フォームと検証コンポーネント
ザ・ Form Componentタペストリーページにユーザー入力用のフォームを作成するために使用されます。フォームには、テキストフィールド、日付フィールド、チェックボックスフィールド、選択オプション、送信ボタンなどを含めることができます。
この章では、いくつかの注目すべきフォームコンポーネントについて詳しく説明します。
チェックボックスコンポーネント
チェックボックスコンポーネントは、相互に排他的な2つのオプションから選択するために使用されます。以下に示すように、チェックボックスを使用してページを作成します-
Checkbox.java
package com.example.MyFirstApplication.pages;
import org.apache.tapestry5.annotations.Property;
public class Checkbox {
@Property
private boolean check1;
@Property
private boolean check2;
}
次に、対応するテンプレートを作成します Checkbox.tml 以下に示すように-
<html t:type = "newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<h3> checkbox component</h3>
<t:form>
<t:checkbox t:id = "check1"/> I have a bike <br/>
<t:checkbox t:id = "check2"/> I have a car
</t:form>
</html>
ここで、チェックボックスパラメータIDは対応するブール値と一致します。
Result −ページhttp:// localhost:8080 / myFirstApplication / checkboxをリクエストすると、次の結果が生成されます。

TextFieldコンポーネント
TextFieldコンポーネントを使用すると、ユーザーは1行のテキストを編集できます。ページを作成Text 以下に示すように。
Text.java
package com.example.MyFirstApplication.pages;
import org.apache.tapestry5.annotations.Property;
import org.apache.tapestry5.corelib.components.TextField;public class Text {
@Property
private String fname;
@Property
private String lname;
}
次に、以下に示すように対応するテンプレートを作成します– Text.tml
<html t:type = "newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<p> Form application </p>
<body>
<h3> Text field created from Tapestry component </h3>
<t:form>
<table>
<tr>
<td>
Firstname: </td> <td><t:textfield t:id = "fname" />
</td>
<td>Lastname: </td> <td> <t:textfield t:id = "lname" /> </td>
</tr>
</table>
</t:form>
</body>
</html>
ここで、テキストページにはという名前のプロパティが含まれています fname そして lname。コンポーネントIDは、プロパティによってアクセスされます。
ページをリクエストすると、次の結果が生成されます-
http://localhost:8080/myFirstApplication/Text
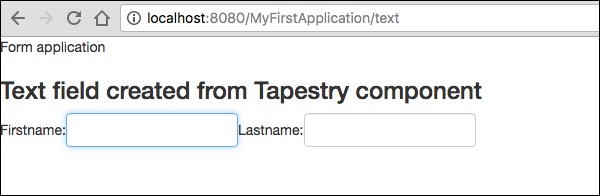
PasswordFieldコンポーネント
PasswordFieldは、パスワード専用のテキストフィールドエントリです。以下に示すようにページパスワードを作成します-
Password.java
package com.example.MyFirstApplication.pages;
import org.apache.tapestry5.annotations.Property;
import org.apache.tapestry5.corelib.components.PasswordField;
public class Password {
@Property
private String pwd;
}
ここで、対応するテンプレートファイルを次のように作成します-
Password.tml
<html t:type = "newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<p> Form application </p>
<h3> Password field created from Tapestry component </h3>
<t:form>
<table>
<tr>
<td> Password: </td>
<td><t:passwordfield t:id = "pwd"/> </td>
</tr>
</table>
</t:form>
</html>
ここで、PasswordFieldコンポーネントには、プロパティを指すパラメーターidがあります。 pwd。ページをリクエストすると、次の結果が生成されます-
http://localhost:8080/myFirstApplication/Password

TextAreaコンポーネント
TextAreaコンポーネントは、複数行の入力テキストコントロールです。以下に示すように、ページTxtAreaを作成します。
TxtArea.java
package com.example.MyFirstApplication.pages;
import org.apache.tapestry5.annotations.Property;
import org.apache.tapestry5.corelib.components.TextArea;
public class TxtArea {
@Property
private String str;
}
次に、対応するテンプレートファイルを以下のように作成します。
TxtArea.tml
<html t:type = "newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<h3>TextArea component </h3>
<t:form>
<table>
<tr>
<td><t:textarea t:id = "str"/>
</td>
</tr>
</table>
</t:form>
</html>
ここで、TextAreaコンポーネントのパラメータidはプロパティ「str」を指しています。ページをリクエストすると、次の結果が生成されます-
http://localhost:8080/myFirstApplication/TxtArea**
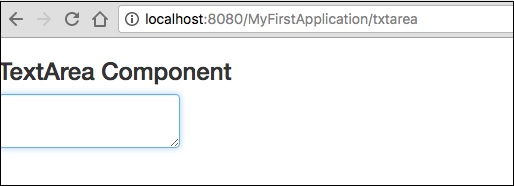
コンポーネントを選択
選択コンポーネントには、選択肢のドロップダウンリストが含まれています。以下に示すように、ページSelectOptionを作成します。
SelectOption.java
package com.example.MyFirstApplication.pages;
import org.apache.tapestry5.annotations.Property;
import org.apache.tapestry5.corelib.components.Select;
public class SelectOption {
@Property
private String color0;
@Property
private Color1 color1;
public enum Color1 {
YELLOW, RED, GREEN, BLUE, ORANGE
}
}
次に、対応するテンプレートを次のように作成します-
SelectOption.tml
<html t:type = "newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<p> Form application </p>
<h3> select component </h3>
<t:form>
<table>
<tr>
<td> Select your color here: </td>
<td> <select t:type = "select" t:id = "color1"></select></td>
</tr>
</table>
</t:form>
</html>
ここで、Selectコンポーネントには2つのパラメーターがあります-
Type −プロパティのタイプは列挙型です。
Id − idは、Tapestryプロパティ「color1」を指します。
ページをリクエストすると、次の結果が生成されます-
http://localhost:8080/myFirstApplication/SelectOption

