Spring MVC - Hibernate Validator Example
L'esempio seguente mostra come utilizzare la gestione degli errori e i convalidatori nei moduli che utilizzano il framework Spring Web MVC. Per cominciare, disponiamo di un IDE Eclipse funzionante e aderiamo ai seguenti passaggi per sviluppare un'applicazione Web basata su modulo dinamico utilizzando Spring Web Framework.
Passo | Descrizione |
---|---|
1 | Crea un progetto con il nome TestWeb sotto un pacchetto com.tutorialspoint come spiegato nel capitolo Spring MVC - Hello World. |
2 | Crea classi Java Student, StudentController e StudentValidator nel pacchetto com.tutorialspoint. |
3 | Crea file di visualizzazione addStudent.jsp e result.jsp nella sottocartella jsp. |
4 | Scarica la libreria di Hibernate Validator Hibernate Validator . Estrai hibernate-validator-5.3.4.Final.jar e le dipendenze richieste presenti nella cartella richiesta del file zip scaricato. Inseriscili nel tuo CLASSPATH. |
5 | Crea un file delle proprietà messages.properties nella cartella SRC. |
6 | Il passaggio finale è creare il contenuto dei file sorgente e di configurazione ed esportare l'applicazione come spiegato di seguito. |
Student.java
package com.tutorialspoint;
import org.hibernate.validator.constraints.NotEmpty;
import org.hibernate.validator.constraints.Range;
public class Student {
@Range(min = 1, max = 150)
private Integer age;
@NotEmpty
private String name;
private Integer id;
public void setAge(Integer age) {
this.age = age;
}
public Integer getAge() {
return age;
}
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setId(Integer id) {
this.id = id;
}
public Integer getId() {
return id;
}
}
StudentController.java
package com.tutorialspoint;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.validation.BindingResult;
import org.springframework.validation.annotation.Validated;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.servlet.ModelAndView;
@Controller
public class StudentController {
@RequestMapping(value = "/addStudent", method = RequestMethod.GET)
public ModelAndView student() {
return new ModelAndView("addStudent", "command", new Student());
}
@ModelAttribute("student")
public Student createStudentModel() {
return new Student();
}
@RequestMapping(value = "/addStudent", method = RequestMethod.POST)
public String addStudent(@ModelAttribute("student") @Validated Student student,
BindingResult bindingResult, Model model) {
if (bindingResult.hasErrors()) {
return "addStudent";
}
model.addAttribute("name", student.getName());
model.addAttribute("age", student.getAge());
model.addAttribute("id", student.getId());
return "result";
}
}
messages.properties
NotEmpty.student.name = Name is required!
Range.student.age = Age value must be between 1 and 150!
Qui, la chiave è <Annotation>. <object-name>. <attribute>. Il valore è il messaggio da visualizzare.
TestWeb-servlet.xml
<beans xmlns = "http://www.springframework.org/schema/beans"
xmlns:context = "http://www.springframework.org/schema/context"
xmlns:mvc = "http://www.springframework.org/schema/mvc"
xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation = "
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd">
<context:component-scan base-package = "com.tutorialspoint" />
<mvc:annotation-driven />
<bean class = "org.springframework.context.support.ResourceBundleMessageSource"
id = "messageSource">
<property name = "basename" value = "messages" />
</bean>
<bean class = "org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name = "prefix" value = "/WEB-INF/jsp/" />
<property name = "suffix" value = ".jsp" />
</bean>
</beans>
Qui, per il primo metodo di servizio student(), abbiamo superato uno spazio vuoto Studentobject>nell'oggetto ModelAndView con il nome "comando", perché il framework spring si aspetta un oggetto con il nome "comando", se si utilizzano i tag <form: form> nel file JSP. Quindi, quando il filestudent() metodo viene chiamato, ritorna addStudent.jsp Visualizza.
