Flutter - Viết mã cụ thể cho Android
Flutter cung cấp một khuôn khổ chung để truy cập tính năng cụ thể của nền tảng. Điều này cho phép nhà phát triển mở rộng chức năng của khung Flutter bằng cách sử dụng mã nền tảng cụ thể. Chức năng cụ thể của nền tảng như máy ảnh, mức pin, trình duyệt, v.v., có thể được truy cập dễ dàng thông qua khung.
Ý tưởng chung về việc truy cập mã cụ thể của nền tảng là thông qua giao thức nhắn tin đơn giản. Mã rung, Máy khách và mã nền tảng và Máy chủ liên kết với một Kênh thông báo chung. Máy khách gửi tin nhắn đến Máy chủ thông qua Kênh thông báo. Máy chủ lắng nghe trên Kênh thông báo, nhận thông báo và thực hiện các chức năng cần thiết và cuối cùng, trả kết quả cho Máy khách thông qua Kênh thông báo.
Kiến trúc mã nền tảng cụ thể được hiển thị trong sơ đồ khối dưới đây:

Giao thức nhắn tin sử dụng codec tin nhắn chuẩn (lớp StandardMessageCodec) hỗ trợ tuần tự hóa nhị phân các giá trị giống JSON như số, chuỗi, boolean, v.v., Tuần tự hóa và hủy tuần tự hóa hoạt động minh bạch giữa máy khách và máy chủ.
Hãy để chúng tôi viết một ứng dụng đơn giản để mở trình duyệt bằng Android SDK và hiểu cách
Tạo ứng dụng Flutter mới trong Android studio, Flutter_browser_app
Thay thế mã main.dart bằng mã dưới đây -
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatelessWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(this.title),
),
body: Center(
child: RaisedButton(
child: Text('Open Browser'),
onPressed: null,
),
),
);
}
}
Ở đây, chúng tôi đã tạo một nút mới để mở trình duyệt và đặt phương thức onPressed của nó là null.
Bây giờ, nhập các gói sau:
import 'dart:async';
import 'package:flutter/services.dart';
Ở đây, services.dart bao gồm chức năng gọi mã nền tảng cụ thể.
Tạo một kênh tin nhắn mới trong tiện ích MyHomePage.
static const platform = const
MethodChannel('flutterapp.tutorialspoint.com/browser');
Viết một phương thức, _openBrowser để gọi phương thức nền tảng cụ thể, phương thức openBrowser thông qua kênh tin nhắn.
Future<void> _openBrowser() async {
try {
final int result = await platform.invokeMethod(
'openBrowser', <String, String>{
'url': "https://flutter.dev"
}
);
}
on PlatformException catch (e) {
// Unable to open the browser
print(e);
}
}
Ở đây, chúng tôi đã sử dụng platform.invokeMethod để gọi openBrowser (được giải thích trong các bước tiếp theo). openBrowser có một đối số, url để mở một url cụ thể.
Thay đổi giá trị của thuộc tính onPressed của RaisedButton từ null thành _openBrowser.
onPressed: _openBrowser,
Mở MainActivity.java (bên trong thư mục android) và nhập thư viện bắt buộc -
import android.app.Activity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import io.flutter.app.FlutterActivity;
import io.flutter.plugin.common.MethodCall;
import io.flutter.plugin.common.MethodChannel;
import io.flutter.plugin.common.MethodChannel.MethodCallHandler;
import io.flutter.plugin.common.MethodChannel.Result;
import io.flutter.plugins.GeneratedPluginRegistrant;
Viết phương thức, openBrowser để mở trình duyệt
private void openBrowser(MethodCall call, Result result, String url) {
Activity activity = this;
if (activity == null) {
result.error("ACTIVITY_NOT_AVAILABLE",
"Browser cannot be opened without foreground
activity", null);
return;
}
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse(url));
activity.startActivity(intent);
result.success((Object) true);
}
Bây giờ, đặt tên kênh trong lớp MainActivity -
private static final String CHANNEL = "flutterapp.tutorialspoint.com/browser";
Viết mã cụ thể cho android để đặt xử lý tin nhắn trong phương thức onCreate -
new MethodChannel(getFlutterView(), CHANNEL).setMethodCallHandler(
new MethodCallHandler() {
@Override
public void onMethodCall(MethodCall call, Result result) {
String url = call.argument("url");
if (call.method.equals("openBrowser")) {
openBrowser(call, result, url);
} else {
result.notImplemented();
}
}
});
Ở đây, chúng ta đã tạo một kênh thông báo bằng cách sử dụng lớp MethodChannel và sử dụng lớp MethodCallHandler để xử lý thông báo. onMethodCall là phương thức thực tế chịu trách nhiệm gọi đúng mã nền tảng cụ thể bằng cách kiểm tra tin nhắn. Phương thức onMethodCall trích xuất url từ tin nhắn và sau đó chỉ gọi openBrowser khi lệnh gọi phương thức là openBrowser. Nếu không, nó trả về phương thức notImplemented.
