NumPy-クイックガイド
NumPyはPythonパッケージです。'NumericalPython'の略です。これは、多次元配列オブジェクトと、配列を処理するためのルーチンのコレクションで構成されるライブラリです。
NumericNumPyの祖先である、はJimHuguninによって開発されました。いくつかの追加機能を備えた別のパッケージNumarrayも開発されました。2005年、Travis Oliphantは、Numarrayの機能をNumericパッケージに組み込んでNumPyパッケージを作成しました。このオープンソースプロジェクトには多くの貢献者がいます。
NumPyを使用した操作
NumPyを使用すると、開発者は次の操作を実行できます-
配列に対する数学的および論理演算。
フーリエ変換と形状操作のためのルーチン。
線形代数に関連する操作。NumPyには、線形代数と乱数生成のための関数が組み込まれています。
NumPy –MatLabの代替品
NumPyは、次のようなパッケージと一緒に使用されることがよくあります。 SciPy (Scientific Python)と Mat−plotlib(プロットライブラリ)。この組み合わせは、テクニカルコンピューティングで人気のあるプラットフォームであるMatLabの代わりとして広く使用されています。ただし、MatLabに代わるPythonは、より現代的で完全なプログラミング言語と見なされています。
これはオープンソースであり、NumPyの追加の利点です。
標準のPythonディストリビューションは、NumPyモジュールにバンドルされていません。軽量の代替手段は、人気のあるPythonパッケージインストーラーを使用してNumPyをインストールすることです。pip。
pip install numpy
NumPyを有効にする最良の方法は、オペレーティングシステムに固有のインストール可能なバイナリパッケージを使用することです。これらのバイナリには、完全なSciPyスタック(NumPy、SciPy、matplotlib、IPython、SymPy、およびnoseパッケージとコアPythonを含む)が含まれています。
ウィンドウズ
アナコンダ(から https://www.continuum.io)はSciPyスタック用の無料のPythonディストリビューションです。LinuxとMacでも利用できます。
キャノピー(https://www.enthought.com/products/canopy/)は、Windows、Linux、およびMac用の完全なSciPyスタックを備えた無料および商用の配布として利用できます。
Python(x、y):これはSciPyスタックとWindowsOS用のSpyderIDEを備えた無料のPythonディストリビューションです。(からダウンロード可能https://www.python-xy.github.io/)
Linux
それぞれのLinuxディストリビューションのパッケージマネージャーは、SciPyスタックに1つ以上のパッケージをインストールするために使用されます。
Ubuntuの場合
sudo apt-get install python-numpy
python-scipy python-matplotlibipythonipythonnotebook python-pandas
python-sympy python-nose
Fedoraの場合
sudo yum install numpyscipy python-matplotlibipython
python-pandas sympy python-nose atlas-devel
ソースからの構築
Core Python(2.6.x、2.7.x、および3.2.x以降)はdistutilsとともにインストールする必要があり、zlibモジュールを有効にする必要があります。
GNU gcc(4.2以降)Cコンパイラが使用可能である必要があります。
NumPyをインストールするには、次のコマンドを実行します。
Python setup.py install
NumPyモジュールが正しくインストールされているかどうかをテストするには、Pythonプロンプトからインポートしてみてください。
import numpy
インストールされていない場合、以下のエラーメッセージが表示されます。
Traceback (most recent call last):
File "<pyshell#0>", line 1, in <module>
import numpy
ImportError: No module named 'numpy'
または、NumPyパッケージは次の構文を使用してインポートされます-
import numpy as np
NumPyで定義されている最も重要なオブジェクトは、と呼ばれるN次元配列型です。 ndarray。同じタイプのアイテムのコレクションについて説明します。コレクション内のアイテムには、ゼロベースのインデックスを使用してアクセスできます。
ndarray内のすべてのアイテムは、メモリ内の同じサイズのブロックを取ります。ndarrayの各要素は、データ型オブジェクトのオブジェクトです(dtype)。
(スライスによって)ndarrayオブジェクトから抽出されたアイテムは、配列スカラータイプの1つのPythonオブジェクトによって表されます。次の図は、ndarray、データ型オブジェクト(dtype)、および配列スカラー型の関係を示しています。

ndarrayクラスのインスタンスは、チュートリアルで後述するさまざまな配列作成ルーチンによって構築できます。基本的なndarrayは、NumPyの配列関数を使用して次のように作成されます-
numpy.array
配列インターフェイスを公開するオブジェクトから、または配列を返すメソッドからndarrayを作成します。
numpy.array(object, dtype = None, copy = True, order = None, subok = False, ndmin = 0)
上記のコンストラクターは次のパラメーターを取ります-
シニア番号 | パラメータと説明 |
---|---|
1 | object 配列インターフェイスメソッドを公開するオブジェクトは、配列または(ネストされた)シーケンスを返します。 |
2 | dtype 配列の必要なデータ型、オプション |
3 | copy オプション。デフォルト(true)では、オブジェクトはコピーされます |
4 | order C(行メジャー)またはF(列メジャー)またはA(任意)(デフォルト) |
5 | subok デフォルトでは、返される配列は強制的に基本クラスの配列になります。trueの場合、通過したサブクラス |
6 | ndmin 結果の配列の最小次元を指定します |
以下の例を見て、理解を深めてください。
例1
import numpy as np
a = np.array([1,2,3])
print a
出力は次のとおりです-
[1, 2, 3]
例2
# more than one dimensions
import numpy as np
a = np.array([[1, 2], [3, 4]])
print a
出力は次のとおりです-
[[1, 2]
[3, 4]]
例3
# minimum dimensions
import numpy as np
a = np.array([1, 2, 3,4,5], ndmin = 2)
print a
出力は次のとおりです-
[[1, 2, 3, 4, 5]]
例4
# dtype parameter
import numpy as np
a = np.array([1, 2, 3], dtype = complex)
print a
出力は次のとおりです-
[ 1.+0.j, 2.+0.j, 3.+0.j]
ザ・ ndarrayオブジェクトは、コンピュータメモリの連続した1次元セグメントと、各アイテムをメモリブロック内の場所にマップするインデックススキームと組み合わせて構成されます。メモリブロックは、要素を行優先の順序(Cスタイル)または列優先の順序(FORTRANまたはMatLabスタイル)で保持します。
NumPyは、Pythonよりもはるかに多様な数値型をサポートしています。次の表は、NumPyで定義されているさまざまなスカラーデータ型を示しています。
シニア番号 | データ型と説明 |
---|---|
1 | bool_ バイトとして格納されたブール値(TrueまたはFalse) |
2 | int_ デフォルトの整数型(C longと同じ、通常はint64またはint32) |
3 | intc C intと同じ(通常はint32またはint64) |
4 | intp インデックス作成に使用される整数(C ssize_tと同じ、通常はint32またはint64) |
5 | int8 バイト(-128〜127) |
6 | int16 整数(-32768〜32767) |
7 | int32 整数(-2147483648から2147483647) |
8 | int64 整数(-9223372036854775808から9223372036854775807) |
9 | uint8 符号なし整数(0から255) |
10 | uint16 符号なし整数(0〜65535) |
11 | uint32 符号なし整数(0から4294967295) |
12 | uint64 符号なし整数(0から18446744073709551615) |
13 | float_ float64の省略形 |
14 | float16 半精度浮動小数点数:符号ビット、5ビットの指数、10ビットの仮数 |
15 | float32 単精度浮動小数点数:符号ビット、8ビット指数、23ビット仮数 |
16 | float64 倍精度浮動小数点数:符号ビット、11ビットの指数、52ビットの仮数 |
17 | complex_ complex128の省略形 |
18 | complex64 2つの32ビットフロート(実数成分と虚数成分)で表される複素数 |
19 | complex128 2つの64ビットフロート(実数成分と虚数成分)で表される複素数 |
NumPy数値型は、dtype(データ型)オブジェクトのインスタンスであり、それぞれに固有の特性があります。dtypeは、np.bool_、np.float32などとして使用できます。
データ型オブジェクト(dtype)
データ型オブジェクトは、次の側面に応じて、配列に対応するメモリの固定ブロックの解釈を記述します。
データのタイプ(整数、float、またはPythonオブジェクト)
データのサイズ
バイトオーダー(リトルエンディアンまたはビッグエンディアン)
構造化タイプの場合、フィールドの名前、各フィールドのデータタイプ、および各フィールドが取得するメモリブロックの一部。
データ型がサブ配列の場合、その形状とデータ型
バイト順序は、データ型の前に「<」または「>」を付けることによって決定されます。「<」は、エンコーディングがリトルエンディアンであることを意味します(最下位は最小アドレスに格納されます)。'>'は、エンコーディングがビッグエンディアンであることを意味します(最上位バイトは最小アドレスに格納されます)。
dtypeオブジェクトは、次の構文を使用して作成されます-
numpy.dtype(object, align, copy)
パラメータは次のとおりです。
Object −データ型オブジェクトに変換されます
Align − trueの場合、フィールドにパディングを追加して、C-structと同様にします。
Copy−dtypeオブジェクトの新しいコピーを作成します。falseの場合、結果は組み込みデータ型オブジェクトへの参照になります
例1
# using array-scalar type
import numpy as np
dt = np.dtype(np.int32)
print dt
出力は次のとおりです-
int32
例2
#int8, int16, int32, int64 can be replaced by equivalent string 'i1', 'i2','i4', etc.
