MFC-リンクリスト
A linked listは線形データ構造であり、各要素は個別のオブジェクトです。リストの各要素(これをノードと呼びます)は、データと次のノードへの参照の2つの項目で構成されます。最後のノードにはnullへの参照があります。
リンクリストは、シーケンスを一緒に表すノードのグループで構成されるデータ構造です。これは、プログラマーが必要なときにいつでもデータを格納するための新しい場所を自動的に作成できるように、構造を使用してデータを格納する方法です。その顕著な特徴のいくつかは次のとおりです。
リンクリストは、アイテムを含む一連のリンクです。
各リンクには、別のリンクへの接続が含まれています。
リスト内の各項目はノードと呼ばれます。
リストに少なくとも1つのノードが含まれている場合、新しいノードがリストの最後の要素として配置されます。
リストにノードが1つしかない場合、そのノードは最初と最後のアイテムを表します。
リンクリストには2つのタイプがあります-
単一リンクリスト
単一リンクリストは、データ構造の一種です。単一リンクリストでは、リスト内の各ノードは、ノードの内容と、リスト内の次のノードへのポインタまたは参照を格納します。

二重リンクリスト
二重リンクリストは、ノードと呼ばれる順次リンクされたレコードのセットで構成されるリンクデータ構造です。各ノードには、ノードのシーケンス内の前のノードと次のノードへの参照である2つのフィールドが含まれています。
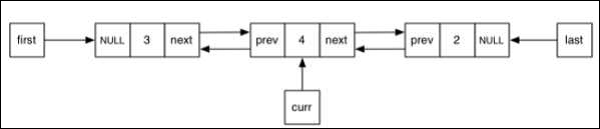
CListクラス
MFCはクラスを提供します CListこれはテンプレートのリンクリストの実装であり、完全に機能します。CListリストは、二重リンクリストのように動作します。タイプPOSITIONの変数は、リストのキーです。POSITION変数をイテレーターとして使用してリストを順番にトラバースしたり、ブックマークとして場所を保持したりできます。
シニア番号 | 名前と説明 |
---|---|
1 | AddHead 要素(または別のリスト内のすべての要素)をリストの先頭に追加します(新しい先頭を作成します)。 |
2 | AddTail 要素(または別のリスト内のすべての要素)をリストの末尾に追加します(新しい末尾を作成します)。 |
3 | Find ポインタ値で指定された要素の位置を取得します。 |
4 | FindIndex ゼロベースのインデックスで指定された要素の位置を取得します。 |
5 | GetAt 指定された位置にある要素を取得します。 |
6 | GetCount このリストの要素の数を返します。 |
7 | GetHead リストのhead要素を返します(空にすることはできません)。 |
8 | GetHeadPosition リストのhead要素の位置を返します。 |
9 | GetNext 反復する次の要素を取得します。 |
10 | GetPrev 反復する前の要素を取得します。 |
11 | GetSize このリストの要素の数を返します。 |
12 | GetTail リストの末尾要素を返します(空にすることはできません)。 |
13 | GetTailPosition リストの末尾要素の位置を返します。 |
14 | InsertAfter 指定された位置の後に新しい要素を挿入します。 |
15 | InsertBefore 指定された位置の前に新しい要素を挿入します。 |
16 | IsEmpty 空のリスト条件(要素なし)をテストします。 |
17 | RemoveAll このリストからすべての要素を削除します。 |
18 | RemoveAt 位置で指定された要素をこのリストから削除します。 |
19 | RemoveHead リストの先頭から要素を削除します。 |
20 | RemoveTail リストの末尾から要素を削除します。 |
21 | SetAt 要素を特定の位置に設定します。 |
以下は、CListオブジェクトに対するさまざまな操作です-
CListオブジェクトを作成する
CList値またはオブジェクトのコレクションを作成するには、最初にコレクションの値のタイプを決定する必要があります。次のコードに示すように、int、CString、doubleなどの既存のプリミティブデータ型の1つを使用できます。
CList<double, double>m_list;
アイテムを追加する
アイテムを追加するには、CList :: AddTail()関数を使用できます。リストの最後に項目を追加します。リストの先頭に要素を追加するには、CList :: AddHead()関数を使用できます。OnInitDialog()CListで、オブジェクトが作成され、次のコードに示すように4つの値が追加されます。
CList<double, double>m_list;
//Add items to the list
m_list.AddTail(100.75);
m_list.AddTail(85.26);
m_list.AddTail(95.78);
m_list.AddTail(90.1);
アイテムを取得する
タイプPOSITIONの変数は、リストのキーです。POSITION変数をイテレーターとして使用して、リストを順番にトラバースできます。
Step 1 −リストから要素を取得するには、次のコードを使用してすべての値を取得します。
//iterate the list
POSITION pos = m_list.GetHeadPosition();
while (pos) {
double nData = m_list.GetNext(pos);
CString strVal;
strVal.Format(L"%.2f\n", nData);
m_strText.Append(strVal);
}
Step 2 −これが完全なCMFCCListDemoDlg :: OnInitDialog()関数です。
BOOL CMFCCListDemoDlg::OnInitDialog() {
CDialogEx::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
CList<double, double>m_list;
//Add items to the list
m_list.AddTail(100.75);
m_list.AddTail(85.26);
m_list.AddTail(95.78);
m_list.AddTail(90.1);
//iterate the list
POSITION pos = m_list.GetHeadPosition();
while (pos) {
double nData = m_list.GetNext(pos);
CString strVal;
strVal.Format(L"%.f\n", nData);
m_strText.Append(strVal);
}
UpdateData(FALSE);
return TRUE; // return TRUE unless you set the focus to a control
}
Step 3 −上記のコードをコンパイルして実行すると、次の出力が表示されます。