RadioGroupコンポーネント
RadioGroupコンポーネントは、Radioコンポーネントのコンテナグループを提供します。RadioコンポーネントとRadioGroupコンポーネントは連携して、オブジェクトのプロパティを更新します。このコンポーネントは、他の無線コンポーネントをラップアラウンドする必要があります。以下に示すように、新しいページ「Radiobutton.java」を作成します-
Radiobutton.java
package com.example.MyFirstApplication.pages;
import org.apache.tapestry5.PersistenceConstants;
import org.apache.tapestry5.annotations.Persist;
import org.apache.tapestry5.annotations.Property;
public class Radiobutton {
@Property
@Persist(PersistenceConstants.FLASH)
private String value;
}
次に、対応するテンプレートファイルを次のように作成します-
Radiobutton.tml
<html t:type = "Newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<h3>RadioGroup component </h3>
<t:form>
<t:radiogroup t:id = "value">
<t:radio t:id = "radioT" value = "literal:T" label = "Male" />
<t:label for = "radioT"/>
<t:radio t:id = "radioF" value = "literal:F" label = "Female"/>
<t:label for = "radioF"/>
</t:radiogroup>
</t:form>
</html>
ここで、RadioGroupコンポーネントIDはプロパティ「value」とバインドしています。ページをリクエストすると、次の結果が得られます。
http://localhost:8080/myFirstApplication/Radiobutton
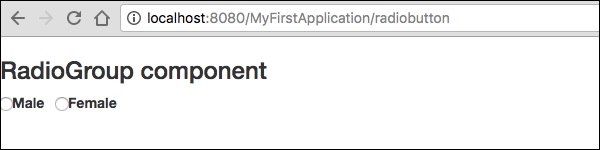
コンポーネントを送信
ユーザーが送信ボタンをクリックすると、タグのアクション設定で指定されたアドレスにフォームが送信されます。ページを作成SubmitComponent 以下に示すように。
package com.example.MyFirstApplication.pages;
import org.apache.tapestry5.annotations.InjectPage;
public class SubmitComponent {
@InjectPage
private Index page1;
Object onSuccess() {
return page1;
}
}
次に、以下に示すように、対応するテンプレートファイルを作成します。
SubmitComponent.tml
<html t:type = "newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<h3>Tapestry Submit component </h3>
<body>
<t:form>
<t:submit t:id = "submit1" value = "Click to go Index"/>
</t:form>
</body>
</html>
ここで、Submitコンポーネントは、値をIndexページに送信します。ページをリクエストすると、次の結果が生成されます-
http://localhost:8080/myFirstApplication/SubmitComponent
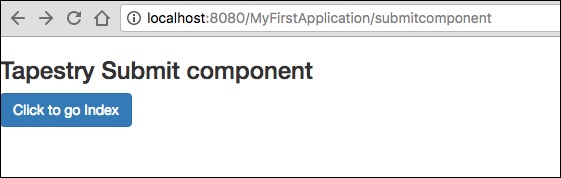
フォームの検証
フォームの検証は通常、クライアントが必要なすべてのデータを入力してフォームを送信した後、サーバーで行われます。クライアントによって入力されたデータが正しくないか、単に欠落している場合、サーバーはすべてのデータをクライアントに送り返し、フォームに正しい情報を再送信するように要求する必要があります。
検証のプロセスを理解するために、次の簡単な例を考えてみましょう。
ページを作成 Validate 以下に示すように。
Validate.java
package com.example.MyFirstApplication.pages;
import org.apache.tapestry5.annotations.Property;
import org.apache.tapestry5.PersistenceConstants;
import org.apache.tapestry5.annotations.Persist;
public class Validate {
@Property
@Persist(PersistenceConstants.FLASH)
private String firstName;
@Property
@Persist(PersistenceConstants.FLASH)
private String lastName;
}
次に、以下に示すように、対応するテンプレートファイルを作成します。
Validate.tml
<html t:type = "newlayout" title = "About MyFirstApplication"
xmlns:t = "http://tapestry.apache.org/schema/tapestry_5_4.xsd"
xmlns:p = "tapestry:parameter">
<t:form>
<table>
<tr>
<td><t:label for = "firstName"/>:</td>
<td><input t:type = "TextField" t:id = "firstName"
t:validate = "required, maxlength = 7" size = "10"/></td>
</tr>
<tr>
<td><t:label for = "lastName"/>:</td>
<td><input t:type = "TextField" t:id = "lastName"
t:validate = "required, maxLength = 5" size = "10"/></td>
</tr>
</table>
<t:submit t:id = "sub" value =" Form validation"/>
</t:form>
</html>
フォーム検証には、次の重要なパラメータがあります-
Max −は、最大値を定義します。たとえば、=«最大値、20»です。
MaxDate− maxDateを定義します。たとえば、=«最大日付、2013年6月9日»です。同様に、MinDateを割り当てることもできます。
MaxLength − maxLength for eg =«最大長、80»。
Min −最小。
MinLength −例の最小の長さ=«最小の長さ、2»。
Email −標準の電子メール正規表現^ \ w [._ \ w] * \ w @ \ w [-._ \ w] * \ w \。\ w2,6 $またはnoneを使用する電子メール検証。
ページをリクエストすると、次の結果が生成されます-
http://localhost:8080/myFirstApplication/Validate
