Spring MVC - Ví dụ về Tải lên Tệp
Ví dụ sau cho thấy cách sử dụng Kiểm soát Tải lên Tệp trong các biểu mẫu sử dụng khuôn khổ Spring Web MVC. Để bắt đầu, hãy để chúng tôi có một IDE Eclipse đang hoạt động tại chỗ và tuân thủ các bước sau để phát triển Ứng dụng web dựa trên Biểu mẫu động bằng cách sử dụng Spring Web Framework.
Bươc | Sự miêu tả |
---|---|
1 | Tạo một dự án với tên HelloWeb dưới một gói com.tutorialspoint như được giải thích trong chương Spring MVC - Hello World. |
2 | Tạo các lớp Java FileModel, FileUploadController trong gói com.tutorialspoint. |
3 | Tạo tập tin dạng xem fileUpload.jsp, success.jsp trong thư mục con jsp. |
4 | Tạo một thư mục temp trong thư mục con WebContent. |
5 | Tải xuống thư viện Apache Commons FileUpload commons-fileupload.jar và thư viện Apache Commons IO commons-io.jar . Đặt chúng vào CLASSPATH của bạn. |
6 | Bước cuối cùng là tạo nội dung của tệp nguồn và cấu hình và xuất ứng dụng như được giải thích bên dưới. |
FileModel.java
package com.tutorialspoint;
import org.springframework.web.multipart.MultipartFile;
public class FileModel {
private MultipartFile file;
public MultipartFile getFile() {
return file;
}
public void setFile(MultipartFile file) {
this.file = file;
}
}
FileUploadController.java
package com.tutorialspoint;
import java.io.File;
import java.io.IOException;
import javax.servlet.ServletContext;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.util.FileCopyUtils;
import org.springframework.validation.BindingResult;
import org.springframework.validation.annotation.Validated;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.servlet.ModelAndView;
@Controller
public class FileUploadController {
@Autowired
ServletContext context;
@RequestMapping(value = "/fileUploadPage", method = RequestMethod.GET)
public ModelAndView fileUploadPage() {
FileModel file = new FileModel();
ModelAndView modelAndView = new ModelAndView("fileUpload", "command", file);
return modelAndView;
}
@RequestMapping(value="/fileUploadPage", method = RequestMethod.POST)
public String fileUpload(@Validated FileModel file, BindingResult result, ModelMap model) throws IOException {
if (result.hasErrors()) {
System.out.println("validation errors");
return "fileUploadPage";
} else {
System.out.println("Fetching file");
MultipartFile multipartFile = file.getFile();
String uploadPath = context.getRealPath("") + File.separator + "temp" + File.separator;
//Now do something with file...
FileCopyUtils.copy(file.getFile().getBytes(), new File(uploadPath+file.getFile().getOriginalFilename()));
String fileName = multipartFile.getOriginalFilename();
model.addAttribute("fileName", fileName);
return "success";
}
}
}
HelloWeb-servlet.xml
<beans xmlns = "http://www.springframework.org/schema/beans"
xmlns:context = "http://www.springframework.org/schema/context"
xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation = "
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd">
<context:component-scan base-package = "com.tutorialspoint" />
<bean class = "org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name = "prefix" value = "/WEB-INF/jsp/" />
<property name = "suffix" value = ".jsp" />
</bean>
<bean id = "multipartResolver"
class = "org.springframework.web.multipart.commons.CommonsMultipartResolver" />
</beans>
Đây, cho phương thức dịch vụ đầu tiên fileUploadPage(), chúng tôi đã vượt qua một khoảng trống FileModelđối tượng trong đối tượng ModelAndView có tên "lệnh", vì khuôn khổ mùa xuân mong đợi một đối tượng có tên "lệnh", nếu bạn đang sử dụng thẻ <form: form> trong tệp JSP của mình. Vì vậy, khifileUploadPage() phương thức được gọi, nó trả về fileUpload.jsp lượt xem.