import numpy as np
dt = np.dtype('i4')
print dt
出力は次のとおりです-
int32
例3
# using endian notation
import numpy as np
dt = np.dtype('>i4')
print dt
出力は次のとおりです-
>i4
次の例は、構造化データ型の使用法を示しています。ここでは、フィールド名と対応するスカラーデータ型を宣言します。
例4
# first create structured data type
import numpy as np
dt = np.dtype([('age',np.int8)])
print dt
出力は次のとおりです-
[('age', 'i1')]
例5
# now apply it to ndarray object
import numpy as np
dt = np.dtype([('age',np.int8)])
a = np.array([(10,),(20,),(30,)], dtype = dt)
print a
出力は次のとおりです-
[(10,) (20,) (30,)]
例6
# file name can be used to access content of age column
import numpy as np
dt = np.dtype([('age',np.int8)])
a = np.array([(10,),(20,),(30,)], dtype = dt)
print a['age']
出力は次のとおりです-
[10 20 30]
例7
次の例では、と呼ばれる構造化データ型を定義しています。 student 文字列フィールド「名前」を使用して、 integer field 「年齢」と float field「マーク」。このdtypeはndarrayオブジェクトに適用されます。
import numpy as np
student = np.dtype([('name','S20'), ('age', 'i1'), ('marks', 'f4')])
print student
出力は次のとおりです-
[('name', 'S20'), ('age', 'i1'), ('marks', '<f4')])
例8
import numpy as np
student = np.dtype([('name','S20'), ('age', 'i1'), ('marks', 'f4')])
a = np.array([('abc', 21, 50),('xyz', 18, 75)], dtype = student)
print a
出力は次のとおりです-
[('abc', 21, 50.0), ('xyz', 18, 75.0)]
各組み込みデータ型には、それを一意に識別する文字コードがあります。
'b' −ブール値
'i' −(符号付き)整数
'u' −符号なし整数
'f' −浮動小数点
'c' −複雑な浮動小数点
'm' −タイムデルタ
'M' −日時
'O' −(Python)オブジェクト
'S', 'a' −(バイト)文字列
'U' − Unicode
'V' −生データ(void)
この章では、NumPyのさまざまな配列属性について説明します。
ndarray.shape
この配列属性は、配列の次元で構成されるタプルを返します。配列のサイズを変更するためにも使用できます。
例1
import numpy as np
a = np.array([[1,2,3],[4,5,6]])
print a.shape
出力は次のとおりです-
(2, 3)
例2
# this resizes the ndarray
import numpy as np
a = np.array([[1,2,3],[4,5,6]])
a.shape = (3,2)
print a
出力は次のとおりです-
[[1, 2]
[3, 4]
[5, 6]]
例3
NumPyは、配列のサイズを変更するための形状変更機能も提供します。
import numpy as np
a = np.array([[1,2,3],[4,5,6]])
b = a.reshape(3,2)
print b
出力は次のとおりです-
[[1, 2]
[3, 4]
[5, 6]]
ndarray.ndim
この配列属性は、配列の次元数を返します。
例1
# an array of evenly spaced numbers
import numpy as np
a = np.arange(24)
print a
出力は次のとおりです-
[0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23]
例2
# this is one dimensional array
import numpy as np
a = np.arange(24)
a.ndim
# now reshape it
b = a.reshape(2,4,3)
print b
# b is having three dimensions
出力は次のとおりです-
[[[ 0, 1, 2]
[ 3, 4, 5]
[ 6, 7, 8]
[ 9, 10, 11]]
[[12, 13, 14]
[15, 16, 17]
[18, 19, 20]
[21, 22, 23]]]
numpy.itemsize
この配列属性は、配列の各要素の長さをバイト単位で返します。
例1
# dtype of array is int8 (1 byte)
import numpy as np
x = np.array([1,2,3,4,5], dtype = np.int8)
print x.itemsize
出力は次のとおりです-
1
例2
# dtype of array is now float32 (4 bytes)
import numpy as np
x = np.array([1,2,3,4,5], dtype = np.float32)
print x.itemsize
出力は次のとおりです-
4
numpy.flags
ndarrayオブジェクトには次の属性があります。その現在の値は、この関数によって返されます。
シニア番号 | 属性と説明 |
---|---|
1 | C_CONTIGUOUS (C) データは、単一のCスタイルの連続したセグメントにあります |
2 | F_CONTIGUOUS (F) データは、単一のFortranスタイルの連続したセグメントにあります |
3 | OWNDATA (O) 配列は、使用するメモリを所有しているか、別のオブジェクトからメモリを借用しています |
4 | WRITEABLE (W) データ領域への書き込みが可能です。これをFalseに設定すると、データがロックされ、読み取り専用になります |
5 | ALIGNED (A) データとすべての要素は、ハードウェアに合わせて適切に配置されます |
6 | UPDATEIFCOPY (U) この配列は、他の配列のコピーです。この配列の割り当てが解除されると、基本配列はこの配列の内容で更新されます |
例
次の例は、フラグの現在の値を示しています。
import numpy as np
x = np.array([1,2,3,4,5])
print x.flags
出力は次のとおりです-
C_CONTIGUOUS : True
F_CONTIGUOUS : True
OWNDATA : True
WRITEABLE : True
ALIGNED : True
UPDATEIFCOPY : False
新しい ndarray オブジェクトは、次の配列作成ルーチンのいずれかによって、または低レベルのndarrayコンストラクターを使用して構築できます。
numpy.empty
指定された形状とdtypeの初期化されていない配列を作成します。次のコンストラクターを使用します-
numpy.empty(shape, dtype = float, order = 'C')
コンストラクターは次のパラメーターを取ります。
シニア番号 | パラメータと説明 |
---|---|
1 | Shape intまたはintのタプルの空の配列の形状 |
2 | Dtype 必要な出力データ型。オプション |
3 | Order Cスタイルの行メジャー配列の場合は「C」、FORTRANスタイルの列メジャー配列の場合は「F」 |
例
次のコードは、空の配列の例を示しています。
import numpy as np
x = np.empty([3,2], dtype = int)
print x
出力は次のとおりです-
[[22649312 1701344351]
[1818321759 1885959276]
[16779776 156368896]]
Note −配列内の要素は、初期化されていないため、ランダムな値を示します。
numpy.zeros
ゼロで埋められた、指定されたサイズの新しい配列を返します。
numpy.zeros(shape, dtype = float, order = 'C')
コンストラクターは次のパラメーターを取ります。
シニア番号 | パラメータと説明 |
---|---|
1 | Shape intまたはintのシーケンス内の空の配列の形状 |
2 | Dtype 必要な出力データ型。オプション |
3 | Order Cスタイルの行メジャー配列の場合は「C」、FORTRANスタイルの列メジャー配列の場合は「F」 |
例1
# array of five zeros. Default dtype is float
import numpy as np
x = np.zeros(5)
print x
出力は次のとおりです-
[ 0. 0. 0. 0. 0.]
例2
import numpy as np
x = np.zeros((5,), dtype = np.int)
print x
これで、出力は次のようになります。
[0 0 0 0 0]
例3
# custom type
import numpy as np
x = np.zeros((2,2), dtype = [('x', 'i4'), ('y', 'i4')])
print x
次の出力が生成されます-
[[(0,0)(0,0)]
[(0,0)(0,0)]]
numpy.ones
指定されたサイズとタイプの新しい配列を返します。
numpy.ones(shape, dtype = None, order = 'C')
コンストラクターは次のパラメーターを取ります。
シニア番号 | パラメータと説明 |
---|---|
1 | Shape intまたはintのタプルの空の配列の形状 |
2 | Dtype 必要な出力データ型。オプション |
3 | Order Cスタイルの行メジャー配列の場合は「C」、FORTRANスタイルの列メジャー配列の場合は「F」 |
例1
# array of five ones. Default dtype is float
import numpy as np
x = np.ones(5)
print x
出力は次のとおりです-
[ 1. 1. 1. 1. 1.]
例2
import numpy as np
x = np.ones([2,2], dtype = int)
print x
これで、出力は次のようになります。
[[1 1]
[1 1]]
この章では、既存のデータから配列を作成する方法について説明します。
numpy.asarray
この関数は、パラメーターが少ないことを除けば、numpy.arrayに似ています。このルーチンは、Pythonシーケンスをndarrayに変換するのに役立ちます。
numpy.asarray(a, dtype = None, order = None)
コンストラクターは次のパラメーターを取ります。
シニア番号 | パラメータと説明 |
---|---|
1 | a リスト、タプルのリスト、タプル、タプルのタプル、リストのタプルなど、任意の形式でデータを入力します |
2 | dtype デフォルトでは、入力データのデータ型は結果のndarrayに適用されます |
3 | order C(行メジャー)またはF(列メジャー)。Cがデフォルトです |
次の例は、 asarray 関数。
例1
# convert list to ndarray
import numpy as np
x = [1,2,3]
a = np.asarray(x)
print a
その出力は次のようになります-
[1 2 3]
例2
# dtype is set
import numpy as np
x = [1,2,3]
a = np.asarray(x, dtype = float)
print a
これで、出力は次のようになります。
[ 1. 2. 3.]
例3
# ndarray from tuple
import numpy as np
x = (1,2,3)
a = np.asarray(x)
print a
その出力は次のようになります-
[1 2 3]
例4
# ndarray from list of tuples
import numpy as np
x = [(1,2,3),(4,5)]
a = np.asarray(x)
print a
ここで、出力は次のようになります-
[(1, 2, 3) (4, 5)]
numpy.frombuffer
この関数は、バッファーを1次元配列として解釈します。バッファインターフェイスを公開するオブジェクトは、パラメータとして使用され、ndarray。
numpy.frombuffer(buffer, dtype = float, count = -1, offset = 0)
コンストラクターは次のパラメーターを取ります。
シニア番号 | パラメータと説明 |
---|---|
1 | buffer バッファインターフェイスを公開するオブジェクト |
2 | dtype 返されたndarrayのデータ型。デフォルトはfloat |
3 | count 読み取るアイテムの数。デフォルトは-1はすべてのデータを意味します |
4 | offset 読み取る開始位置。デフォルトは0です |
例
次の例は、 frombuffer 関数。
import numpy as np
s = 'Hello World'
a = np.frombuffer(s, dtype = 'S1')
print a
これがその出力です-
['H' 'e' 'l' 'l' 'o' ' ' 'W' 'o' 'r' 'l' 'd']
numpy.fromiter
この関数は、 ndarray反復可能なオブジェクトからのオブジェクト。この関数によって、新しい1次元配列が返されます。
numpy.fromiter(iterable, dtype, count = -1)
ここで、コンストラクターは次のパラメーターを取ります。
シニア番号 | パラメータと説明 |
---|---|
1 | iterable 反復可能なオブジェクト |
2 | dtype 結果の配列のデータ型 |
3 | count イテレータから読み取るアイテムの数。デフォルトは-1で、これはすべてのデータが読み取られることを意味します |
次の例は、組み込みの使用方法を示しています range()リストオブジェクトを返す関数。このリストのイテレータは、ndarray オブジェクト。
例1
# create list object using range function
import numpy as np
list = range(5)
print list
その出力は次のとおりです-
[0, 1, 2, 3, 4]
例2
# obtain iterator object from list
import numpy as np
list = range(5)
it = iter(list)
# use iterator to create ndarray
x = np.fromiter(it, dtype = float)
print x
これで、出力は次のようになります。
[0. 1. 2. 3. 4.]
この章では、数値範囲から配列を作成する方法を説明します。
numpy.arange
この関数は、 ndarray指定された範囲内の等間隔の値を含むオブジェクト。関数の形式は次のとおりです-
numpy.arange(start, stop, step, dtype)
コンストラクターは次のパラメーターを取ります。
シニア番号 | パラメータと説明 |
---|---|
1 | start インターバルの開始。省略した場合、デフォルトは0です。 |
2 | stop 間隔の終わり(この番号は含まれません) |
3 | step 値間の間隔。デフォルトは1です。 |
4 | dtype 結果のndarrayのデータ型。指定しない場合、入力のデータ型が使用されます |
次の例は、この関数の使用方法を示しています。
例1
import numpy as np
x = np.arange(5)
print x
その出力は次のようになります-
[0 1 2 3 4]
例2
import numpy as np
# dtype set
x = np.arange(5, dtype = float)
print x
ここで、出力は次のようになります。
[0. 1. 2. 3. 4.]
例3
# start and stop parameters set
import numpy as np
x = np.arange(10,20,2)
print x
その出力は次のとおりです-
[10 12 14 16 18]
numpy.linspace
この関数はに似ています arange()関数。この関数では、ステップサイズの代わりに、間隔の間に等間隔の値の数が指定されます。この関数の使用法は次のとおりです-
numpy.linspace(start, stop, num, endpoint, retstep, dtype)
コンストラクターは次のパラメーターを取ります。
シニア番号 | パラメータと説明 |
---|---|
1 | start シーケンスの開始値 |
2 | stop エンドポイントがtrueに設定されている場合、シーケンスに含まれるシーケンスの終了値 |
3 | num 生成される等間隔のサンプルの数。デフォルトは50です |
4 | endpoint デフォルトではTrueであるため、停止値がシーケンスに含まれます。falseの場合、含まれません |
5 | retstep trueの場合、サンプルを返し、連続する数値の間を移動します |
6 | dtype 出力のデータ型 ndarray |
次の例は、使用法を示しています linspace 関数。
例1
import numpy as np
x = np.linspace(10,20,5)
print x
その出力は次のようになります-
[10. 12.5 15. 17.5 20.]
