GWT - JUnit Integration
GWT bietet hervorragende Unterstützung für das automatisierte Testen von clientseitigem Code mithilfe des JUnit-Testframeworks. In diesem Artikel werden wir die Integration von GWT und JUNIT demonstrieren.
Laden Sie das Junit-Archiv herunter
JUnit Offizielle Seite - https://www.junit.org
Herunterladen Junit-4.10.jar
Betriebssystem | Archivname |
---|---|
Windows | junit4.10.jar |
Linux | junit4.10.jar |
Mac | junit4.10.jar |
Speichern Sie die heruntergeladene JAR-Datei an einem Ort auf Ihrem Computer. Wir haben es bei gespeichertC:/ > JUNIT
Suchen Sie den GWT-Installationsordner
Betriebssystem | GWT-Installationsordner |
---|---|
Windows | C: \ GWT \ gwt-2.1.0 |
Linux | /usr/local/GWT/gwt-2.1.0 |
Mac | /Library/GWT/gwt-2.1.0 |
GWTTestCase-Klasse
GWT bietet GWTTestCaseBasisklasse, die JUnit-Integration bietet. Durch Ausführen einer kompilierten Klasse, die GWTTestCase unter JUnit erweitert, wird der HtmlUnit-Browser gestartet, der dazu dient, Ihr Anwendungsverhalten während der Testausführung zu emulieren.
GWTTestCase ist eine abgeleitete Klasse von JUnits TestCase und kann mit JUnit TestRunner ausgeführt werden.
Verwenden von webAppCreator
GWT bietet ein spezielles Befehlszeilenprogramm webAppCreator Dies kann einen Starter-Testfall für uns generieren sowie Ameisenziele und Eclipse-Startkonfigurationen zum Testen sowohl im Entwicklungsmodus als auch im Produktionsmodus.
Öffnen Sie die Eingabeaufforderung und gehen Sie zu C:\ > GWT_WORKSPACE > Hier möchten Sie ein neues Projekt mit Testunterstützung erstellen. Führen Sie den folgenden Befehl aus
C:\GWT_WORKSPACE>C:\GWT\gwt-2.1.0\webAppCreator
-out HelloWorld
-junit C:\JUNIT\junit-4.10.jar
com.tutorialspoint.HelloWorld
Bemerkenswerte Punkte
- Wir führen das Befehlszeilenprogramm webAppCreator aus.
- HelloWorld ist der Name des zu erstellenden Projekts
- Die Option -junit weist webAppCreator an, dem Projekt den Junit-Support hinzuzufügen
- com.tutorialspoint.HelloWorld ist der Name des Moduls
Überprüfen Sie die Ausgabe.
Created directory HelloWorld\src
Created directory HelloWorld\war
Created directory HelloWorld\war\WEB-INF
Created directory HelloWorld\war\WEB-INF\lib
Created directory HelloWorld\src\com\tutorialspoint
Created directory HelloWorld\src\com\tutorialspoint\client
Created directory HelloWorld\src\com\tutorialspoint\server
Created directory HelloWorld\src\com\tutorialspoint\shared
Created directory HelloWorld\test\com\tutorialspoint
Created directory HelloWorld\test\com\tutorialspoint\client
Created file HelloWorld\src\com\tutorialspoint\HelloWorld.gwt.xml
Created file HelloWorld\war\HelloWorld.html
Created file HelloWorld\war\HelloWorld.css
Created file HelloWorld\war\WEB-INF\web.xml
Created file HelloWorld\src\com\tutorialspoint\client\HelloWorld.java
Created file
HelloWorld\src\com\tutorialspoint\client\GreetingService.java
Created file
HelloWorld\src\com\tutorialspoint\client\GreetingServiceAsync.java
Created file
HelloWorld\src\com\tutorialspoint\server\GreetingServiceImpl.java
Created file HelloWorld\src\com\tutorialspoint\shared\FieldVerifier.java
Created file HelloWorld\build.xml
Created file HelloWorld\README.txt
Created file HelloWorld\test\com\tutorialspoint\HelloWorldJUnit.gwt.xml
Created file HelloWorld\test\com\tutorialspoint\client\HelloWorldTest.java
Created file HelloWorld\.project
Created file HelloWorld\.classpath
Created file HelloWorld\HelloWorld.launch
Created file HelloWorld\HelloWorldTest-dev.launch
Created file HelloWorld\HelloWorldTest-prod.launch
Grundlegendes zur Testklasse: HelloWorldTest.java
package com.tutorialspoint.client;
import com.tutorialspoint.shared.FieldVerifier;
import com.google.gwt.core.client.GWT;
import com.google.gwt.junit.client.GWTTestCase;
import com.google.gwt.user.client.rpc.AsyncCallback;
import com.google.gwt.user.client.rpc.ServiceDefTarget;
/**
* GWT JUnit tests must extend GWTTestCase.