Il secondo metodo di servizio addStudent() verrà chiamato contro un metodo POST su HelloWeb/addStudentURL. Preparerai il tuo oggetto modello in base alle informazioni inviate. Infine, verrà restituita una vista "risultato" dal metodo del servizio, che risulterà nel rendering di result.jsp. In caso di errori generati utilizzando il validatore, viene restituita la stessa vista "addStudent", Spring inietta automaticamente i messaggi di erroreBindingResult in vista.
addStudent.jsp
<%@taglib uri = "http://www.springframework.org/tags/form" prefix = "form"%>
<html>
<head>
<title>Spring MVC Form Handling</title>
</head>
<style>
.error {
color: #ff0000;
}
.errorblock {
color: #000;
background-color: #ffEEEE;
border: 3px solid #ff0000;
padding: 8px;
margin: 16px;
}
</style>
<body>
<h2>Student Information</h2>
<form:form method = "POST" action = "/TestWeb/addStudent" commandName = "student">
<form:errors path = "*" cssClass = "errorblock" element = "div" />
<table>
<tr>
<td><form:label path = "name">Name</form:label></td>
<td><form:input path = "name" /></td>
<td><form:errors path = "name" cssClass = "error" /></td>
</tr>
<tr>
<td><form:label path = "age">Age</form:label></td>
<td><form:input path = "age" /></td>
<td><form:errors path = "age" cssClass = "error" /></td>
</tr>
<tr>
<td><form:label path = "id">id</form:label></td>
<td><form:input path = "id" /></td>
</tr>
<tr>
<td colspan = "2">
<input type = "submit" value = "Submit"/>
</td>
</tr>
</table>
</form:form>
</body>
</html>
Qui stiamo usando il tag <form: errors /> con path = "*" per visualizzare i messaggi di errore. Ad esempio:
<form:errors path = "*" cssClass = "errorblock" element = "div" />
Renderà i messaggi di errore per tutte le convalide di input. Stiamo utilizzando il tag <form: errors /> con path = "name" per visualizzare il messaggio di errore per il campo del nome.
Ad esempio:
<form:errors path = "name" cssClass = "error" />
<form:errors path = "age" cssClass = "error" />
Renderà i messaggi di errore per le convalide del campo nome e età.
result.jsp
<%@taglib uri = "http://www.springframework.org/tags/form" prefix = "form"%>
<html>
<head>
<title>Spring MVC Form Handling</title>
</head>
<body>
<h2>Submitted Student Information</h2>
<table>
<tr>
<td>Name</td>
<td>${name}</td>
</tr>
<tr>
<td>Age</td>
<td>${age}</td>
</tr>
<tr>
<td>ID</td>
<td>${id}</td>
</tr>
</table>
</body>
</html>
Una volta terminata la creazione dei file sorgente e di configurazione, esporta l'applicazione. Fai clic destro sulla tua applicazione, usaExport → WAR File opzione e salva il file HelloWeb.war file nella cartella webapps di Tomcat.
Ora, avvia il server Tomcat e assicurati di essere in grado di accedere ad altre pagine web dalla cartella webapps utilizzando un browser standard. Prova un URL -http://localhost:8080/TestWeb/addStudent e vedremo la seguente schermata, se hai inserito valori non validi.
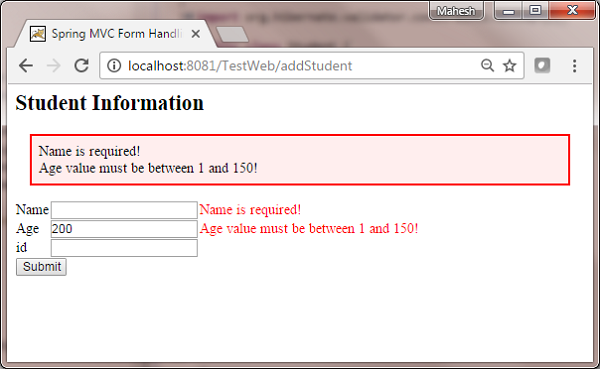