例2
# endpoint set to false
import numpy as np
x = np.linspace(10,20, 5, endpoint = False)
print x
出力は次のようになります-
[10. 12. 14. 16. 18.]
例3
# find retstep value
import numpy as np
x = np.linspace(1,2,5, retstep = True)
print x
# retstep here is 0.25
これで、出力は次のようになります。
(array([ 1. , 1.25, 1.5 , 1.75, 2. ]), 0.25)
numpy.logspace
この関数は、 ndarray対数目盛で等間隔に配置された数値を含むオブジェクト。スケールの開始エンドポイントと停止エンドポイントは、ベースのインデックスであり、通常は10です。
numpy.logspace(start, stop, num, endpoint, base, dtype)
次のパラメータは、の出力を決定します logspace 関数。
シニア番号 | パラメータと説明 |
---|---|
1 | start シーケンスの開始点はベーススタートです |
2 | stop シーケンスの最終値はベースストップです |
3 | num 範囲間の値の数。デフォルトは50です |
4 | endpoint trueの場合、stopは範囲内の最後の値です |
5 | base ログスペースの基数、デフォルトは10 |
6 | dtype 出力配列のデータ型。指定しない場合は、他の入力引数に依存します |
次の例は、 logspace 関数。
例1
import numpy as np
# default base is 10
a = np.logspace(1.0, 2.0, num = 10)
print a
その出力は次のようになります-
[ 10. 12.91549665 16.68100537 21.5443469 27.82559402
35.93813664 46.41588834 59.94842503 77.42636827 100. ]
例2
# set base of log space to 2
import numpy as np
a = np.logspace(1,10,num = 10, base = 2)
print a
これで、出力は次のようになります。
[ 2. 4. 8. 16. 32. 64. 128. 256. 512. 1024.]
ndarrayオブジェクトのコンテンツは、Pythonの組み込みコンテナオブジェクトと同じように、インデックス作成またはスライスによってアクセスおよび変更できます。
前述のように、ndarrayオブジェクトのアイテムはゼロベースのインデックスに従います。3種類のインデックス作成方法が利用可能です-field access, basic slicing そして advanced indexing。
基本的なスライスは、Pythonの基本的な概念であるn次元へのスライスの拡張です。Pythonスライスオブジェクトは、start, stop、および step ビルトインへのパラメータ slice関数。このスライスオブジェクトは、配列の一部を抽出するために配列に渡されます。
例1
import numpy as np
a = np.arange(10)
s = slice(2,7,2)
print a[s]
その出力は次のとおりです-
[2 4 6]
上記の例では、 ndarray オブジェクトはによって準備されます arange()関数。次に、スライスオブジェクトは、開始値、停止値、およびステップ値2、7、および2でそれぞれ定義されます。このスライスオブジェクトがndarrayに渡されると、インデックス2から7までのステップ2のオブジェクトの一部がスライスされます。
同じ結果は、コロンで区切られたスライスパラメータ:( start:stop:step)を直接に与えることによっても取得できます。 ndarray オブジェクト。
例2
import numpy as np
a = np.arange(10)
b = a[2:7:2]
print b
ここでは、同じ出力が得られます-
[2 4 6]
パラメータを1つだけ入力すると、インデックスに対応する1つのアイテムが返されます。その前に:を挿入すると、そのインデックス以降のすべてのアイテムが抽出されます。2つのパラメーター(それらの間に:を含む)が使用される場合、デフォルトのステップ1で2つのインデックス(停止インデックスを含まない)の間の項目がスライスされます。
例3
# slice single item
import numpy as np
a = np.arange(10)
b = a[5]
print b
その出力は次のとおりです-
5
例4
# slice items starting from index
import numpy as np
a = np.arange(10)
print a[2:]
これで、出力は次のようになります。
[2 3 4 5 6 7 8 9]
例5
# slice items between indexes
import numpy as np
a = np.arange(10)
print a[2:5]
ここで、出力は次のようになります。
[2 3 4]
上記の説明は多次元に適用されます ndarray あまりにも。
例6
import numpy as np
a = np.array([[1,2,3],[3,4,5],[4,5,6]])
print a
# slice items starting from index
print 'Now we will slice the array from the index a[1:]'
print a[1:]
出力は次のとおりです-
[[1 2 3]
[3 4 5]
[4 5 6]]
Now we will slice the array from the index a[1:]
[[3 4 5]
[4 5 6]]
スライスには省略記号(…)を含めて、配列の次元と同じ長さの選択タプルを作成することもできます。行の位置で省略記号を使用すると、行の項目で構成されるndarrayが返されます。
例7
# array to begin with
import numpy as np
a = np.array([[1,2,3],[3,4,5],[4,5,6]])
print 'Our array is:'
print a
print '\n'
# this returns array of items in the second column
print 'The items in the second column are:'
print a[...,1]
print '\n'
# Now we will slice all items from the second row
print 'The items in the second row are:'
print a[1,...]
print '\n'
# Now we will slice all items from column 1 onwards
print 'The items column 1 onwards are:'
print a[...,1:]
このプログラムの出力は次のとおりです-
Our array is:
[[1 2 3]
[3 4 5]
[4 5 6]]
The items in the second column are:
[2 4 5]
The items in the second row are:
[3 4 5]
The items column 1 onwards are:
[[2 3]
[4 5]
[5 6]]
非タプルシーケンスであるndarray、整数またはブールデータ型のndarrayオブジェクト、または少なくとも1つの項目がシーケンスオブジェクトであるタプルから選択することができます。高度なインデックス作成では、常にデータのコピーが返されます。これに対して、スライスはビューを提示するだけです。
高度なインデックス作成には2つのタイプがあります- Integer そして Boolean。
整数インデックス
このメカニズムは、N次元のインデックスに基づいて配列内の任意のアイテムを選択するのに役立ちます。各整数配列は、その次元へのインデックスの数を表します。インデックスがターゲットのndarrayの次元と同じ数の整数配列で構成されている場合、インデックスは単純になります。
次の例では、ndarrayオブジェクトの各行から指定された列の1つの要素が選択されています。したがって、行インデックスにはすべての行番号が含まれ、列インデックスは選択する要素を指定します。
例1
import numpy as np
x = np.array([[1, 2], [3, 4], [5, 6]])
y = x[[0,1,2], [0,1,0]]
print y
その出力は次のようになります-
[1 4 5]
選択には、最初の配列の(0,0)、(1,1)、および(2,0)の要素が含まれます。
次の例では、4X3配列のコーナーに配置された要素が選択されています。選択の行インデックスは[0、0]と[3,3]ですが、列インデックスは[0,2]と[0,2]です。
例2
import numpy as np
x = np.array([[ 0, 1, 2],[ 3, 4, 5],[ 6, 7, 8],[ 9, 10, 11]])
print 'Our array is:'
print x
print '\n'
rows = np.array([[0,0],[3,3]])
cols = np.array([[0,2],[0,2]])
y = x[rows,cols]
print 'The corner elements of this array are:'
print y
このプログラムの出力は次のとおりです-
Our array is:
[[ 0 1 2]
[ 3 4 5]
[ 6 7 8]
[ 9 10 11]]
The corner elements of this array are:
[[ 0 2]
[ 9 11]]
結果として選択されるのは、コーナー要素を含むndarrayオブジェクトです。
高度なインデックス作成と基本的なインデックス作成は、1つのスライス(:)または省略記号(…)とインデックス配列を使用して組み合わせることができます。次の例では、行にスライスを使用し、列に高度なインデックスを使用しています。スライスを両方に使用した場合の結果は同じです。ただし、高度なインデックスはコピーになり、メモリレイアウトが異なる場合があります。
例3
import numpy as np
x = np.array([[ 0, 1, 2],[ 3, 4, 5],[ 6, 7, 8],[ 9, 10, 11]])
print 'Our array is:'
print x
print '\n'
# slicing
z = x[1:4,1:3]
print 'After slicing, our array becomes:'
print z
print '\n'
# using advanced index for column
y = x[1:4,[1,2]]
print 'Slicing using advanced index for column:'
print y
このプログラムの出力は次のようになります-
Our array is:
[[ 0 1 2]
[ 3 4 5]
[ 6 7 8]
[ 9 10 11]]
After slicing, our array becomes:
[[ 4 5]
[ 7 8]
[10 11]]
Slicing using advanced index for column:
[[ 4 5]
[ 7 8]
[10 11]]
ブール配列インデックス
このタイプの高度なインデックス付けは、結果のオブジェクトが比較演算子などのブール演算の結果であることが意図されている場合に使用されます。
例1
この例では、ブールインデックスの結果として5より大きいアイテムが返されます。
import numpy as np
x = np.array([[ 0, 1, 2],[ 3, 4, 5],[ 6, 7, 8],[ 9, 10, 11]])
print 'Our array is:'
print x
print '\n'
# Now we will print the items greater than 5
print 'The items greater than 5 are:'
print x[x > 5]
このプログラムの出力は次のようになります-
Our array is:
[[ 0 1 2]
[ 3 4 5]
[ 6 7 8]
[ 9 10 11]]
The items greater than 5 are:
[ 6 7 8 9 10 11]
例2
この例では、〜(補数演算子)を使用してNaN(数値ではない)要素を省略しています。
import numpy as np
a = np.array([np.nan, 1,2,np.nan,3,4,5])
print a[~np.isnan(a)]
その出力は次のようになります-
[ 1. 2. 3. 4. 5.]
例3
次の例は、配列から複雑でない要素を除外する方法を示しています。
import numpy as np
a = np.array([1, 2+6j, 5, 3.5+5j])
print a[np.iscomplex(a)]
ここで、出力は次のとおりです。
[2.0+6.j 3.5+5.j]
用語 broadcasting算術演算中にさまざまな形状の配列を処理するNumPyの機能を指します。配列の算術演算は通常、対応する要素に対して実行されます。2つの配列がまったく同じ形状の場合、これらの操作はスムーズに実行されます。
例1
import numpy as np
a = np.array([1,2,3,4])
b = np.array([10,20,30,40])
c = a * b
print c
その出力は次のとおりです-
[10 40 90 160]
2つの配列の次元が異なる場合、要素間の操作はできません。ただし、NumPyでは、ブロードキャスト機能があるため、類似していない形状の配列での操作は引き続き可能です。小さい方の配列はbroadcast 互換性のある形状になるように、より大きな配列のサイズに合わせます。
以下のルールを満たせば放送可能-
小さい配列 ndim 他のものよりもその形に「1」が付加されています。
出力形状の各次元のサイズは、その次元の入力サイズの最大値です。
特定の次元でのサイズが出力サイズと一致するか、値が正確に1である場合、入力を計算に使用できます。
入力のディメンションサイズが1の場合、そのディメンションの最初のデータエントリが、そのディメンションに沿ったすべての計算に使用されます。
配列のセットは broadcastable 上記のルールが有効な結果を生成し、次のいずれかが当てはまる場合-
配列の形状はまったく同じです。
配列の次元数は同じで、各次元の長さは共通の長さまたは1です。
次元が少なすぎる配列は、その形状の前に長さ1の次元を付けることができるため、上記の特性は真です。
次の番組は放送例です。
例2
import numpy as np
a = np.array([[0.0,0.0,0.0],[10.0,10.0,10.0],[20.0,20.0,20.0],[30.0,30.0,30.0]])
b = np.array([1.0,2.0,3.0])
print 'First array:'
print a
print '\n'
print 'Second array:'
print b
print '\n'
print 'First Array + Second Array'
print a + b
このプログラムの出力は次のようになります-
First array:
[[ 0. 0. 0.]
