValue.render('html', doctype='html')
Step 9 - รหัสที่จะรวมอยู่ใน submit.html มีดังนี้ -
<!DOCTYPE html>
<head>
<title>Input the new link</title>
</head>
<body class = "submit">
<div id = " header">
<h1>Submit new link</h1>
</div>
<form action = "" method = "post">
<table summary = "">
<tr>
<th><label for = " username">Your name:</label></th>
<td><input type = " text" id = " username" name = " username" /></td>
</tr>
<tr>
<th><label for = " url">Link URL:</label></th>
<td><input type = " text" id=" url" name= " url" /></td>
</tr>
<tr>
<th><label for = " title">Title:</label></th>
<td><input type = " text" name = " title" /></td>
</tr>
<tr>
<td></td>
<td>
<input type = " submit" value = " Submit" />
<input type = " submit" name = " cancel" value = "Cancel" />
</td>
</tr>
</table>
</form>
<div id = "footer">
</div>
</body>
</html>
Step 10 - คุณจะได้รับผลลัพธ์ต่อไปนี้ -
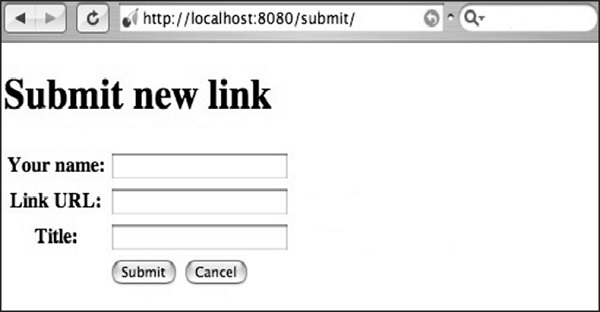
ที่นี่ชื่อวิธีการกำหนดเป็น“ POST” การตรวจสอบข้ามเมธอดที่ระบุในไฟล์เป็นสิ่งสำคัญเสมอ หากเมธอดมีเมธอด“ POST” ควรตรวจสอบค่าในฐานข้อมูลอีกครั้งในฟิลด์ที่เหมาะสม
หากเมธอดมีเมธอด“ GET” ค่าที่จะบันทึกจะปรากฏใน URL