Phương thức dịch vụ thứ hai fileUpload() sẽ được gọi đối với một phương thức POST trên HelloWeb/fileUploadPageURL. Bạn sẽ chuẩn bị tệp để tải lên dựa trên thông tin đã gửi. Cuối cùng, chế độ xem "thành công" sẽ được trả về từ phương thức dịch vụ, điều này sẽ dẫn đến kết xuất thành công.jsp.
fileUpload.jsp
<%@ page contentType="text/html; charset = UTF-8" %>
<%@ taglib prefix = "form" uri = "http://www.springframework.org/tags/form"%>
<html>
<head>
<title>File Upload Example</title>
</head>
<body>
<form:form method = "POST" modelAttribute = "fileUpload"
enctype = "multipart/form-data">
Please select a file to upload :
<input type = "file" name = "file" />
<input type = "submit" value = "upload" />
</form:form>
</body>
</html>
Ở đây, chúng tôi đang sử dụng modelAttribute thuộc tính value = "fileUpload" để ánh xạ kiểm soát Tải lên tệp với mô hình máy chủ.
thành công.jsp
<%@ page contentType = "text/html; charset = UTF-8" %>
<html>
<head>
<title>File Upload Example</title>
</head>
<body>
FileName :
lt;b> ${fileName} </b> - Uploaded Successfully.
</body>
</html>
Khi bạn đã hoàn tất việc tạo tệp nguồn và cấu hình, hãy xuất ứng dụng của bạn. Nhấp chuột phải vào ứng dụng của bạn, sử dụngExport → WAR File và lưu tệp HelloWeb.war trong thư mục ứng dụng web của Tomcat.
Bây giờ, hãy khởi động máy chủ Tomcat của bạn và đảm bảo rằng bạn có thể truy cập các trang web khác từ thư mục ứng dụng web bằng trình duyệt chuẩn. Hãy thử một URL–http://localhost:8080/HelloWeb/fileUploadPage và chúng ta sẽ thấy màn hình sau, nếu mọi thứ đều ổn với Ứng dụng Web Mùa xuân.
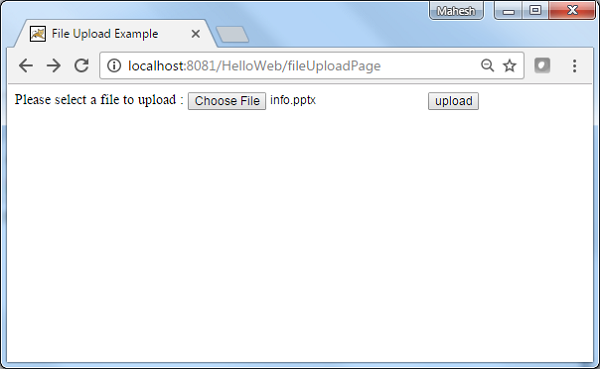
Sau khi gửi các thông tin cần thiết, hãy nhấp vào nút gửi để gửi biểu mẫu. Bạn sẽ thấy màn hình sau, nếu mọi thứ đều ổn với Ứng dụng Web Mùa xuân.
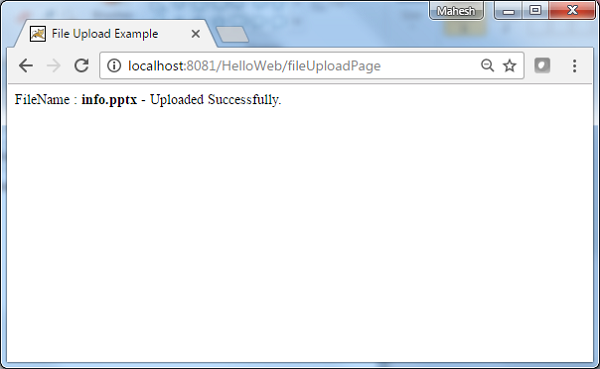