JSF-国際化
国際化は、ステータスメッセージ、GUIコンポーネントのラベル、通貨、日付がプログラムにハードコーディングされていない手法です。代わりに、ソースコードの外部のリソースバンドルに保存され、動的に取得されます。JSFは、リソースバンドルを処理するための非常に便利な方法を提供します。
JSFアプリケーションを内部化するには、次の手順が必要です。
ステップ1:プロパティファイルを定義する
ロケールごとにプロパティファイルを作成します。名前は<file-name> _ <locale> .properties形式である必要があります。
デフォルトのロケールはファイル名で省略できます。
messages.properties
greeting = Hello World!
messages_fr.properties
greeting = Bonjour tout le monde!
ステップ2:faces-config.xmlを更新します
顔-config.xml
<application>
<locale-config>
<default-locale>en</default-locale>
<supported-locale>fr</supported-locale>
</locale-config>
<resource-bundle>
<base-name>com.tutorialspoint.messages</base-name>
<var>msg</var>
</resource-bundle>
</application>
ステップ3:resource-bundlevarを使用する
home.xhtml
<h:outputText value = "#{msg['greeting']}" />
アプリケーション例
JSFの国際化をテストするためのテストJSFアプリケーションを作成しましょう。
ステップ | 説明 |
---|---|
1 | JSF-最初のアプリケーションの章で説明されているように、パッケージcom.tutorialspoint.testの下にhelloworldという名前のプロジェクトを作成します。 |
2 | src→maiフォルダの下にresourcesフォルダを作成します。 |
3 | src→main→resourcesフォルダーの下にcomフォルダーを作成します。 |
4 | src→main→resources→comフォルダーの下にtutorialspointフォルダーを作成します。 |
5 | src→main→resources→com→tutorialspointフォルダーの下にmessages.propertiesファイルを作成します。以下に説明するように変更します。 |
6 | src→main→resources→com→tutorialspointフォルダーの下にmessages_fr.propertiesファイルを作成します。以下に説明するように変更します。 |
7 | 以下で説明するように、古いWEB-INFfでfaces-config.xmlを作成します。 |
8 | 以下で説明するように、パッケージcom.tutorialspoint.testの下にUserData.javaを作成します。 |
9 | 以下で説明するようにhome.xhtmlを変更します。残りのファイルは変更しないでください。 |
10 | アプリケーションをコンパイルして実行し、ビジネスロジックが要件に従って機能していることを確認します。 |
11 | 最後に、warファイルの形式でアプリケーションをビルドし、Apache TomcatWebサーバーにデプロイします。 |
12 | 最後のステップで以下に説明するように、適切なURLを使用してWebアプリケーションを起動します。 |
messages.properties
greeting = Hello World!
messages_fr.properties
greeting = Bonjour tout le monde!
顔-config.xml
<?xml version = "1.0" encoding = "UTF-8"?>
<faces-config
xmlns = "http://java.sun.com/xml/ns/javaee"
xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation = "http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-facesconfig_2_0.xsd"
version = "2.0">
<application>
<locale-config>
<default-locale>en</default-locale>
<supported-locale>fr</supported-locale>
</locale-config>
<resource-bundle>
<base-name>com.tutorialspoint.messages</base-name>
<var>msg</var>
</resource-bundle>
</application>
</faces-config>
UserData.java
package com.tutorialspoint.test;
import java.io.Serializable;
import java.util.LinkedHashMap;
import java.util.Locale;
import java.util.Map;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.SessionScoped;
import javax.faces.context.FacesContext;
import javax.faces.event.ValueChangeEvent;
@ManagedBean(name = "userData", eager = true)
@SessionScoped
public class UserData implements Serializable {
private static final long serialVersionUID = 1L;
private String locale;
private static Map<String,Object> countries;
static {
countries = new LinkedHashMap<String,Object>();
countries.put("English", Locale.ENGLISH);
countries.put("French", Locale.FRENCH);
}
public Map<String, Object> getCountries() {
return countries;
}
public String getLocale() {
return locale;
}
public void setLocale(String locale) {
this.locale = locale;
}
//value change event listener
public void localeChanged(ValueChangeEvent e) {
String newLocaleValue = e.getNewValue().toString();
for (Map.Entry<String, Object> entry : countries.entrySet()) {
if(entry.getValue().toString().equals(newLocaleValue)) {
FacesContext.getCurrentInstance()
.getViewRoot().setLocale((Locale)entry.getValue());
}
}
}
}
home.xhtml
<?xml version = "1.0" encoding = "UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns = "http://www.w3.org/1999/xhtml"
xmlns:h = "http://java.sun.com/jsf/html"
xmlns:f = "http://java.sun.com/jsf/core">
<h:head>
<title>JSF tutorial</title>
</h:head>
<h:body>
<h2>Internalization Language Example</h2>
<h:form>
<h3><h:outputText value = "#{msg['greeting']}" /></h3>
<h:panelGrid columns = "2">
Language :
<h:selectOneMenu value = "#{userData.locale}" onchange = "submit()"
valueChangeListener = "#{userData.localeChanged}">
<f:selectItems value = "#{userData.countries}" />
</h:selectOneMenu>
</h:panelGrid>
</h:form>
</h:body>
</html>
すべての変更を行う準備ができたら、JSF-最初のアプリケーションの章で行ったように、アプリケーションをコンパイルして実行しましょう。アプリケーションに問題がない場合は、次の結果が得られます。
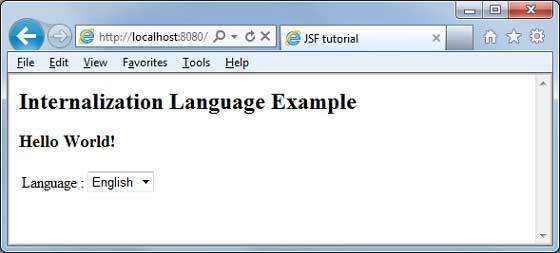
ドロップダウンから言語を変更します。次の出力が表示されます。
