ApacheTapestry-組み込みコンポーネント
この章では、Tapestryに組み込まれているコンポーネントについて適切な例を挙げて説明します。Tapestryは、65を超える組み込みコンポーネントをサポートしています。カスタムコンポーネントを作成することもできます。注目すべきコンポーネントのいくつかを詳しく説明しましょう。
コンポーネントの場合
ifコンポーネントは、条件付きでブロックをレンダリングするために使用されます。条件はテストパラメータによってチェックされます。
ページを作成 IfSample.java 以下に示すように-
package com.example.MyFirstApplication.pages;
public class Ifsample {
public String getUser() {
return "user1";
}
}
次に、対応するテンプレートファイルを次のように作成します-
<html t:type = "newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<h3>If-else component example </h3>
<t:if test = "user">
Hello ${user}
<p:else>
<h4> You are not a Tapestry user </h4>
</p:else>
</t:if>
</html>
ページをリクエストすると、次のような結果が表示されます。
Result − http:// localhost:8080 / MyFirstApplication / ifsample
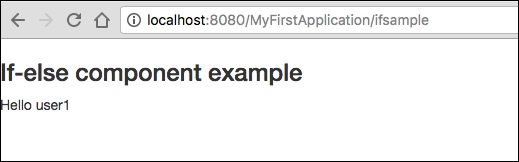
コンポーネントを委任しない限り
ザ・ unless component上で説明したifコンポーネントの正反対です。一方、delegate component単独ではレンダリングを行いません。代わりに、通常はマークアップをブロック要素に委任します。コンポーネントがデリゲートとブロックを使用して動的コンテンツを条件付きで交換できる場合を除きます。
ページを作成 Unless.java 次のように。
package com.example.MyFirstApplication.pages;
import org.apache.tapestry5.Block;
import org.apache.tapestry5.annotations.Property;
import org.apache.tapestry5.ioc.annotations.Inject;
import org.apache.tapestry5.PersistenceConstants;
import org.apache.tapestry5.annotations.Persist;
public class Unless {
@Property
@Persist(PersistenceConstants.FLASH)
private String value;
@Property
private Boolean bool;
@Inject
Block t, f, n;
public Block getCase() {
if (bool == Boolean.TRUE ) {
return t;
} else {
return f;
}
}
}
次に、対応するテンプレートファイルを次のように作成します-
<html t:type = "newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<h4> Delegate component </h4>
<div class = "div1">
<t:delegate to = "case"/>
</div>
<h4> If-Unless component </h4>
<div class = "div1">
<t:if test = "bool">
<t:delegate to = "block:t"/>
</t:if>
<t:unless test = "bool">
<t:delegate to = "block:notT"/>
</t:unless>
</div>
<t:block id = "t">
bool == Boolean.TRUE.
</t:block>
<t:block id = "notT">
bool = Boolean.FALSE.
</t:block>
<t:block id = "f">
bool == Boolean.FALSE.
</t:block>
</html>
ページをリクエストすると、次のような結果が表示されます。
Result − http:// localhost:8080 / MyFirstApplication / unless