Mã nguồn hoàn chỉnh của ứng dụng như sau:
main.dart
MainActivity.java
package com.tutorialspoint.flutterapp.flutter_browser_app;
import android.app.Activity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import io.flutter.app.FlutterActivity;
import io.flutter.plugin.common.MethodCall;
import io.flutter.plugin.common.MethodChannel.Result;
import io.flutter.plugins.GeneratedPluginRegistrant;
public class MainActivity extends FlutterActivity {
private static final String CHANNEL = "flutterapp.tutorialspoint.com/browser";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
GeneratedPluginRegistrant.registerWith(this);
new MethodChannel(getFlutterView(), CHANNEL).setMethodCallHandler(
new MethodCallHandler() {
@Override
public void onMethodCall(MethodCall call, Result result) {
String url = call.argument("url");
if (call.method.equals("openBrowser")) {
openBrowser(call, result, url);
} else {
result.notImplemented();
}
}
}
);
}
private void openBrowser(MethodCall call, Result result, String url) {
Activity activity = this; if (activity == null) {
result.error(
"ACTIVITY_NOT_AVAILABLE", "Browser cannot be opened without foreground activity", null
);
return;
}
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse(url));
activity.startActivity(intent);
result.success((Object) true);
}
}
main.dart
import 'package:flutter/material.dart';
import 'dart:async';
import 'package:flutter/services.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(
title: 'Flutter Demo Home Page'
),
);
}
}
class MyHomePage extends StatelessWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
static const platform = const MethodChannel('flutterapp.tutorialspoint.com/browser');
Future<void> _openBrowser() async {
try {
final int result = await platform.invokeMethod('openBrowser', <String, String>{
'url': "https://flutter.dev"
});
}
on PlatformException catch (e) {
// Unable to open the browser print(e);
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(this.title),
),
body: Center(
child: RaisedButton(
child: Text('Open Browser'),
onPressed: _openBrowser,
),
),
);
}
}
Chạy ứng dụng và nhấp vào nút Mở Trình duyệt và bạn có thể thấy rằng trình duyệt đã được khởi chạy. Ứng dụng Trình duyệt - Trang chủ như được hiển thị trong ảnh chụp màn hình ở đây -
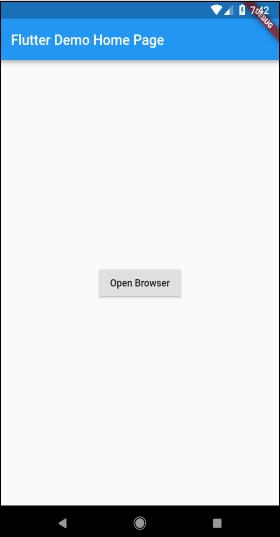
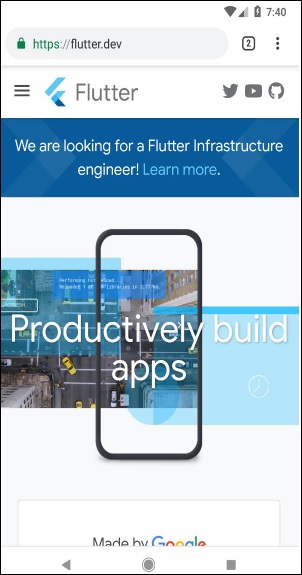