ReactJS-Fluxの使用
この章では、Reactアプリケーションでフラックスパターンを実装する方法を学習します。我々は使用するだろうReduxフレームワーク。この章の目的は、接続に必要なすべての部品の最も簡単な例を示すことです。Redux そして React。
ステップ1-Reduxをインストールする
Reduxを経由してインストールします command prompt 窓。
C:\Users\username\Desktop\reactApp>npm install --save react-redux
ステップ2-ファイルとフォルダを作成する
このステップでは、フォルダとファイルを作成します actions、 reducers、および components。作業が完了すると、フォルダー構造は次のようになります。
C:\Users\Tutorialspoint\Desktop\reactApp>mkdir actions
C:\Users\Tutorialspoint\Desktop\reactApp>mkdir components
C:\Users\Tutorialspoint\Desktop\reactApp>mkdir reducers
C:\Users\Tutorialspoint\Desktop\reactApp>type nul > actions/actions.js
C:\Users\Tutorialspoint\Desktop\reactApp>type nul > reducers/reducers.js
C:\Users\Tutorialspoint\Desktop\reactApp>type nul > components/AddTodo.js
C:\Users\Tutorialspoint\Desktop\reactApp>type nul > components/Todo.js
C:\Users\Tutorialspoint\Desktop\reactApp>type nul > components/TodoList.js

ステップ3-アクション
アクションは、を使用するJavaScriptオブジェクトです。 typeストアに送信する必要があるデータについて通知するプロパティ。私たちは定義していますADD_TODOリストに新しいアイテムを追加するために使用されるアクション。ザ・addTodo 関数は、アクションを返し、を設定するアクションクリエーターです。 id 作成されたすべてのアイテムに対して。
アクション/actions.js
export const ADD_TODO = 'ADD_TODO'
let nextTodoId = 0;
export function addTodo(text) {
return {
type: ADD_TODO,
id: nextTodoId++,
text
};
}
ステップ4-レデューサー
アクションはアプリの変更をトリガーするだけですが、 reducersそれらの変更を指定します。使用していますswitch 検索するステートメント ADD_TODOアクション。レデューサーは、2つのパラメーターをとる関数です(state そして action)更新された状態を計算して返します。
最初の関数は新しいアイテムを作成するために使用され、2番目の関数はそのアイテムをリストにプッシュします。終わりに向かって、私たちは使用していますcombineReducers 将来使用する可能性のある新しいレデューサーを追加できるヘルパー関数。
reducers / reducers.js
import { combineReducers } from 'redux'
import { ADD_TODO } from '../actions/actions'
function todo(state, action) {
switch (action.type) {
case ADD_TODO:
return {
id: action.id,
text: action.text,
}
default:
return state
}
}
function todos(state = [], action) {
switch (action.type) {
case ADD_TODO:
return [
...state,
todo(undefined, action)
]
default:
return state
}
}
const todoApp = combineReducers({
todos
})
export default todoApp
ステップ5-保存
ストアは、アプリの状態を保持する場所です。レデューサーがあれば、ストアを作成するのは非常に簡単です。店舗の物件をprovider ルートコンポーネントをラップする要素。
main.js
import React from 'react'
import { render } from 'react-dom'
import { createStore } from 'redux'
import { Provider } from 'react-redux'
import App from './App.jsx'
import todoApp from './reducers/reducers'
let store = createStore(todoApp)
let rootElement = document.getElementById('app')
render(
<Provider store = {store}>
<App />
</Provider>,
rootElement
)
ステップ6-ルートコンポーネント
ザ・ Appコンポーネントは、アプリのルートコンポーネントです。ルートコンポーネントのみがreduxを認識している必要があります。注意すべき重要な部分はconnect ルートコンポーネントを接続するために使用される関数 App に store。
この関数はかかります select引数として機能します。Select関数はストアから状態を取得し、小道具を返します(visibleTodos)コンポーネントで使用できます。
App.jsx
import React, { Component } from 'react'
import { connect } from 'react-redux'
import { addTodo } from './actions/actions'
import AddTodo from './components/AddTodo.js'
import TodoList from './components/TodoList.js'
class App extends Component {
render() {
const { dispatch, visibleTodos } = this.props
return (
<div>
<AddTodo onAddClick = {text =>dispatch(addTodo(text))} />
<TodoList todos = {visibleTodos}/>
</div>
)
}
}
function select(state) {
return {
visibleTodos: state.todos
}
}
export default connect(select)(App);
ステップ7-その他のコンポーネント
これらのコンポーネントはreduxを認識すべきではありません。
components / AddTodo.js
import React, { Component, PropTypes } from 'react'
export default class AddTodo extends Component {
render() {
return (
<div>
<input type = 'text' ref = 'input' />
<button onClick = {(e) => this.handleClick(e)}>
Add
</button>
</div>
)
}
handleClick(e) {
const node = this.refs.input
const text = node.value.trim()
this.props.onAddClick(text)
node.value = ''
}
}
components / Todo.js
import React, { Component, PropTypes } from 'react'
export default class Todo extends Component {
render() {
return (
<li>
{this.props.text}
</li>
)
}
}
components / TodoList.js
import React, { Component, PropTypes } from 'react'
import Todo from './Todo.js'
export default class TodoList extends Component {
render() {
return (
<ul>
{this.props.todos.map(todo =>
<Todo
key = {todo.id}
{...todo}
/>
)}
</ul>
)
}
}
アプリを起動すると、リストにアイテムを追加できるようになります。
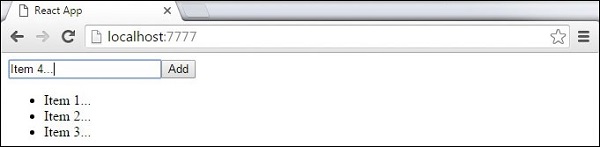