Desarrollo de Windows10: comunicación de aplicaciones
La comunicación de aplicación a aplicación significa que su aplicación puede hablar o comunicarse con otra aplicación que esté instalada en el mismo dispositivo. Esta no es una característica nueva en la aplicación Plataforma universal de Windows (UWP) y también estaba disponible en Windows 8.1.
En Windows 10, se introducen algunas formas nuevas y mejoradas para comunicarse fácilmente entre aplicaciones en el mismo dispositivo. La comunicación entre dos aplicaciones puede realizarse de las siguientes formas:
- Una aplicación que inicia otra aplicación con algunos datos.
- Las aplicaciones simplemente intercambian datos sin iniciar nada.
La principal ventaja de la comunicación de aplicación a aplicación es que puede dividir las aplicaciones en trozos más pequeños, que se pueden mantener, actualizar y consumir fácilmente.
Preparando su aplicación
Si sigue los pasos que se indican a continuación, otras aplicaciones pueden iniciar su aplicación.
Agregue una declaración de protocolo en el manifiesto del paquete de la aplicación.
Haga doble clic en el Package.appxmanifest archivo, que está disponible en el Explorador de soluciones como se muestra a continuación.
Ve a la Declaration pestaña y escriba el Nombre del protocolo como se muestra a continuación.
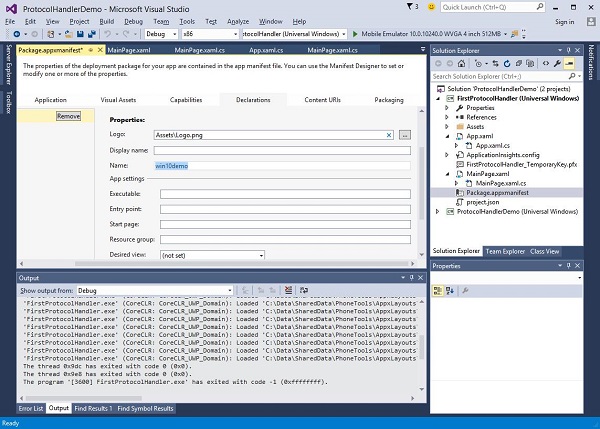
El siguiente paso es agregar el activation código, por lo que la aplicación puede responder adecuadamente cuando la inicie la otra aplicación.
Para responder a las activaciones del protocolo, debemos anular la OnActivatedmétodo de la clase de activación. Entonces, agregue el siguiente código enApp.xaml.cs archivo.
protected override void OnActivated(IActivatedEventArgs args) {
ProtocolActivatedEventArgs protocolArgs = args as ProtocolActivatedEventArgs;
if (args != null){
Frame rootFrame = Window.Current.Content as Frame;
// Do not repeat app initialization when the Window already has content,
// just ensure that the window is active
if (rootFrame == null){
// Create a Frame to act as the navigation context and navigate to the first page
rootFrame = new Frame();
// Set the default language
rootFrame.Language = Windows.Globalization.ApplicationLanguages.Languages[0];
rootFrame.NavigationFailed += OnNavigationFailed;
// Place the frame in the current Window
Window.Current.Content = rootFrame;
}
if (rootFrame.Content == null){
// When the navigation stack isn't restored, navigate to the
// first page, configuring the new page by passing required
// information as a navigation parameter
rootFrame.Navigate(typeof(MainPage), null);
}
// Ensure the current window is active
Window.Current.Activate();
}
}
Para iniciar la aplicación, simplemente puede usar el Launcher.LaunchUriAsync método, que lanzará la aplicación con el protocolo especificado en este método.
await Windows.System.Launcher.LaunchUriAsync(new Uri("win10demo:?SomeData=123"));
Entendamos esto con un ejemplo simple en el que tenemos dos aplicaciones para UWP con ProtocolHandlerDemo y FirstProtocolHandler.
En este ejemplo, el ProtocolHandlerDemo La aplicación contiene un botón y al hacer clic en el botón, se abrirá el FirstProtocolHandler solicitud.
El código XAML en la aplicación ProtocolHandlerDemo, que contiene un botón, se muestra a continuación.
<Page
x:Class = "ProtocolHandlerDemo.MainPage"
xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local = "using:ProtocolHandlerDemo"
xmlns:d = "http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable = "d">
<Grid Background = "{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Button x:Name = "LaunchButton" Content = " Launch First Protocol App"
FontSize = "24" HorizontalAlignment = "Center"
Click = "LaunchButton_Click"/>
</Grid>
</Page>
A continuación se muestra el código C #, en el que se implementa el evento de clic en el botón.
using System;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
// The Blank Page item template is documented at
http://go.microsoft.com/fwlink/?LinkId=402352&clcid=0x409
namespace ProtocolHandlerDemo {
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class MainPage : Page {
public MainPage(){
this.InitializeComponent();
}
private async void LaunchButton_Click(object sender, RoutedEventArgs e) {
await Windows.System.Launcher.LaunchUriAsync(new
Uri("win10demo:?SomeData=123"));
}
}
}
Ahora echemos un vistazo al FirstProtocolHandlertabla de aplicaciones. A continuación se muestra el código XAML en el que se crea un bloque de texto con algunas propiedades.
<Page
x:Class = "FirstProtocolHandler.MainPage"
xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local = "using:FirstProtocolHandler"
xmlns:d = "http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable = "d">
<Grid Background = "{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock Text = "You have successfully launch First Protocol Application"
TextWrapping = "Wrap" Style = "{StaticResource SubtitleTextBlockStyle}"
Margin = "30,39,0,0" VerticalAlignment = "Top" HorizontalAlignment = "Left"
Height = "100" Width = "325"/>
</Grid>
</Page>
La implementación de C # del App.xaml.cs archivo en el que OnActicatedse anula se muestra a continuación. Agregue el siguiente código dentro de la clase App en elApp.xaml.cs archivo.
protected override void OnActivated(IActivatedEventArgs args) {
ProtocolActivatedEventArgs protocolArgs = args as ProtocolActivatedEventArgs;
if (args != null) {
Frame rootFrame = Window.Current.Content as Frame;
// Do not repeat app initialization when the Window already has content,
// just ensure that the window is active
if (rootFrame == null) {
// Create a Frame to act as the navigation context and navigate to
the first page
rootFrame = new Frame();
// Set the default language
rootFrame.Language = Windows.Globalization.ApplicationLanguages.Languages[0];
rootFrame.NavigationFailed += OnNavigationFailed;
// Place the frame in the current Window
Window.Current.Content = rootFrame;
}
if (rootFrame.Content == null) {
// When the navigation stack isn't restored navigate to the
// first page, configuring the new page by passing required
// information as a navigation parameter
rootFrame.Navigate(typeof(MainPage), null);
}
// Ensure the current window is active
Window.Current.Activate();
}
}
Cuando compila y ejecuta el ProtocolHandlerDemo aplicación en un emulador, verá la siguiente ventana.
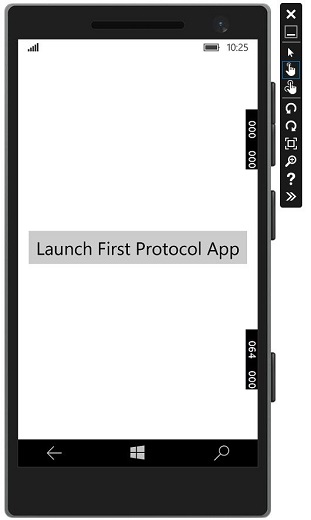
Ahora, cuando haga clic en el botón, se abrirá el FirstProtocolHandler aplicación como se muestra a continuación.