*/
public class HelloWorldTest extends GWTTestCase {
/**
* must refer to a valid module that sources this class.
*/
public String getModuleName() {
return "com.tutorialspoint.HelloWorldJUnit";
}
/**
* tests the FieldVerifier.
*/
public void testFieldVerifier() {
assertFalse(FieldVerifier.isValidName(null));
assertFalse(FieldVerifier.isValidName(""));
assertFalse(FieldVerifier.isValidName("a"));
assertFalse(FieldVerifier.isValidName("ab"));
assertFalse(FieldVerifier.isValidName("abc"));
assertTrue(FieldVerifier.isValidName("abcd"));
}
/**
* this test will send a request to the server using the greetServer
* method in GreetingService and verify the response.
*/
public void testGreetingService() {
/* create the service that we will test. */
GreetingServiceAsync greetingService =
GWT.create(GreetingService.class);
ServiceDefTarget target = (ServiceDefTarget) greetingService;
target.setServiceEntryPoint(GWT.getModuleBaseURL()
+ "helloworld/greet");
/* since RPC calls are asynchronous, we will need to wait
for a response after this test method returns. This line
tells the test runner to wait up to 10 seconds
before timing out. */
delayTestFinish(10000);
/* send a request to the server. */
greetingService.greetServer("GWT User",
new AsyncCallback<String>() {
public void onFailure(Throwable caught) {
/* The request resulted in an unexpected error. */
fail("Request failure: " + caught.getMessage());
}
public void onSuccess(String result) {
/* verify that the response is correct. */
assertTrue(result.startsWith("Hello, GWT User!"));
/* now that we have received a response, we need to
tell the test runner that the test is complete.
You must call finishTest() after an asynchronous test
finishes successfully, or the test will time out.*/
finishTest();
}
});
}
}
Bemerkenswerte Punkte
Sr.Nr. | Hinweis |
---|---|
1 | Die HelloWorldTest-Klasse wurde im Paket com.tutorialspoint.client im Verzeichnis HelloWorld / test generiert. |
2 | Die HelloWorldTest-Klasse enthält Unit-Testfälle für HelloWorld. |
3 | Die HelloWorldTest-Klasse erweitert die GWTTestCase-Klasse im Paket com.google.gwt.junit.client. |
4 | Die HelloWorldTest-Klasse verfügt über eine abstrakte Methode (getModuleName), die den Namen des GWT-Moduls zurückgeben muss. Für HelloWorld ist dies com.tutorialspoint.HelloWorldJUnit. |
5 | Die HelloWorldTest-Klasse wird mit zwei Beispieltestfällen testFieldVerifier, testSimple, generiert. Wir haben testGreetingService hinzugefügt. |
6 | Diese Methoden verwenden eine der vielen assert * -Funktionen, die sie von der JUnit Assert-Klasse erben, die ein Vorfahr von GWTTestCase ist. |
7 | Die Funktion assertTrue (boolean) bestätigt, dass das übergebene boolesche Argument als true ausgewertet wird. Wenn nicht, schlägt der Test fehl, wenn er in JUnit ausgeführt wird. |
Beispiel für die vollständige Integration von GWT - JUnit
In diesem Beispiel werden Sie durch einfache Schritte geführt, um ein Beispiel für die JUnit-Integration in GWT zu zeigen.
Führen Sie die folgenden Schritte aus, um die oben erstellte GWT-Anwendung zu aktualisieren:
Schritt | Beschreibung |
---|---|
1 | Importieren Sie das Projekt mit dem Namen HelloWorld in Eclipse mithilfe des Assistenten zum Importieren vorhandener Projekte (Datei → Importieren → Allgemein → Vorhandene Projekte in den Arbeitsbereich). |
2 | Ändern Sie HelloWorld.gwt.xml , HelloWorld.css , HelloWorld.html und HelloWorld.java wie unten erläutert. Lassen Sie den Rest der Dateien unverändert. |
3 | Kompilieren Sie die Anwendung und führen Sie sie aus, um das Ergebnis der implementierten Logik zu überprüfen. |
Es folgt die Projektstruktur in Eclipse.

Es folgt der Inhalt des modifizierten Moduldeskriptors src/com.tutorialspoint/HelloWorld.gwt.xml.
