GWT-이벤트 처리
GWT는 Java AWT 또는 SWING 사용자 인터페이스 프레임 워크와 유사한 이벤트 핸들러 모델을 제공합니다.
리스너 인터페이스는 위젯이 이벤트를 알리기 위해 호출하는 하나 이상의 메소드를 정의합니다. GWT는 가능한 다양한 이벤트에 해당하는 인터페이스 목록을 제공합니다.
특정 유형의 이벤트를 수신하려는 클래스는 연관된 핸들러 인터페이스를 구현 한 다음 위젯에 대한 참조를 전달하여 이벤트 세트를 구독합니다.
예를 들어 Button 수업 게시 click events따라서 ClickHandler 를 구현하는 클래스를 작성하여click 행사.
이벤트 핸들러 인터페이스
모든 GWT 이벤트 핸들러는 EventHandler 인터페이스 에서 확장되었으며 각 핸들러에는 단일 인수가있는 단일 메소드 만 있습니다. 이 인수는 항상 연관된 이벤트 유형의 객체입니다. 마다event개체에는 전달 된 이벤트 개체를 조작하는 여러 메서드가 있습니다. 예를 들어 클릭 이벤트의 경우 다음과 같이 핸들러를 작성해야합니다.
/**
* create a custom click handler which will call
* onClick method when button is clicked.
*/
public class MyClickHandler implements ClickHandler {
@Override
public void onClick(ClickEvent event) {
Window.alert("Hello World!");
}
}
이제 클릭 이벤트를 수신하려는 모든 클래스는 addClickHandler() 다음과 같이 이벤트 핸들러를 등록하려면-
/**
* create button and attach click handler
*/
Button button = new Button("Click Me!");
button.addClickHandler(new MyClickHandler());
이벤트 유형을 지원하는 각 위젯에는 HandlerRegistration add 형식의 메소드가 있습니다.Foo매니저(Foo이벤트) 어디서 Foo Click, Error, KeyPress 등과 같은 실제 이벤트입니다.
다음은 중요한 GWT 이벤트 핸들러 및 관련 이벤트 및 핸들러 등록 방법의 목록입니다.
Sr. 아니. | 이벤트 인터페이스 | 이벤트 방법 및 설명 |
---|---|---|
1 | 선택 전 핸들러 <I> | void on Before Selection (Before Selection Event<I> event); BeforeSelectionEvent가 시작될 때 호출됩니다. |
2 | BlurHandler | void on Blur(Blur Event event); Blur Event가 발생하면 호출됩니다. |
삼 | ChangeHandler | void on Change(ChangeEvent event); 변경 이벤트가 발생하면 호출됩니다. |
4 | ClickHandler | void on Click(ClickEvent event); 네이티브 클릭 이벤트가 시작되면 호출됩니다. |
5 | CloseHandler <T> | void on Close(CloseEvent<T> event); CloseEvent가 시작되면 호출됩니다. |
6 | 상황에 맞는 메뉴 처리기 | void on Context Menu(Context Menu Event event); 네이티브 컨텍스트 메뉴 이벤트가 시작될 때 호출됩니다. |
7 | 더블 클릭 핸들러 | void on Double Click(Double Click Event event); 더블 클릭 이벤트가 발생하면 호출됩니다. |
8 | 오류 처리기 | void on Error(Error Event event); 오류 이벤트가 발생하면 호출됩니다. |
9 | 포커스 핸들러 | void on Focus(Focus Event event); Focus Event가 발생하면 호출됩니다. |
10 | Form Panel.Submit Complete Handler | void on Submit Complete(Form Panel.Submit Complete Event event); 양식이 성공적으로 제출되면 시작됩니다. |
11 | FormPanel.SubmitHandler | void on Submit(Form Panel.Submit Event event); 양식이 제출되면 시작됩니다. |
12 | 키 다운 핸들러 | void on Key Down(Key Down Event event); KeyDownEvent가 시작될 때 호출됩니다. |
13 | KeyPressHandler | void on KeyPress(KeyPressEvent event); KeyPressEvent가 시작될 때 호출됩니다. |
14 | KeyUpHandler | void on KeyUp(KeyUpEvent event); KeyUpEvent가 시작될 때 호출됩니다. |