途中でアイテムを追加
リストの中央にアイテムを追加するには、CList ::。InsertAfter()関数とCList ::。InsertBefore()関数を使用できます。2つのパラメータが必要です。1つは位置(追加できる場所)、2つ目は値です。
Step 1 −次のコードに示すように、新しいアイテムを挿入しましょう。
BOOL CMFCCListDemoDlg::OnInitDialog() {
CDialogEx::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
CList<double, double>m_list;
//Add items to the list
m_list.AddTail(100.75);
m_list.AddTail(85.26);
m_list.AddTail(95.78);
m_list.AddTail(90.1);
POSITION position = m_list.Find(85.26);
m_list.InsertBefore(position, 200.0);
m_list.InsertAfter(position, 300.0);
//iterate the list
POSITION pos = m_list.GetHeadPosition();
while (pos) {
double nData = m_list.GetNext(pos);
CString strVal;
strVal.Format(L"%.2f\n", nData);
m_strText.Append(strVal);
}
UpdateData(FALSE);
return TRUE; // return TRUE unless you set the focus to a control
}
Step 2 −これで、最初に値85.26の位置を取得し、次にその値の前後に1つの要素を挿入したことがわかります。
Step 3 −上記のコードをコンパイルして実行すると、次の出力が表示されます。

アイテム値の更新
配列の途中でアイテムを更新するには、CArray ::。SetAt()関数を使用できます。それには2つのパラメータが必要です。1つは位置、2つ目は値です。
次のコードに示すように、リストの300.00を400に更新しましょう。
BOOL CMFCCListDemoDlg::OnInitDialog() {
CDialogEx::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
CList<double, double>m_list;
//Add items to the list
m_list.AddTail(100.75);
m_list.AddTail(85.26);
m_list.AddTail(95.78);
m_list.AddTail(90.1);
POSITION position = m_list.Find(85.26);
m_list.InsertBefore(position, 200.0);
m_list.InsertAfter(position, 300.0);
position = m_list.Find(300.00);
m_list.SetAt(position, 400.00);
//iterate the list
POSITION pos = m_list.GetHeadPosition();
while (pos) {
double nData = m_list.GetNext(pos);
CString strVal;
strVal.Format(L"%.2f\n", nData);
m_strText.Append(strVal);
}
UpdateData(FALSE);
return TRUE; // return TRUE unless you set the focus to a control
}
上記のコードをコンパイルして実行すると、次の出力が表示されます。これで、300.00の値が400.00に更新されたことがわかります。

アイテムを削除する
特定のアイテムを削除するには、CList :: RemoveAt()関数を使用できます。リストからすべての要素を削除するには、CList :: RemoveAll()関数を使用できます。
値として95.78を持つ要素を削除しましょう。
BOOL CMFCCListDemoDlg::OnInitDialog() {
CDialogEx::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
CList<double, double>m_list;
//Add items to the list
m_list.AddTail(100.75);
m_list.AddTail(85.26);
m_list.AddTail(95.78);
m_list.AddTail(90.1);
POSITION position = m_list.Find(85.26);
m_list.InsertBefore(position, 200.0);
m_list.InsertAfter(position, 300.0);
position = m_list.Find(300.00);
m_list.SetAt(position, 400.00);
position = m_list.Find(95.78);
m_list.RemoveAt(position);
//iterate the list
POSITION pos = m_list.GetHeadPosition();
while (pos) {
double nData = m_list.GetNext(pos);
CString strVal;
strVal.Format(L"%.2f\n", nData);
m_strText.Append(strVal);
}
UpdateData(FALSE);
return TRUE; // return TRUE unless you set the focus to a control
}
上記のコードをコンパイルして実行すると、次の出力が表示されます。これで、95.78の値がリストの一部ではなくなったことがわかります。