<?xml version = "1.0" encoding = "UTF-8"?>
<module rename-to = 'helloworld'>
<!-- Inherit the core Web Toolkit stuff. -->
<inherits name = 'com.google.gwt.user.User'/>
<!-- Inherit the default GWT style sheet. -->
<inherits name = 'com.google.gwt.user.theme.clean.Clean'/>
<!-- Inherit the UiBinder module. -->
<inherits name = "com.google.gwt.uibinder.UiBinder"/>
<!-- Specify the app entry point class. -->
<entry-point class = 'com.tutorialspoint.client.HelloWorld'/>
<!-- Specify the paths for translatable code -->
<source path = 'client'/>
<source path = 'shared'/>
</module>
Im Folgenden finden Sie den Inhalt der geänderten Stylesheet-Datei war/HelloWorld.css.
body {
text-align: center;
font-family: verdana, sans-serif;
}
h1 {
font-size: 2em;
font-weight: bold;
color: #777777;
margin: 40px 0px 70px;
text-align: center;
}
Es folgt der Inhalt der geänderten HTML-Hostdatei war/HelloWorld.html.
<html>
<head>
<title>Hello World</title>
<link rel = "stylesheet" href = "HelloWorld.css"/>
<script language = "javascript" src = "helloworld/helloworld.nocache.js">
</script>
</head>
<body>
<h1>JUnit Integration Demonstration</h1>
<div id = "gwtContainer"></div>
</body>
</html>
Ersetzen Sie den Inhalt von HelloWorld.java in src/com.tutorialspoint/client Paket mit dem folgenden
package com.tutorialspoint.client;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.core.client.GWT;
import com.google.gwt.event.dom.client.ClickEvent;
import com.google.gwt.event.dom.client.ClickHandler;
import com.google.gwt.event.dom.client.KeyCodes;
import com.google.gwt.event.dom.client.KeyUpEvent;
import com.google.gwt.event.dom.client.KeyUpHandler;
import com.google.gwt.user.client.Window;
import com.google.gwt.user.client.rpc.AsyncCallback;
import com.google.gwt.user.client.ui.Button;
import com.google.gwt.user.client.ui.DecoratorPanel;
import com.google.gwt.user.client.ui.HasHorizontalAlignment;
import com.google.gwt.user.client.ui.HorizontalPanel;
import com.google.gwt.user.client.ui.Label;
import com.google.gwt.user.client.ui.RootPanel;
import com.google.gwt.user.client.ui.TextBox;
import com.google.gwt.user.client.ui.VerticalPanel;
public class HelloWorld implements EntryPoint {
public void onModuleLoad() {
/*create UI */
final TextBox txtName = new TextBox();
txtName.setWidth("200");
txtName.addKeyUpHandler(new KeyUpHandler() {
@Override
public void onKeyUp(KeyUpEvent event) {
if(event.getNativeKeyCode() == KeyCodes.KEY_ENTER){
Window.alert(getGreeting(txtName.getValue()));
}
}
});
Label lblName = new Label("Enter your name: ");
Button buttonMessage = new Button("Click Me!");
buttonMessage.addClickHandler(new ClickHandler() {
@Override
public void onClick(ClickEvent event) {
Window.alert(getGreeting(txtName.getValue()));
}
});
HorizontalPanel hPanel = new HorizontalPanel();
hPanel.add(lblName);
hPanel.add(txtName);
hPanel.setCellWidth(lblName, "130");
VerticalPanel vPanel = new VerticalPanel();
vPanel.setSpacing(10);
vPanel.add(hPanel);
vPanel.add(buttonMessage);
vPanel.setCellHorizontalAlignment(buttonMessage,
HasHorizontalAlignment.ALIGN_RIGHT);
DecoratorPanel panel = new DecoratorPanel();
panel.add(vPanel);
// Add widgets to the root panel.
RootPanel.get("gwtContainer").add(panel);
}
public String getGreeting(String name){
return "Hello "+name+"!";
}
}
Ersetzen Sie den Inhalt von HelloWorldTest.java in test/com.tutorialspoint/client Paket mit dem folgenden
package com.tutorialspoint.client;
import com.tutorialspoint.shared.FieldVerifier;
import com.google.gwt.core.client.GWT;
import com.google.gwt.junit.client.GWTTestCase;
import com.google.gwt.user.client.rpc.AsyncCallback;
import com.google.gwt.user.client.rpc.ServiceDefTarget;
/**
* GWT JUnit tests must extend GWTTestCase.
*/
public class HelloWorldTest extends GWTTestCase {
/**
* must refer to a valid module that sources this class.
*/
public String getModuleName() {
return "com.tutorialspoint.HelloWorldJUnit";
}
/**
* tests the FieldVerifier.