ループコンポーネント
ループコンポーネントは、コレクションアイテムをループし、すべての値/反復の本体をレンダリングするための基本コンポーネントです。
以下に示すようにループページを作成します-
Loop.java
package com.example.MyFirstApplication.pages;
import org.apache.tapestry5.annotations.Property;
public class Loop {
@Property
private int i;
}
次に、対応するテンプレートLoop.tmlを作成します
Loop.tml
<html t:type = "newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<p>This is sample parameter rendering example...</p>
<ol>
<li t:type = "loop" source = "1..5" value = "var:i">${var:i}</li>
</ol>
</html>
ループコンポーネントには次の2つのパラメータがあります-
source−コレクションソース。1…5は、指定された範囲の配列を作成するために使用されるプロパティ展開です。
var−レンダリング変数。テンプレートの本体に現在の値をレンダリングするために使用されます。
ページをリクエストすると、次のような結果が表示されます-
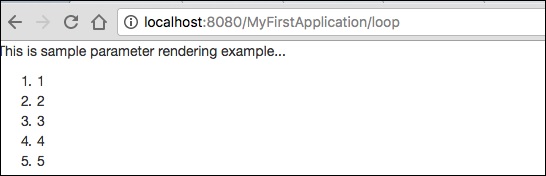
PageLinkコンポーネント
PageLinkコンポーネントは、あるページから別のページにページをリンクするために使用されます。以下のようにPageLinkテストページを作成します-PageLink.java。
package com.example.MyFirstApplication.pages;
public class PageLink {
}
次に、以下に示すように、対応するテンプレートファイルを作成します-
PageLink.tml
<html t:type = "newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<body>
<h3><u>Page Link</u> </h3>
<div class = "page">
<t:pagelink page = "Index">Click here to navigate Index page</t:pagelink>
<br/>
</div>
</body>
</html>
PageLinkコンポーネントには、ターゲットのタペストリーページを参照する必要があるページパラメータがあります。
Result − http:// localhost:8080 / myFirstApplication / pagelink
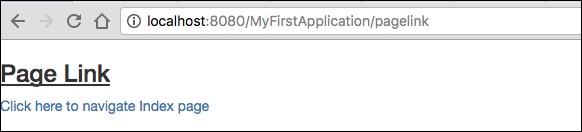
EventLinkコンポーネント
EventLinkコンポーネントは、URLを介してイベント名と対応するパラメーターを送信します。以下に示すように、EventsLinkページクラスを作成します。
EventsLink.java
package com.example.MyFirstApplication.pages;
import org.apache.tapestry5.annotations.Property;
public class EventsLink {
@Property
private int x;
void onActivate(int count) {
this.x = x;
}
int onPassivate() {
return x;
}
void onAdd(int value) {
x += value;
}
}
次に、対応する「EventsLink」テンプレートファイルを次のように作成します-
EventsLink.tml
<html t:type = "newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<h3> Event link example </h3>
AddedCount = ${x}. <br/>
<t:eventlink t:event = "add" t:context = "literal:1">
Click here to add count
</t:eventlink><br/>
</html>
EventLinkには次の2つのパラメータがあります-
Event−EventLinkコンポーネントでトリガーされるイベントの名前。デフォルトでは、コンポーネントのIDを指します。
Context−これはオプションのパラメータです。リンクのコンテキストを定義します。
Result − http:// localhost:8080 / myFirstApplication / EventsLink

カウント値をクリックすると、次の出力スクリーンショットに示すように、ページにURLにイベント名が表示されます。

ActionLinkコンポーネント
ActionLinkコンポーネントはEventLinkコンポーネントに似ていますが、ターゲットコンポーネントIDのみを送信します。デフォルトのイベント名はactionです。
以下に示すように、「ActivationLinks.java」ページを作成します。
ActivationLinks.java
package com.example.MyFirstApplication.pages;
import org.apache.tapestry5.annotations.Property;
public class ActivationLinks {
@Property
private int x;
void onActivate(int count) {
this.x = x;
}
int onPassivate() {
return x;
}
void onActionFromsub(int value) {
x -= value;
}
}
次に、以下に示すように、対応するテンプレートファイルを作成します-
ActivationLinks.tml
<html t:type = "Newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<div class = "div1">
Count = ${count}. <br/>
<t:actionlink t:id = "sub" t:context = "literal:1">
Decrement
</t:actionlink><br/>
</div>
</html>
ここでは、 OnActionFromSub ActionLinkコンポーネントをクリックすると、メソッドが呼び出されます。
Result − http:// localhost:8080 / myFirstApplication / ActivationsLink
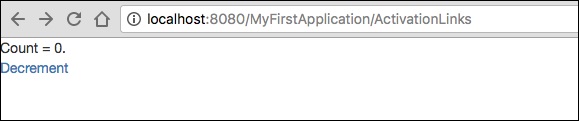
アラートコンポーネント
警告ダイアログボックスは、主にユーザーに警告メッセージを表示するために使用されます。たとえば、入力フィールドに必須のテキストが必要であるが、ユーザーが入力を提供しない場合、検証の一環として、アラートボックスを使用して警告メッセージを表示できます。
次のプログラムに示すように、「アラート」ページを作成します。
Alerts.java
package com.example.MyFirstApplication.pages;
public class Alerts {
public String getUser() {
return "user1";
}
}
次に、対応するテンプレートファイルを次のように作成します-
Alerts.tml
<html t:type = "Newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<h3>Alerts</h3>
<div class = "alert alert-info">
<h5> Welcome ${user} </h5>
</div>
</html>
アラートには3つの重大度レベルがあります。
- Info
- Warn
- Error
上記のテンプレートは、情報アラートを使用して作成されています。それは次のように定義されていますalert-info。必要に応じて、他の重大度を作成できます。
ページをリクエストすると、次の結果が生成されます-
http://localhost:8080/myFirstApplication/Alerts
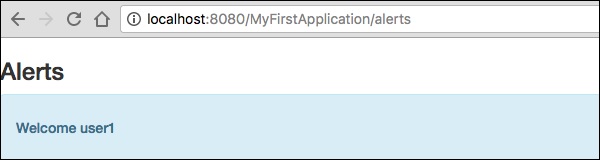