15 | LoadHandler | void on Load(LoadEvent event); LoadEvent가 시작될 때 호출됩니다. |
16 | MouseDownHandler | void on MouseDown(MouseDownEvent event); MouseDown이 실행될 때 호출됩니다. |
17 | MouseMoveHandler | void on MouseMove(MouseMoveEvent event); MouseMoveEvent가 시작될 때 호출됩니다. |
18 | MouseOutHandler | void on MouseOut(MouseOutEvent event); MouseOutEvent가 시작될 때 호출됩니다. |
19 | MouseOverHandler | void on MouseOver(MouseOverEvent event); MouseOverEvent가 시작되면 호출됩니다. |
20 | MouseUpHandler | void on MouseUp(MouseUpEvent event); MouseUpEvent가 시작되면 호출됩니다. |
21 | MouseWheelHandler | void on MouseWheel(MouseWheelEvent event); MouseWheelEvent가 시작되면 호출됩니다. |
22 | ResizeHandler | void on Resize(ResizeEvent event); 위젯 크기가 조정되면 시작됩니다. |
23 | ScrollHandler | void on Scroll(ScrollEvent event); ScrollEvent가 시작될 때 호출됩니다. |
24 | SelectionHandler <I> | void on Selection(SelectionEvent<I> event); SelectionEvent가 시작될 때 호출됩니다. |
25 | ValueChangeHandler <I> | void on ValueChange(ValueChangeEvent<I> event); ValueChangeEvent가 시작되면 호출됩니다. |
26 | Window.ClosingHandler | void on WindowClosing(Window.ClosingEvent event); 브라우저 창이 닫히거나 다른 사이트로 이동하기 직전에 시작됩니다. |
27 | Window.ScrollHandler | void on WindowScroll(Window.ScrollEvent event); 브라우저 창이 스크롤되면 시작됩니다. |
이벤트 방법
앞서 언급했듯이 각 핸들러에는 이벤트 객체를 보유하는 단일 인수가있는 단일 메서드가 있습니다 ( 예 : void onClick (ClickEvent event) 또는 void onKeyDown (KeyDownEvent event)) . 이 행사는 같은 객체 ClickEvent 및 KeyDownEvent이 아래에 나열되어있는 몇 가지 일반적인 방법이있다 -
Sr. 아니. | 방법 및 설명 |
---|---|
1 | protected void dispatch(ClickHandler handler) 이 메서드는 HandlerManager에 의해서만 호출되어야합니다. |
2 | DomEvent.Type <FooHandler> getAssociatedType() 이 메소드는 등록에 사용 된 유형을 반환합니다. Foo 행사. |
삼 | static DomEvent.Type<FooHandler> getType() 이 메소드는 다음과 관련된 이벤트 유형을 가져옵니다. Foo 이벤트. |
4 | public java.lang.Object getSource() 이 메서드는이 이벤트를 마지막으로 시작한 소스를 반환합니다. |
5 | protected final boolean isLive() 이 메서드는 이벤트가 라이브인지 여부를 반환합니다. |
6 | protected void kill() 이 메소드는 이벤트를 종료합니다. |
예
이 예는 간단한 단계를 통해 Click 이벤트 및 KeyDownGWT에서 이벤트 처리. 다음 단계에 따라 GWT에서 생성 한 GWT 애플리케이션을 업데이트합니다 -애플리케이션 생성 장-
단계 | 기술 |
---|---|
1 | GWT- 애플리케이션 만들기 장에 설명 된대로 com.tutorialspoint 패키지 아래에 HelloWorld 라는 이름으로 프로젝트를 만듭니다 . |
2 | 아래 설명과 같이 HelloWorld.gwt.xml , HelloWorld.css , HelloWorld.html 및 HelloWorld.java 를 수정하십시오 . 나머지 파일은 변경하지 마십시오. |
삼 | 애플리케이션을 컴파일하고 실행하여 구현 된 논리의 결과를 확인합니다. |
다음은 수정 된 모듈 설명 자의 내용입니다. src/com.tutorialspoint/HelloWorld.gwt.xml.