*/
public void testFieldVerifier() {
assertFalse(FieldVerifier.isValidName(null));
assertFalse(FieldVerifier.isValidName(""));
assertFalse(FieldVerifier.isValidName("a"));
assertFalse(FieldVerifier.isValidName("ab"));
assertFalse(FieldVerifier.isValidName("abc"));
assertTrue(FieldVerifier.isValidName("abcd"));
}
/**
* this test will send a request to the server using the greetServer
* method in GreetingService and verify the response.
*/
public void testGreetingService() {
/* create the service that we will test. */
GreetingServiceAsync greetingService =
GWT.create(GreetingService.class);
ServiceDefTarget target = (ServiceDefTarget) greetingService;
target.setServiceEntryPoint(GWT.getModuleBaseURL()
+ "helloworld/greet");
/* since RPC calls are asynchronous, we will need to wait
for a response after this test method returns. This line
tells the test runner to wait up to 10 seconds
before timing out. */
delayTestFinish(10000);
/* send a request to the server. */
greetingService.greetServer("GWT User",
new AsyncCallback<String>() {
public void onFailure(Throwable caught) {
/* The request resulted in an unexpected error. */
fail("Request failure: " + caught.getMessage());
}
public void onSuccess(String result) {
/* verify that the response is correct. */
assertTrue(result.startsWith("Hello, GWT User!"));
/* now that we have received a response, we need to
tell the test runner that the test is complete.
You must call finishTest() after an asynchronous test
finishes successfully, or the test will time out.*/
finishTest();
}
});
/**
* tests the getGreeting method.
*/
public void testGetGreeting() {
HelloWorld helloWorld = new HelloWorld();
String name = "Robert";
String expectedGreeting = "Hello "+name+"!";
assertEquals(expectedGreeting,helloWorld.getGreeting(name));
}
}
}
Führen Sie Testfälle in Eclipse mit generierten Startkonfigurationen aus
Wir werden Unit-Tests in Eclipse unter Verwendung der von webAppCreator generierten Startkonfigurationen sowohl für den Entwicklungsmodus als auch für den Produktionsmodus ausführen.
Führen Sie den JUnit-Test im Entwicklungsmodus aus
- Wählen Sie in der Eclipse-Menüleiste Ausführen → Konfigurationen ausführen ...
- Wählen Sie im Abschnitt JUnit die Option HelloWorldTest-dev aus
- Um die Änderungen an den Argumenten zu speichern, drücken Sie Übernehmen
- Drücken Sie Ausführen, um den Test auszuführen
Wenn mit Ihrer Anwendung alles in Ordnung ist, führt dies zu folgendem Ergebnis:
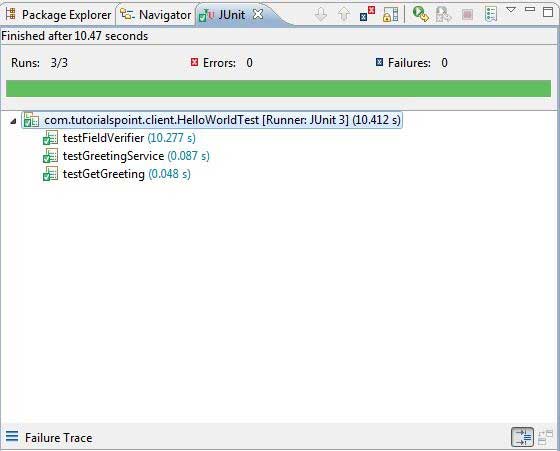
Führen Sie den JUnit-Test im Produktionsmodus aus
- Wählen Sie in der Eclipse-Menüleiste Ausführen → Konfigurationen ausführen ...
- Wählen Sie im Abschnitt JUnit die Option HelloWorldTest-prod aus
- Um die Änderungen an den Argumenten zu speichern, drücken Sie Übernehmen
- Drücken Sie Ausführen, um den Test auszuführen
Wenn mit Ihrer Anwendung alles in Ordnung ist, führt dies zu folgendem Ergebnis:
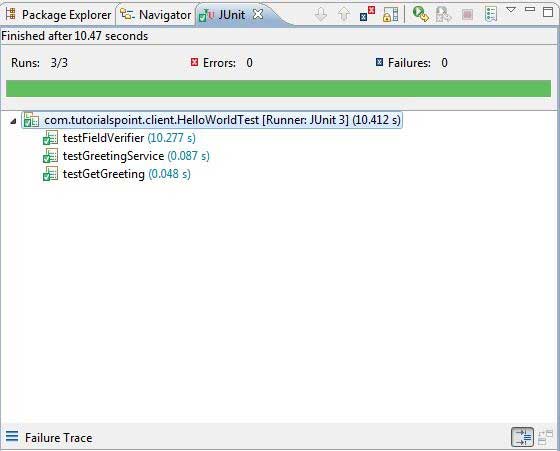