[ 10. 10. 10.]
[ 20. 20. 20.]
[ 30. 30. 30.]]
Second array:
[ 1. 2. 3.]
First Array + Second Array
[[ 1. 2. 3.]
[ 11. 12. 13.]
[ 21. 22. 23.]
[ 31. 32. 33.]]
次の図は、配列がどのようになっているのかを示しています b と互換性を持つように放送されます a。
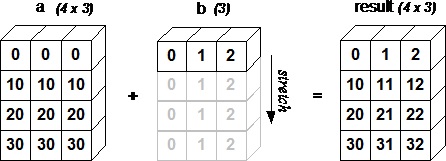
NumPyパッケージにはイテレータオブジェクトが含まれています numpy.nditer。これは効率的な多次元イテレータオブジェクトであり、これを使用して配列を反復処理できます。配列の各要素は、Pythonの標準イテレータインターフェイスを使用してアクセスされます。
arange()関数を使用して3X4配列を作成し、を使用して反復処理してみましょう。 nditer。
例1
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'Original array is:'
print a
print '\n'
print 'Modified array is:'
for x in np.nditer(a):
print x,
このプログラムの出力は次のとおりです-
Original array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Modified array is:
0 5 10 15 20 25 30 35 40 45 50 55
例2
反復の順序は、特定の順序を考慮せずに、配列のメモリレイアウトに一致するように選択されます。これは、上記の配列の転置を繰り返すことで確認できます。
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'Original array is:'
print a
print '\n'
print 'Transpose of the original array is:'
b = a.T
print b
print '\n'
print 'Modified array is:'
for x in np.nditer(b):
print x,
上記のプログラムの出力は次のとおりです。
Original array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Transpose of the original array is:
[[ 0 20 40]
[ 5 25 45]
[10 30 50]
[15 35 55]]
Modified array is:
0 5 10 15 20 25 30 35 40 45 50 55
反復順序
同じ要素がFスタイルの順序を使用して格納されている場合、イテレータは配列を反復するより効率的な方法を選択します。
例1
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'Original array is:'
print a
print '\n'
print 'Transpose of the original array is:'
b = a.T
print b
print '\n'
print 'Sorted in C-style order:'
c = b.copy(order='C')
print c
for x in np.nditer(c):
print x,
print '\n'
print 'Sorted in F-style order:'
c = b.copy(order='F')
print c
for x in np.nditer(c):
print x,
その出力は次のようになります-
Original array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Transpose of the original array is:
[[ 0 20 40]
[ 5 25 45]
[10 30 50]
[15 35 55]]
Sorted in C-style order:
[[ 0 20 40]
[ 5 25 45]
[10 30 50]
[15 35 55]]
0 20 40 5 25 45 10 30 50 15 35 55
Sorted in F-style order:
[[ 0 20 40]
[ 5 25 45]
[10 30 50]
[15 35 55]]
0 5 10 15 20 25 30 35 40 45 50 55
例2
強制することが可能です nditer 明示的に言及することにより、特定の順序を使用することに反対します。
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'Original array is:'
print a
print '\n'
print 'Sorted in C-style order:'
for x in np.nditer(a, order = 'C'):
print x,
print '\n'
print 'Sorted in F-style order:'
for x in np.nditer(a, order = 'F'):
print x,
その出力は次のようになります-
Original array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Sorted in C-style order:
0 5 10 15 20 25 30 35 40 45 50 55
Sorted in F-style order:
0 20 40 5 25 45 10 30 50 15 35 55
配列値の変更
ザ・ nditer オブジェクトには、という別のオプションのパラメータがあります op_flags。デフォルト値は読み取り専用ですが、読み取り/書き込みモードまたは書き込み専用モードに設定できます。これにより、このイテレータを使用して配列要素を変更できるようになります。
例
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'Original array is:'
print a
print '\n'
for x in np.nditer(a, op_flags = ['readwrite']):
x[...] = 2*x
print 'Modified array is:'
print a
その出力は次のとおりです-
Original array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Modified array is:
[[ 0 10 20 30]
[ 40 50 60 70]
[ 80 90 100 110]]
外部ループ
nditerクラスコンストラクタには ‘flags’ 次の値を取ることができるパラメータ-
シニア番号 | パラメータと説明 |
---|---|
1 | c_index C_orderインデックスを追跡できます |
2 | f_index Fortran_orderインデックスが追跡されます |
3 | multi-index 反復ごとに1つのインデックスのタイプを追跡できます |
4 | external_loop 与えられた値が、ゼロ次元配列ではなく、複数の値を持つ1次元配列になるようにします |
例
次の例では、各列に対応する1次元配列がイテレーターによってトラバースされます。
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'Original array is:'
print a
print '\n'
print 'Modified array is:'
for x in np.nditer(a, flags = ['external_loop'], order = 'F'):
print x,
出力は次のとおりです-
Original array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Modified array is:
[ 0 20 40] [ 5 25 45] [10 30 50] [15 35 55]
ブロードキャストの反復
2つのアレイが broadcastable、組み合わせ nditerオブジェクトはそれらを同時に繰り返すことができます。配列がa 次元は3X4で、別の配列があります b 次元1X4の場合、次のタイプのイテレータが使用されます(配列 b のサイズに放送されます a)。
例
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'First array is:'
print a
print '\n'
print 'Second array is:'
b = np.array([1, 2, 3, 4], dtype = int)
print b
print '\n'
print 'Modified array is:'
for x,y in np.nditer([a,b]):
print "%d:%d" % (x,y),
その出力は次のようになります-
First array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Second array is:
[1 2 3 4]
Modified array is:
0:1 5:2 10:3 15:4 20:1 25:2 30:3 35:4 40:1 45:2 50:3 55:4
ndarrayオブジェクトの要素を操作するために、NumPyパッケージでいくつかのルーチンを使用できます。それらは以下のタイプに分類することができます-
形を変える
シニア番号 | 形状と説明 |
---|---|
1 | 形を変える データを変更せずに配列に新しい形状を与えます |
2 | 平らな 配列上の1次元イテレータ |
3 | 平らにする 1次元に折りたたまれた配列のコピーを返します |
4 | ラヴェル 連続するフラット化された配列を返します |
転置演算
シニア番号 | 操作と説明 |
---|---|
1 | 転置 配列の次元を並べ替えます |
2 | ndarray.T self.transpose()と同じ |
3 | ロールアクシス 指定した軸を後方に回転させます |
4 | swapaxes 配列の2つの軸を交換します |
寸法の変更
シニア番号 | 寸法と説明 |
---|---|
1 | 放送 放送を模倣したオブジェクトを生成します |
2 | Broadcast_to 配列を新しい形状にブロードキャストします |
3 | expand_dims 配列の形状を拡張します |
4 | スクイーズ 配列の形状から1次元のエントリを削除します |
配列の結合
シニア番号 | 配列と説明 |
---|---|
1 | 連結する 既存の軸に沿って配列のシーケンスを結合します |
2 | スタック 新しい軸に沿って配列のシーケンスを結合します |
3 | hstack 配列を水平方向に順番にスタックします(列単位) |
4 | vstack 配列を垂直方向(行方向)に順番にスタックします |
配列の分割
シニア番号 | 配列と説明 |
---|---|
1 | スプリット 配列を複数のサブ配列に分割します |
2 | hsplit 配列を複数のサブ配列に水平方向(列方向)に分割します |
3 | vsplit 配列を複数のサブ配列に垂直方向(行方向)に分割します |
要素の追加/削除
シニア番号 | 要素と説明 |
---|---|
1 | サイズ変更 指定された形状の新しい配列を返します |
2 | 追加 配列の最後に値を追加します |
3 | インサート 指定されたインデックスの前に、指定された軸に沿って値を挿入します |
4 | 削除 軸に沿ったサブ配列が削除された新しい配列を返します |
5 | ユニーク 配列の一意の要素を検索します |
以下は、NumPyパッケージで利用可能なビット演算の関数です。
シニア番号 | 操作と説明 |
---|---|
1 | bitwise_and 配列要素のビット単位のAND演算を計算します |
2 | bitwise_or 配列要素のビットごとのOR演算を計算します |
3 | 反転 ビット単位で計算しません |
4 | 左方移動 バイナリ表現のビットを左にシフトします |
5 | right_shift バイナリ表現のビットを右にシフトします |
次の関数は、dtypenumpy.string_またはnumpy.unicode_の配列に対してベクトル化された文字列操作を実行するために使用されます。これらは、Pythonの組み込みライブラリにある標準の文字列関数に基づいています。
シニア番号 | 機能と説明 |
---|---|
1 | 追加() strまたはUnicodeの2つの配列の要素ごとの文字列連結を返します |
2 | かける() 要素ごとに複数の連結を含む文字列を返します |
3 | センター() 指定された長さの文字列の中央に要素がある指定された文字列のコピーを返します |
4 | Capitalize() 最初の文字のみを大文字にした文字列のコピーを返します |
5 | 題名() 文字列またはUnicodeの要素ごとのタイトルケースバージョンを返します |
6 | lower() 要素が小文字に変換された配列を返します |
7 | アッパー() 要素が大文字に変換された配列を返します |
8 | スプリット() セパレータ区切り文字を使用して、文字列内の単語のリストを返します |
9 | splitlines() 要素内の行のリストを返し、行の境界で分割します |
10 | ストリップ() 先頭と末尾の文字が削除されたコピーを返します |
11 | join() シーケンス内の文字列を連結した文字列を返します |
12 | replace() 出現するすべての部分文字列が新しい文字列に置き換えられた文字列のコピーを返します |
13 | decode() str.decodeを要素ごとに呼び出します |
14 | エンコード() str.encodeを要素ごとに呼び出します |
これらの関数は、文字配列クラス(numpy.char)で定義されています。古いNumarrayパッケージには、chararrayクラスが含まれていました。numpy.charクラスの上記の関数は、ベクトル化された文字列操作を実行するのに役立ちます。
当然のことながら、NumPyにはさまざまな数学演算が多数含まれています。NumPyは、標準の三角関数、算術演算、複素数の処理などの関数を提供します。
三角関数
NumPyには、指定された角度の三角関数の比率をラジアンで返す標準の三角関数があります。
Example
import numpy as np
a = np.array([0,30,45,60,90])
print 'Sine of different angles:'
# Convert to radians by multiplying with pi/180
print np.sin(a*np.pi/180)
print '\n'
print 'Cosine values for angles in array:'
print np.cos(a*np.pi/180)
print '\n'
print 'Tangent values for given angles:'
print np.tan(a*np.pi/180)
これがその出力です-
Sine of different angles:
[ 0. 0.5 0.70710678 0.8660254 1. ]
Cosine values for angles in array:
[ 1.00000000e+00 8.66025404e-01 7.07106781e-01 5.00000000e-01
6.12323400e-17]
Tangent values for given angles:
[ 0.00000000e+00 5.77350269e-01 1.00000000e+00 1.73205081e+00
1.63312394e+16]
arcsin, arcos, そして arctan関数は、指定された角度のsin、cos、およびtanの三角関数の逆関数を返します。これらの関数の結果は、次の方法で確認できます。numpy.degrees() function ラジアンを度に変換することによって。
Example
import numpy as np
a = np.array([0,30,45,60,90])
print 'Array containing sine values:'
sin = np.sin(a*np.pi/180)
print sin
print '\n'
print 'Compute sine inverse of angles. Returned values are in radians.'
inv = np.arcsin(sin)
print inv
print '\n'
print 'Check result by converting to degrees:'
print np.degrees(inv)
print '\n'
print 'arccos and arctan functions behave similarly:'
cos = np.cos(a*np.pi/180)
print cos
print '\n'
print 'Inverse of cos:'
inv = np.arccos(cos)
print inv
print '\n'
print 'In degrees:'
print np.degrees(inv)
print '\n'
print 'Tan function:'
tan = np.tan(a*np.pi/180)
print tan
print '\n'
print 'Inverse of tan:'
inv = np.arctan(tan)
print inv
print '\n'
print 'In degrees:'
print np.degrees(inv)
その出力は次のとおりです-
Array containing sine values:
[ 0. 0.5 0.70710678 0.8660254 1. ]
Compute sine inverse of angles. Returned values are in radians.