<?xml version = "1.0" encoding = "UTF-8"?>
<module rename-to = 'helloworld'>
<!-- Inherit the core Web Toolkit stuff. -->
<inherits name = 'com.google.gwt.user.User'/>
<!-- Inherit the default GWT style sheet. -->
<inherits name = 'com.google.gwt.user.theme.clean.Clean'/>
<!-- Specify the app entry point class. -->
<entry-point class = 'com.tutorialspoint.client.HelloWorld'/>
<!-- Specify the paths for translatable code -->
<source path = 'client'/>
<source path = 'shared'/>
</module>
다음은 수정 된 스타일 시트 파일의 내용입니다. war/HelloWorld.css.
body {
text-align: center;
font-family: verdana, sans-serif;
}
h1 {
font-size: 2em;
font-weight: bold;
color: #777777;
margin: 40px 0px 70px;
text-align: center;
}
다음은 수정 된 HTML 호스트 파일의 내용입니다. war/HelloWorld.html.
<html>
<head>
<title>Hello World</title>
<link rel = "stylesheet" href = "HelloWorld.css"/>
<script language = "javascript" src = "helloworld/helloworld.nocache.js">
</script>
</head>
<body>
<h1>Event Handling Demonstration</h1>
<div id = "gwtContainer"></div>
</body>
</html>
Java 파일의 다음 내용을 갖도록합시다 src/com.tutorialspoint/HelloWorld.java GWT에서 이벤트 처리를 사용하는 방법을 보여줍니다.
package com.tutorialspoint.client;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.event.dom.client.ClickEvent;
import com.google.gwt.event.dom.client.ClickHandler;
import com.google.gwt.event.dom.client.KeyCodes;
import com.google.gwt.event.dom.client.KeyDownEvent;
import com.google.gwt.event.dom.client.KeyDownHandler;
import com.google.gwt.user.client.Window;
import com.google.gwt.user.client.ui.Button;
import com.google.gwt.user.client.ui.DecoratorPanel;
import com.google.gwt.user.client.ui.HasHorizontalAlignment;
import com.google.gwt.user.client.ui.RootPanel;
import com.google.gwt.user.client.ui.TextBox;
import com.google.gwt.user.client.ui.VerticalPanel;
public class HelloWorld implements EntryPoint {
public void onModuleLoad() {
/**
* create textbox and attach key down handler
*/
TextBox textBox = new TextBox();
textBox.addKeyDownHandler(new MyKeyDownHandler());
/*
* create button and attach click handler
*/
Button button = new Button("Click Me!");
button.addClickHandler(new MyClickHandler());
VerticalPanel panel = new VerticalPanel();
panel.setSpacing(10);
panel.setHorizontalAlignment(HasHorizontalAlignment.ALIGN_CENTER);
panel.setSize("300", "100");
panel.add(textBox);
panel.add(button);
DecoratorPanel decoratorPanel = new DecoratorPanel();
decoratorPanel.add(panel);
RootPanel.get("gwtContainer").add(decoratorPanel);
}
/**
* create a custom click handler which will call
* onClick method when button is clicked.
*/
private class MyClickHandler implements ClickHandler {
@Override
public void onClick(ClickEvent event) {
Window.alert("Hello World!");
}
}
/**
* create a custom key down handler which will call
* onKeyDown method when a key is down in textbox.
*/
private class MyKeyDownHandler implements KeyDownHandler {
@Override
public void onKeyDown(KeyDownEvent event) {
if(event.getNativeKeyCode() == KeyCodes.KEY_ENTER){
Window.alert(((TextBox)event.getSource()).getValue());
}
}
}
}
모든 변경이 완료되면 GWT-Create Application 장 에서했던 것처럼 개발 모드에서 애플리케이션을 컴파일하고 실행하겠습니다 . 응용 프로그램에 문제가 없으면 다음과 같은 결과가 생성됩니다.
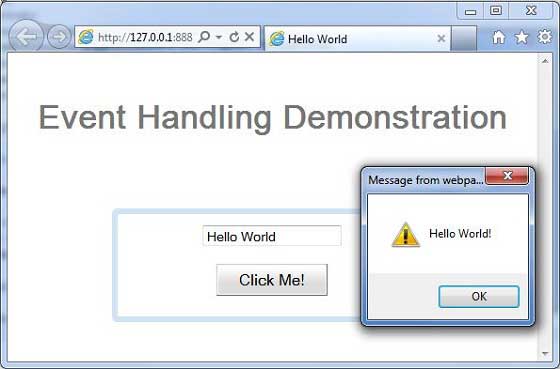