[ 0. 0.52359878 0.78539816 1.04719755 1.57079633]
Check result by converting to degrees:
[ 0. 30. 45. 60. 90.]
arccos and arctan functions behave similarly:
[ 1.00000000e+00 8.66025404e-01 7.07106781e-01 5.00000000e-01
6.12323400e-17]
Inverse of cos:
[ 0. 0.52359878 0.78539816 1.04719755 1.57079633]
In degrees:
[ 0. 30. 45. 60. 90.]
Tan function:
[ 0.00000000e+00 5.77350269e-01 1.00000000e+00 1.73205081e+00
1.63312394e+16]
Inverse of tan:
[ 0. 0.52359878 0.78539816 1.04719755 1.57079633]
In degrees:
[ 0. 30. 45. 60. 90.]
丸めのための関数
numpy.around()
これは、必要な精度に丸められた値を返す関数です。この関数は次のパラメーターを取ります。
numpy.around(a,decimals)
どこ、
シニア番号 | パラメータと説明 |
---|---|
1 | a 入力データ |
2 | decimals 四捨五入する小数点以下の桁数。デフォルトは0です。負の場合、整数は小数点の左側の位置に丸められます。 |
Example
import numpy as np
a = np.array([1.0,5.55, 123, 0.567, 25.532])
print 'Original array:'
print a
print '\n'
print 'After rounding:'
print np.around(a)
print np.around(a, decimals = 1)
print np.around(a, decimals = -1)
次の出力を生成します-
Original array:
[ 1. 5.55 123. 0.567 25.532]
After rounding:
[ 1. 6. 123. 1. 26. ]
[ 1. 5.6 123. 0.6 25.5]
[ 0. 10. 120. 0. 30. ]
numpy.floor()
この関数は、入力パラメーター以下の最大の整数を返します。の床scalar x は最もおおきい integer i、 そのような i <= x。Pythonでは、フローリングは常に0から丸められることに注意してください。
Example
import numpy as np
a = np.array([-1.7, 1.5, -0.2, 0.6, 10])
print 'The given array:'
print a
print '\n'
print 'The modified array:'
print np.floor(a)
次の出力を生成します-
The given array:
[ -1.7 1.5 -0.2 0.6 10. ]
The modified array:
[ -2. 1. -1. 0. 10.]
numpy.ceil()
ceil()関数は、入力値の上限、つまり、 scalar x 最小です integer i、 そのような i >= x.
Example
import numpy as np
a = np.array([-1.7, 1.5, -0.2, 0.6, 10])
print 'The given array:'
print a
print '\n'
print 'The modified array:'
print np.ceil(a)
次の出力が生成されます-
The given array:
[ -1.7 1.5 -0.2 0.6 10. ]
The modified array:
[ -1. 2. -0. 1. 10.]
add()、subtract()、multiply()、divide()などの算術演算を実行するための入力配列は、同じ形状であるか、配列ブロードキャストルールに準拠している必要があります。
例
import numpy as np
a = np.arange(9, dtype = np.float_).reshape(3,3)
print 'First array:'
print a
print '\n'
print 'Second array:'
b = np.array([10,10,10])
print b
print '\n'
print 'Add the two arrays:'
print np.add(a,b)
print '\n'
print 'Subtract the two arrays:'
print np.subtract(a,b)
print '\n'
print 'Multiply the two arrays:'
print np.multiply(a,b)
print '\n'
print 'Divide the two arrays:'
print np.divide(a,b)
次の出力が生成されます-
First array:
[[ 0. 1. 2.]
[ 3. 4. 5.]
[ 6. 7. 8.]]
Second array:
[10 10 10]
Add the two arrays:
[[ 10. 11. 12.]
[ 13. 14. 15.]
[ 16. 17. 18.]]
Subtract the two arrays:
[[-10. -9. -8.]
[ -7. -6. -5.]
[ -4. -3. -2.]]
Multiply the two arrays:
[[ 0. 10. 20.]
[ 30. 40. 50.]
[ 60. 70. 80.]]
Divide the two arrays:
[[ 0. 0.1 0.2]
[ 0.3 0.4 0.5]
[ 0.6 0.7 0.8]]
NumPyで利用できる他の重要な算術関数のいくつかについて説明しましょう。
numpy.reciprocal()
この関数は、引数の逆数を要素ごとに返します。絶対値が1より大きい要素の場合、Pythonが整数除算を処理する方法のため、結果は常に0になります。整数0の場合、オーバーフロー警告が発行されます。
例
import numpy as np
a = np.array([0.25, 1.33, 1, 0, 100])
print 'Our array is:'
print a
print '\n'
print 'After applying reciprocal function:'
print np.reciprocal(a)
print '\n'
b = np.array([100], dtype = int)
print 'The second array is:'
print b
print '\n'
print 'After applying reciprocal function:'
print np.reciprocal(b)
次の出力が生成されます-
Our array is:
[ 0.25 1.33 1. 0. 100. ]
After applying reciprocal function:
main.py:9: RuntimeWarning: divide by zero encountered in reciprocal
print np.reciprocal(a)
[ 4. 0.7518797 1. inf 0.01 ]
The second array is:
[100]
After applying reciprocal function:
[0]
numpy.power()
この関数は、最初の入力配列の要素をベースとして扱い、2番目の入力配列の対応する要素の累乗で返します。
import numpy as np
a = np.array([10,100,1000])
print 'Our array is:'
print a
print '\n'
print 'Applying power function:'
print np.power(a,2)
print '\n'
print 'Second array:'
b = np.array([1,2,3])
print b
print '\n'
print 'Applying power function again:'
print np.power(a,b)
次の出力が生成されます-
Our array is:
[ 10 100 1000]
Applying power function:
[ 100 10000 1000000]
Second array:
[1 2 3]
Applying power function again:
[ 10 10000 1000000000]
numpy.mod()
この関数は、入力配列内の対応する要素の除算の余りを返します。関数numpy.remainder() 同じ結果が得られます。
import numpy as np
a = np.array([10,20,30])
b = np.array([3,5,7])
print 'First array:'
print a
print '\n'
print 'Second array:'
print b
print '\n'
print 'Applying mod() function:'
print np.mod(a,b)
print '\n'
print 'Applying remainder() function:'
print np.remainder(a,b)
次の出力が生成されます-
First array:
[10 20 30]
Second array:
[3 5 7]
Applying mod() function:
[1 0 2]
Applying remainder() function:
[1 0 2]
次の関数は、複素数の配列に対して操作を実行するために使用されます。
numpy.real() −複素数データ型引数の実数部を返します。
numpy.imag() −複素数データ型引数の虚数部を返します。
numpy.conj() −虚数部の符号を変更して得られる複素共役を返します。
numpy.angle()−複素数の偏角を返します。関数には次数パラメーターがあります。trueの場合、度の角度が返されます。それ以外の場合、角度はラジアンです。
import numpy as np
a = np.array([-5.6j, 0.2j, 11. , 1+1j])
print 'Our array is:'
print a
print '\n'
print 'Applying real() function:'
print np.real(a)
print '\n'
print 'Applying imag() function:'
print np.imag(a)
print '\n'
print 'Applying conj() function:'
print np.conj(a)
print '\n'
print 'Applying angle() function:'
print np.angle(a)
print '\n'
print 'Applying angle() function again (result in degrees)'
print np.angle(a, deg = True)
次の出力が生成されます-
Our array is:
[ 0.-5.6j 0.+0.2j 11.+0.j 1.+1.j ]
Applying real() function:
[ 0. 0. 11. 1.]
Applying imag() function:
[-5.6 0.2 0. 1. ]
Applying conj() function:
[ 0.+5.6j 0.-0.2j 11.-0.j 1.-1.j ]
Applying angle() function:
[-1.57079633 1.57079633 0. 0.78539816]
Applying angle() function again (result in degrees)
[-90. 90. 0. 45.]
NumPyには、配列内の特定の要素から最小、最大、パーセンタイルの標準偏差や分散などを見つけるための有用な統計関数が多数あります。機能は次のように説明されます-
numpy.amin()およびnumpy.amax()
これらの関数は、指定された軸に沿って指定された配列の要素から最小値と最大値を返します。
例
import numpy as np
a = np.array([[3,7,5],[8,4,3],[2,4,9]])
print 'Our array is:'
print a
print '\n'
print 'Applying amin() function:'
print np.amin(a,1)
print '\n'
print 'Applying amin() function again:'
print np.amin(a,0)
print '\n'
print 'Applying amax() function:'
print np.amax(a)
print '\n'
print 'Applying amax() function again:'
print np.amax(a, axis = 0)
次の出力が生成されます-
Our array is:
[[3 7 5]
[8 4 3]
[2 4 9]]
Applying amin() function:
[3 3 2]
Applying amin() function again:
[2 4 3]
Applying amax() function:
9
Applying amax() function again:
[8 7 9]
numpy.ptp()
ザ・ numpy.ptp() 関数は、軸に沿った値の範囲(最大-最小)を返します。
import numpy as np
a = np.array([[3,7,5],[8,4,3],[2,4,9]])
print 'Our array is:'
print a
print '\n'
print 'Applying ptp() function:'
print np.ptp(a)
print '\n'
print 'Applying ptp() function along axis 1:'
print np.ptp(a, axis = 1)
print '\n'
print 'Applying ptp() function along axis 0:'
print np.ptp(a, axis = 0)
次の出力が生成されます-
Our array is:
[[3 7 5]
[8 4 3]
[2 4 9]]
Applying ptp() function:
7
Applying ptp() function along axis 1:
[4 5 7]
Applying ptp() function along axis 0:
[6 3 6]
numpy.percentile()
パーセンタイル(またはパーセンタイル)は、統計で使用される測定値であり、観測値のグループ内の観測値の特定のパーセンテージがそれを下回る値を示します。関数numpy.percentile() 次の引数を取ります。
numpy.percentile(a, q, axis)
どこ、
シニア番号 | 引数と説明 |
---|---|
1 | a 入力配列 |
2 | q 計算するパーセンタイルは0〜100の間でなければなりません |
3 | axis パーセンタイルが計算される軸 |
例
import numpy as np
a = np.array([[30,40,70],[80,20,10],[50,90,60]])
print 'Our array is:'
print a
print '\n'
print 'Applying percentile() function:'
print np.percentile(a,50)
print '\n'
print 'Applying percentile() function along axis 1:'
print np.percentile(a,50, axis = 1)
print '\n'
print 'Applying percentile() function along axis 0:'
print np.percentile(a,50, axis = 0)
次の出力が生成されます-
Our array is:
[[30 40 70]
[80 20 10]
[50 90 60]]
Applying percentile() function:
50.0
Applying percentile() function along axis 1:
[ 40. 20. 60.]
Applying percentile() function along axis 0:
[ 50. 40. 60.]
numpy.median()
Medianデータサンプルの上半分を下半分から分離する値として定義されます。ザ・numpy.median() 関数は、次のプログラムに示すように使用されます。
例
import numpy as np
a = np.array([[30,65,70],[80,95,10],[50,90,60]])
print 'Our array is:'
print a
print '\n'
print 'Applying median() function:'
print np.median(a)
print '\n'
print 'Applying median() function along axis 0:'
print np.median(a, axis = 0)
print '\n'
print 'Applying median() function along axis 1:'
print np.median(a, axis = 1)
次の出力が生成されます-
Our array is:
[[30 65 70]
[80 95 10]
[50 90 60]]
Applying median() function:
65.0
Applying median() function along axis 0:
[ 50. 90. 60.]
Applying median() function along axis 1:
[ 65. 80. 60.]
numpy.mean()
算術平均は、軸に沿った要素の合計を要素の数で割ったものです。ザ・numpy.mean()関数は、配列内の要素の算術平均を返します。軸が言及されている場合、それはそれに沿って計算されます。
例
import numpy as np
a = np.array([[1,2,3],[3,4,5],[4,5,6]])
print 'Our array is:'
print a
print '\n'
print 'Applying mean() function:'
print np.mean(a)
print '\n'
print 'Applying mean() function along axis 0:'
print np.mean(a, axis = 0)
print '\n'
print 'Applying mean() function along axis 1:'
print np.mean(a, axis = 1)
次の出力が生成されます-
Our array is:
[[1 2 3]
[3 4 5]
[4 5 6]]
Applying mean() function:
3.66666666667
Applying mean() function along axis 0:
[ 2.66666667 3.66666667 4.66666667]
Applying mean() function along axis 1:
[ 2. 4. 5.]
numpy.average()
加重平均は、各コンポーネントにその重要性を反映する係数を掛けた結果の平均です。ザ・numpy.average()関数は、別の配列で指定されたそれぞれの重みに従って、配列内の要素の加重平均を計算します。関数は軸パラメータを持つことができます。軸が指定されていない場合、配列はフラット化されます。
配列[1,2,3,4]と対応する重み[4,3,2,1]を考慮して、加重平均は、対応する要素の積を加算し、その合計を重みの合計で割ることによって計算されます。
加重平均=(1 * 4 + 2 * 3 + 3 * 2 + 4 * 1)/(4 + 3 + 2 + 1)
例
import numpy as np
a = np.array([1,2,3,4])
print 'Our array is:'
print a
print '\n'
print 'Applying average() function:'
print np.average(a)
print '\n'
# this is same as mean when weight is not specified
wts = np.array([4,3,2,1])
print 'Applying average() function again:'
print np.average(a,weights = wts)
print '\n'
# Returns the sum of weights, if the returned parameter is set to True.
print 'Sum of weights'
print np.average([1,2,3, 4],weights = [4,3,2,1], returned = True)
次の出力が生成されます-
Our array is:
[1 2 3 4]
Applying average() function:
2.5
Applying average() function again:
2.0
Sum of weights
(2.0, 10.0)
多次元配列では、計算軸を指定できます。
例
import numpy as np
a = np.arange(6).reshape(3,2)
print 'Our array is:'
print a
print '\n'
print 'Modified array:'
wt = np.array([3,5])
print np.average(a, axis = 1, weights = wt)
print '\n'
print 'Modified array:'
print np.average(a, axis = 1, weights = wt, returned = True)
次の出力が生成されます-
Our array is:
[[0 1]
[2 3]
[4 5]]
Modified array:
[ 0.625 2.625 4.625]
Modified array:
(array([ 0.625, 2.625, 4.625]), array([ 8., 8., 8.]))
標準偏差
標準偏差は、平均からの偏差の2乗の平均の平方根です。標準偏差の式は次のとおりです。
std = sqrt(mean(abs(x - x.mean())**2))
配列が[1、2、3、4]の場合、その平均は2.5です。したがって、偏差の2乗は[2.25、0.25、0.25、2.25]であり、その平均の平方根を4で割った値、つまりsqrt(5/4)は1.1180339887498949です。
例
import numpy as np
print np.std([1,2,3,4])
次の出力が生成されます-
1.1180339887498949
分散
分散は、偏差の2乗の平均です。 mean(abs(x - x.mean())**2)。言い換えると、標準偏差は分散の平方根です。
例
import numpy as np
print np.var([1,2,3,4])
次の出力が生成されます-
1.25
NumPyでは、さまざまな並べ替え関連の機能を利用できます。これらの並べ替え関数は、さまざまな並べ替えアルゴリズムを実装します。各並べ替えアルゴリズムは、実行速度、最悪の場合のパフォーマンス、必要なワークスペース、およびアルゴリズムの安定性によって特徴付けられます。次の表は、3つのソートアルゴリズムの比較を示しています。
種類 | 速度 | 最悪の場合 | 作業スペース | 安定 |
---|---|---|---|---|
「クイックソート」 | 1 | O(n ^ 2) | 0 | 番号 |
「マージソート」 | 2 | O(n * log(n)) | 〜n / 2 | はい |
「ヒープソート」 | 3 | O(n * log(n)) | 0 | 番号 |
numpy.sort()
sort()関数は、入力配列のソートされたコピーを返します。次のパラメータがあります-
numpy.sort(a, axis, kind, order)
どこ、
シニア番号 | パラメータと説明 |
---|---|
1 | a ソートする配列 |
2 | axis 配列がソートされる軸。ない場合、配列はフラット化され、最後の軸で並べ替えられます |
3 | kind デフォルトはクイックソートです |
4 | order 配列にフィールドが含まれている場合、ソートされるフィールドの順序 |
例
import numpy as np
a = np.array([[3,7],[9,1]])
print 'Our array is:'
print a
print '\n'
print 'Applying sort() function:'
print np.sort(a)
print '\n'
print 'Sort along axis 0:'
print np.sort(a, axis = 0)
print '\n'
# Order parameter in sort function
dt = np.dtype([('name', 'S10'),('age', int)])
a = np.array([("raju",21),("anil",25),("ravi", 17), ("amar",27)], dtype = dt)
print 'Our array is:'
print a
print '\n'
print 'Order by name:'
print np.sort(a, order = 'name')
次の出力が生成されます-
Our array is:
[[3 7]
[9 1]]
Applying sort() function:
[[3 7]
[1 9]]
Sort along axis 0:
[[3 1]
[9 7]]
Our array is:
[('raju', 21) ('anil', 25) ('ravi', 17) ('amar', 27)]
Order by name:
[('amar', 27) ('anil', 25) ('raju', 21) ('ravi', 17)]
numpy.argsort()
ザ・ numpy.argsort()関数は、指定された軸に沿って、指定された種類の並べ替えを使用して入力配列に対して間接的な並べ替えを実行し、データのインデックスの配列を返します。このインデックス配列は、並べ替えられた配列を作成するために使用されます。
例
import numpy as np
x = np.array([3, 1, 2])
print 'Our array is:'
print x
print '\n'
print 'Applying argsort() to x:'
y = np.argsort(x)
print y
print '\n'
print 'Reconstruct original array in sorted order:'
print x[y]
print '\n'
print 'Reconstruct the original array using loop:'
for i in y:
print x[i],
次の出力が生成されます-
Our array is:
[3 1 2]
Applying argsort() to x:
[1 2 0]
Reconstruct original array in sorted order:
[1 2 3]
Reconstruct the original array using loop:
1 2 3
numpy.lexsort()
関数は、一連のキーを使用して間接ソートを実行します。キーは、スプレッドシートの列として表示できます。この関数は、ソートされたデータを取得できるインデックスの配列を返します。最後のキーがたまたまソートの主キーであることに注意してください。
例
import numpy as np
nm = ('raju','anil','ravi','amar')
dv = ('f.y.', 's.y.', 's.y.', 'f.y.')
ind = np.lexsort((dv,nm))
print 'Applying lexsort() function:'
print ind
print '\n'
print 'Use this index to get sorted data:'
print [nm[i] + ", " + dv[i] for i in ind]
次の出力が生成されます-
Applying lexsort() function:
[3 1 0 2]
Use this index to get sorted data:
['amar, f.y.', 'anil, s.y.', 'raju, f.y.', 'ravi, s.y.']
NumPyモジュールには、配列内を検索するための多くの関数があります。最大値、最小値、および特定の条件を満たす要素を見つけるための関数を使用できます。
numpy.argmax()およびnumpy.argmin()
これらの2つの関数は、指定された軸に沿ってそれぞれ最大要素と最小要素のインデックスを返します。
例
import numpy as np
a = np.array([[30,40,70],[80,20,10],[50,90,60]])
print 'Our array is:'
print a
print '\n'
print 'Applying argmax() function:'
print np.argmax(a)
print '\n'
print 'Index of maximum number in flattened array'
print a.flatten()
print '\n'
print 'Array containing indices of maximum along axis 0:'
maxindex = np.argmax(a, axis = 0)
print maxindex
print '\n'
print 'Array containing indices of maximum along axis 1:'
maxindex = np.argmax(a, axis = 1)
print maxindex
print '\n'
print 'Applying argmin() function:'
minindex = np.argmin(a)
print minindex
print '\n'
print 'Flattened array:'
print a.flatten()[minindex]
print '\n'
print 'Flattened array along axis 0:'
minindex = np.argmin(a, axis = 0)
print minindex
print '\n'
print 'Flattened array along axis 1:'
minindex = np.argmin(a, axis = 1)
print minindex
次の出力が生成されます-
Our array is:
[[30 40 70]
[80 20 10]
[50 90 60]]
Applying argmax() function:
7
Index of maximum number in flattened array
[30 40 70 80 20 10 50 90 60]
Array containing indices of maximum along axis 0:
[1 2 0]
Array containing indices of maximum along axis 1:
[2 0 1]
Applying argmin() function:
5
Flattened array:
10
Flattened array along axis 0:
[0 1 1]
Flattened array along axis 1:
[0 2 0]
numpy.nonzero()
ザ・ numpy.nonzero() 関数は、入力配列内のゼロ以外の要素のインデックスを返します。
例
import numpy as np
a = np.array([[30,40,0],[0,20,10],[50,0,60]])
print 'Our array is:'
print a
print '\n'
print 'Applying nonzero() function:'
print np.nonzero (a)
次の出力が生成されます-
Our array is:
[[30 40 0]
[ 0 20 10]
[50 0 60]]
Applying nonzero() function:
(array([0, 0, 1, 1, 2, 2]), array([0, 1, 1, 2, 0, 2]))
numpy.where()
where()関数は、指定された条件が満たされる入力配列内の要素のインデックスを返します。
例
import numpy as np
x = np.arange(9.).reshape(3, 3)
print 'Our array is:'
print x
print 'Indices of elements > 3'
y = np.where(x > 3)
print y
print 'Use these indices to get elements satisfying the condition'
print x[y]
次の出力が生成されます-
Our array is:
[[ 0. 1. 2.]
[ 3. 4. 5.]
[ 6. 7. 8.]]
Indices of elements > 3
(array([1, 1, 2, 2, 2]), array([1, 2, 0, 1, 2]))
Use these indices to get elements satisfying the condition
[ 4. 5. 6. 7. 8.]
numpy.extract()
ザ・ extract() 関数は、任意の条件を満たす要素を返します。
import numpy as np
x = np.arange(9.).reshape(3, 3)
print 'Our array is:'
print x
# define a condition
condition = np.mod(x,2) == 0
print 'Element-wise value of condition'
print condition
print 'Extract elements using condition'
print np.extract(condition, x)
次の出力が生成されます-
Our array is:
[[ 0. 1. 2.]
[ 3. 4. 5.]
[ 6. 7. 8.]]
Element-wise value of condition
[[ True False True]
[False True False]
[ True False True]]
Extract elements using condition
[ 0. 2. 4. 6. 8.]
コンピュータのメモリに保存されるデータは、CPUが使用するアーキテクチャに依存することがわかりました。リトルエンディアン(最下位は最小アドレスに格納されます)またはビッグエンディアン(最下位バイトは最小アドレスに格納されます)の場合があります。
numpy.ndarray.byteswap()
ザ・ numpy.ndarray.byteswap() 関数は、ビッグエンディアンとリトルエンディアンの2つの表現を切り替えます。
import numpy as np
a = np.array([1, 256, 8755], dtype = np.int16)
print 'Our array is:'
print a
print 'Representation of data in memory in hexadecimal form:'
print map(hex,a)
# byteswap() function swaps in place by passing True parameter
print 'Applying byteswap() function:'
print a.byteswap(True)
print 'In hexadecimal form:'
print map(hex,a)
# We can see the bytes being swapped
次の出力が生成されます-
Our array is:
[1 256 8755]
Representation of data in memory in hexadecimal form:
['0x1', '0x100', '0x2233']
Applying byteswap() function:
[256 1 13090]
In hexadecimal form:
['0x100', '0x1', '0x3322']
関数の実行中に、入力配列のコピーを返すものもあれば、ビューを返すものもあります。コンテンツが物理的に別の場所に保存されている場合、それは呼び出されますCopy。一方、同じメモリコンテンツの異なるビューが提供される場合、それを次のように呼びます。View。
コピーなし
単純な割り当てでは、配列オブジェクトのコピーは作成されません。代わりに、元の配列と同じid()を使用してアクセスします。ザ・id() Cのポインタと同様に、Pythonオブジェクトのユニバーサル識別子を返します。
さらに、どちらかの変更はもう一方にも反映されます。たとえば、一方の形状を変更すると、もう一方の形状も変更されます。
例
import numpy as np
a = np.arange(6)
print 'Our array is:'
print a
print 'Applying id() function:'
print id(a)
print 'a is assigned to b:'
b = a
print b
print 'b has same id():'
print id(b)
print 'Change shape of b:'
b.shape = 3,2
print b
print 'Shape of a also gets changed:'
print a
次の出力が生成されます-
Our array is:
[0 1 2 3 4 5]
Applying id() function:
139747815479536
a is assigned to b:
[0 1 2 3 4 5]
b has same id():
139747815479536
Change shape of b:
[[0 1]
[2 3]
[4 5]]
Shape of a also gets changed:
[[0 1]
[2 3]
[4 5]]
表示または浅いコピー
NumPyは ndarray.view()元の配列と同じデータを調べる新しい配列オブジェクトであるメソッド。以前の場合とは異なり、新しい配列の次元を変更しても、元の配列の次元は変更されません。
例
import numpy as np
# To begin with, a is 3X2 array
a = np.arange(6).reshape(3,2)
print 'Array a:'
print a
print 'Create view of a:'
b = a.view()
print b
print 'id() for both the arrays are different:'
print 'id() of a:'
print id(a)
print 'id() of b:'
print id(b)
# Change the shape of b. It does not change the shape of a
b.shape = 2,3
print 'Shape of b:'
print b
print 'Shape of a:'
print a
次の出力が生成されます-
Array a:
[[0 1]
[2 3]
[4 5]]
Create view of a:
[[0 1]
[2 3]
[4 5]]
id() for both the arrays are different:
id() of a:
140424307227264
id() of b:
140424151696288
Shape of b:
[[0 1 2]
[3 4 5]]
Shape of a:
[[0 1]
[2 3]
[4 5]]
配列のスライスはビューを作成します。
例
import numpy as np
a = np.array([[10,10], [2,3], [4,5]])
print 'Our array is:'
print a
print 'Create a slice:'
s = a[:, :2]
print s
次の出力が生成されます-
Our array is:
[[10 10]
[ 2 3]
[ 4 5]]
Create a slice:
[[10 10]
[ 2 3]
[ 4 5]]
ディープコピー
ザ・ ndarray.copy()関数はディープコピーを作成します。これはアレイとそのデータの完全なコピーであり、元のアレイとは共有されません。
例
import numpy as np
a = np.array([[10,10], [2,3], [4,5]])
print 'Array a is:'
print a
print 'Create a deep copy of a:'
b = a.copy()
print 'Array b is:'
print b
#b does not share any memory of a
print 'Can we write b is a'
print b is a
print 'Change the contents of b:'
b[0,0] = 100
print 'Modified array b:'
print b
print 'a remains unchanged:'
print a
次の出力が生成されます-
Array a is:
[[10 10]
[ 2 3]
[ 4 5]]
Create a deep copy of a:
Array b is:
[[10 10]
[ 2 3]
[ 4 5]]
Can we write b is a
False
Change the contents of b:
Modified array b:
[[100 10]
[ 2 3]
[ 4 5]]
a remains unchanged:
[[10 10]
[ 2 3]
[ 4 5]]
NumPyパッケージにはMatrixライブラリが含まれています numpy.matlib。このモジュールには、ndarrayオブジェクトの代わりに行列を返す関数があります。
matlib.empty()
ザ・ matlib.empty()関数は、エントリを初期化せずに新しい行列を返します。この関数は次のパラメーターを取ります。
numpy.matlib.empty(shape, dtype, order)
どこ、
シニア番号 | パラメータと説明 |
---|---|
1 | shape int またはのタプル int 新しいマトリックスの形状を定義する |
2 | Dtype オプション。出力のデータ型 |
3 | order CまたはF |
例
import numpy.matlib
import numpy as np
print np.matlib.empty((2,2))
# filled with random data
次の出力が生成されます-
[[ 2.12199579e-314, 4.24399158e-314]
[ 4.24399158e-314, 2.12199579e-314]]
numpy.matlib.zeros()
この関数は、ゼロで満たされた行列を返します。
import numpy.matlib
import numpy as np
print np.matlib.zeros((2,2))
次の出力が生成されます-
[[ 0. 0.]
[ 0. 0.]]
numpy.matlib.ones()
この関数は、1で満たされた行列を返します。
import numpy.matlib
import numpy as np
print np.matlib.ones((2,2))
次の出力が生成されます-
[[ 1. 1.]
[ 1. 1.]]
numpy.matlib.eye()
この関数は、対角要素に沿って1、その他の場所にゼロを持つ行列を返します。この関数は次のパラメーターを取ります。
numpy.matlib.eye(n, M,k, dtype)
どこ、
シニア番号 | パラメータと説明 |
---|---|
1 | n 結果の行列の行数 |
2 | M 列の数。デフォルトはnです。 |
3 | k 対角線のインデックス |
4 | dtype 出力のデータ型 |
例
import numpy.matlib
import numpy as np
print np.matlib.eye(n = 3, M = 4, k = 0, dtype = float)
次の出力が生成されます-
[[ 1. 0. 0. 0.]
[ 0. 1. 0. 0.]
[ 0. 0. 1. 0.]]
numpy.matlib.identity()
ザ・ numpy.matlib.identity()関数は、指定されたサイズの単位行列を返します。単位行列は、すべての対角要素が1である正方行列です。
import numpy.matlib
import numpy as np
print np.matlib.identity(5, dtype = float)
次の出力が生成されます-
[[ 1. 0. 0. 0. 0.]
[ 0. 1. 0. 0. 0.]
[ 0. 0. 1. 0. 0.]
[ 0. 0. 0. 1. 0.]
[ 0. 0. 0. 0. 1.]]
numpy.matlib.rand()
ザ・ numpy.matlib.rand() 関数は、ランダムな値で満たされた指定されたサイズの行列を返します。
例
import numpy.matlib
import numpy as np
print np.matlib.rand(3,3)
次の出力が生成されます-
[[ 0.82674464 0.57206837 0.15497519]
[ 0.33857374 0.35742401 0.90895076]
[ 0.03968467 0.13962089 0.39665201]]
Note行列は常に2次元ですが、ndarrayはn次元の配列です。両方のオブジェクトは相互変換可能です。
例
import numpy.matlib
import numpy as np
i = np.matrix('1,2;3,4')
print i
次の出力が生成されます-
[[1 2]
[3 4]]
例
import numpy.matlib
import numpy as np
j = np.asarray(i)
print j
次の出力が生成されます-
[[1 2]
[3 4]]
例
import numpy.matlib
import numpy as np
k = np.asmatrix (j)
print k
次の出力が生成されます-
[[1 2]
[3 4]]
NumPyパッケージには numpy.linalg線形代数に必要なすべての機能を提供するモジュール。このモジュールの重要な機能のいくつかを次の表に示します。
シニア番号 | 機能と説明 |
---|---|
1 | ドット 2つの配列の内積 |
2 | vdot 2つのベクトルの内積 |
3 | 内側 2つの配列の内積 |
4 | matmul 2つの配列の行列積 |
5 | 行列式 配列の行列式を計算します |
6 | 解決する 線形行列方程式を解きます |
7 | inv 行列の逆行列を見つけます |
Matplotlibは、Python用のプロットライブラリです。これは、NumPyと一緒に使用され、MatLabの効果的なオープンソースの代替となる環境を提供します。また、PyQtやwxPythonなどのグラフィックツールキットでも使用できます。
Matplotlibモジュールは、John D.Hunterによって最初に作成されました。2012年以来、MichaelDroettboomが主要な開発者です。現在、Matplotlibver。1.5.1は利用可能な安定バージョンです。このパッケージは、バイナリ配布およびwww.matplotlib.orgのソースコード形式で入手できます。
従来、パッケージは次のステートメントを追加することでPythonスクリプトにインポートされます-
from matplotlib import pyplot as plt
ここに pyplot()2Dデータをプロットするために使用されるmatplotlibライブラリで最も重要な関数です。次のスクリプトは方程式をプロットしますy = 2x + 5
例
import numpy as np
from matplotlib import pyplot as plt
x = np.arange(1,11)
y = 2 * x + 5
plt.title("Matplotlib demo")
plt.xlabel("x axis caption")
plt.ylabel("y axis caption")
plt.plot(x,y)
plt.show()
ndarrayオブジェクトxはから作成されます np.arange() function の値として x axis。の対応する値y axis 別の場所に保管されています ndarray object y。これらの値は、plot() matplotlibパッケージのpyplotサブモジュールの機能。
グラフィック表現はによって表示されます show() 関数。
上記のコードは次の出力を生成するはずです-

線形グラフの代わりに、フォーマット文字列をに追加することにより、値を個別に表示できます。 plot()関数。以下のフォーマット文字を使用できます。
シニア番号 | キャラクターと説明 |
---|---|
1 | '-' 実線スタイル |
2 | '--' 破線のスタイル |
3 | '-.' 一点鎖線スタイル |
4 | ':' 点線スタイル |
5 | '.' ポイントマーカー |
6 | ',' ピクセルマーカー |
7 | 'o' サークルマーカー |
8 | 'v' Triangle_downマーカー |
9 | '^' Triangle_upマーカー |
10 | '<' Triangle_leftマーカー |
11 | '>' Triangle_rightマーカー |
12 | '1' Tri_downマーカー |
13 | '2' Tri_upマーカー |
14 | '3' Tri_leftマーカー |
15 | '4' Tri_rightマーカー |
16 | 's' 正方形のマーカー |
17 | 'p' ペンタゴンマーカー |
18 | '*' スターマーカー |
19 | 'h' Hexagon1マーカー |
20 | 'H' Hexagon2マーカー |
21 | '+' プラスマーカー |
22 | 'x' Xマーカー |
23 | 'D' ダイヤモンドマーカー |
24 | 'd' Thin_diamondマーカー |
25 | '|' Vlineマーカー |
26 | '_' Hlineマーカー |
次の色の略語も定義されています。
キャラクター | 色 |
---|---|
'b' | 青い |
'g' | 緑 |
'r' | 赤 |
'c' | シアン |
'm' | 赤紫色 |
'y' | 黄 |
「k」 | ブラック |
「w」 | 白い |
上記の例の線の代わりに、点を表す円を表示するには、 “ob” plot()関数のフォーマット文字列として。
例
import numpy as np
from matplotlib import pyplot as plt
x = np.arange(1,11)
y = 2 * x + 5
plt.title("Matplotlib demo")
plt.xlabel("x axis caption")
plt.ylabel("y axis caption")
plt.plot(x,y,"ob")
plt.show()
上記のコードは次の出力を生成するはずです-
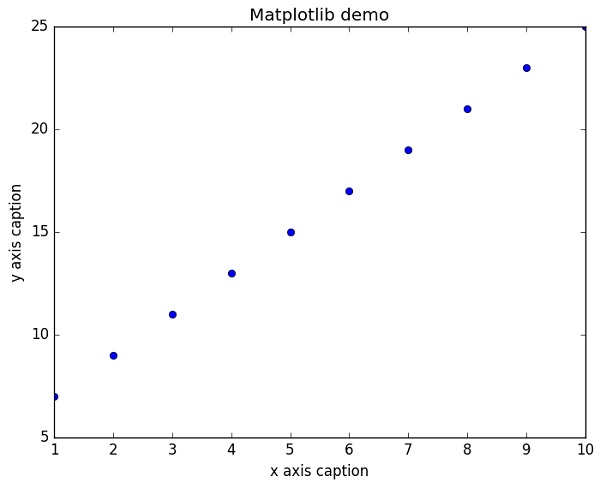
正弦波プロット
次のスクリプトは、 sine wave plot matplotlibを使用します。
例
import numpy as np
import matplotlib.pyplot as plt
# Compute the x and y coordinates for points on a sine curve
x = np.arange(0, 3 * np.pi, 0.1)
y = np.sin(x)
plt.title("sine wave form")
# Plot the points using matplotlib
plt.plot(x, y)
plt.show()
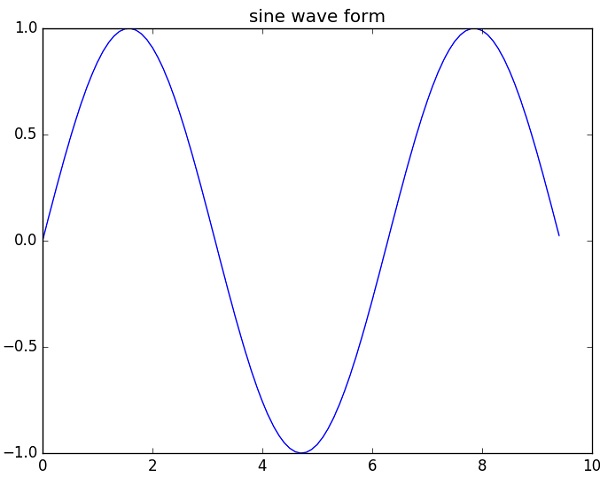
subplot()
subplot()関数を使用すると、同じ図にさまざまなものをプロットできます。次のスクリプトでは、sine そして cosine values プロットされます。
例
import numpy as np
import matplotlib.pyplot as plt
# Compute the x and y coordinates for points on sine and cosine curves
x = np.arange(0, 3 * np.pi, 0.1)
y_sin = np.sin(x)
y_cos = np.cos(x)
# Set up a subplot grid that has height 2 and width 1,
# and set the first such subplot as active.
plt.subplot(2, 1, 1)
# Make the first plot
plt.plot(x, y_sin)
plt.title('Sine')
# Set the second subplot as active, and make the second plot.
plt.subplot(2, 1, 2)
plt.plot(x, y_cos)
plt.title('Cosine')
# Show the figure.
plt.show()
上記のコードは次の出力を生成するはずです-

バー()
ザ・ pyplot submodule 提供します bar()棒グラフを生成する関数。次の例では、2セットの棒グラフを作成します。x そして y 配列。
例
from matplotlib import pyplot as plt
x = [5,8,10]
y = [12,16,6]
x2 = [6,9,11]
y2 = [6,15,7]
plt.bar(x, y, align = 'center')
plt.bar(x2, y2, color = 'g', align = 'center')
plt.title('Bar graph')
plt.ylabel('Y axis')
plt.xlabel('X axis')
plt.show()
このコードは次の出力を生成するはずです-
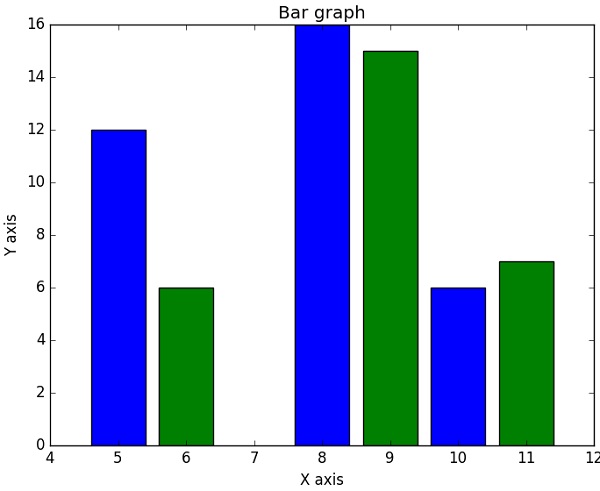
NumPyには numpy.histogram()データの度数分布をグラフで表した関数。と呼ばれるクラス間隔に対応する等しい水平サイズの長方形bin そして variable height 周波数に対応します。
numpy.histogram()
numpy.histogram()関数は、入力配列とビンを2つのパラメーターとして受け取ります。ビン配列の連続する要素は、各ビンの境界として機能します。
import numpy as np
a = np.array([22,87,5,43,56,73,55,54,11,20,51,5,79,31,27])
np.histogram(a,bins = [0,20,40,60,80,100])
hist,bins = np.histogram(a,bins = [0,20,40,60,80,100])
print hist
print bins
次の出力が生成されます-
[3 4 5 2 1]
[0 20 40 60 80 100]
plt()
Matplotlibは、このヒストグラムの数値表現をグラフに変換できます。ザ・plt() function of pyplotサブモジュールは、データとbin配列を含む配列をパラメーターとして受け取り、ヒストグラムに変換します。
from matplotlib import pyplot as plt
import numpy as np
a = np.array([22,87,5,43,56,73,55,54,11,20,51,5,79,31,27])
plt.hist(a, bins = [0,20,40,60,80,100])
plt.title("histogram")
plt.show()
次の出力が生成されます-
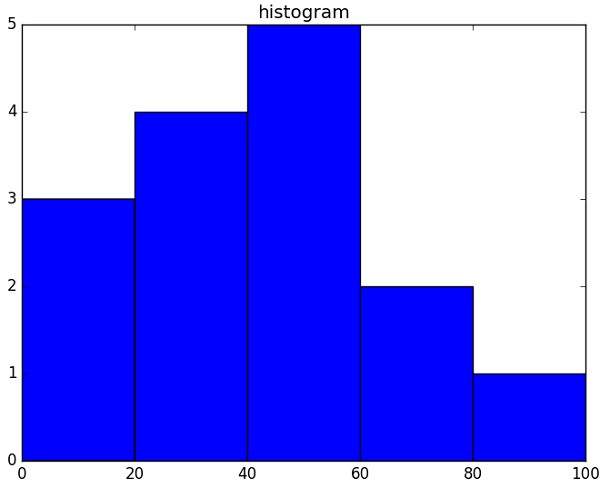
ndarrayオブジェクトは、ディスクファイルに保存したりディスクファイルからロードしたりできます。使用可能なIO関数は次のとおりです。
load() そして save() 関数は/ numPyバイナリファイルを処理します( npy 拡張)
loadtxt() そして savetxt() 関数は通常のテキストファイルを処理します
NumPyは、ndarrayオブジェクトの単純なファイル形式を導入しています。この.npy ファイルは、ndarrayを再構築するために必要なデータ、形状、dtype、およびその他の情報をディスクファイルに格納し、ファイルが異なるアーキテクチャの別のマシン上にある場合でも、配列が正しく取得されるようにします。
numpy.save()
ザ・ numpy.save() fileは、入力配列をディスクファイルに格納します。 npy 拡張。
import numpy as np
a = np.array([1,2,3,4,5])
np.save('outfile',a)
から配列を再構築するには outfile.npy、 使用する load() 関数。
import numpy as np
b = np.load('outfile.npy')
print b
次の出力が生成されます-
array([1, 2, 3, 4, 5])
save()関数とload()関数は、追加のブールパラメーターを受け入れます allow_pickles。Pythonのpickleは、ディスクファイルに保存したり、ディスクファイルから読み取ったりする前に、オブジェクトをシリアル化および逆シリアル化するために使用されます。
savetxt()
単純なテキストファイル形式での配列データの保存と取得は、 savetxt() そして loadtxt() 関数。
例
import numpy as np
a = np.array([1,2,3,4,5])
np.savetxt('out.txt',a)
b = np.loadtxt('out.txt')
print b
次の出力が生成されます-
[ 1. 2. 3. 4. 5.]
savetxt()およびloadtxt()関数は、ヘッダー、フッター、区切り文字などの追加のオプションパラメーターを受け入れます。