Yii-コントローラー
コントローラーは、要求の処理と応答の生成を担当します。ユーザーのリクエスト後、コントローラーはリクエストデータを分析し、モデルに渡し、モデルの結果をビューに挿入して、応答を生成します。
アクションを理解する
コントローラにはアクションが含まれます。これらは、ユーザーが実行を要求できる基本単位です。コントローラは、1つまたは複数のアクションを持つことができます。
見てみましょう SiteController 基本的なアプリケーションテンプレートの
<?php
namespace app\controllers;
use Yii;
use yii\filters\AccessControl;
use yii\web\Controller;
use yii\filters\VerbFilter;
use app\models\LoginForm;
use app\models\ContactForm;
class SiteController extends Controller {
public function behaviors() {
return [
'access' => [
'class' => AccessControl::className(),
'only' => ['logout'],
'rules' => [
[
'actions' => ['logout'],
'allow' => true,
'roles' => ['@'],
],
],
],
'verbs' => [
'class' => VerbFilter::className(),
'actions' => [
'logout' => ['post'],
],
],
];
}
public function actions() {
return [
'error' => [
'class' => 'yii\web\ErrorAction',
],
'captcha' => [
'class' => 'yii\captcha\CaptchaAction',
'fixedVerifyCode' => YII_ENV_TEST ? 'testme' : null,
],
];
}
public function actionIndex() {
return $this->render('index');
}
public function actionLogin() {
if (!\Yii::$app->user->isGuest) {
return $this->goHome();
}
$model = new LoginForm();
if ($model->load(Yii::$app->request->post()) && $model->login()) {
return $this->goBack();
}
return $this->render('login', [
'model' => $model,
]);
}
public function actionLogout() {
Yii::$app->user->logout();
return $this->goHome();
}
public function actionContact() {
//load ContactForm model
$model = new ContactForm();
//if there was a POST request, then try to load POST data into a model
if ($model->load(Yii::$app->request->post()) && $model>contact(Yii::$app->params
['adminEmail'])) {
Yii::$app->session->setFlash('contactFormSubmitted');
return $this->refresh();
}
return $this->render('contact', [
'model' => $model,
]);
}
public function actionAbout() {
return $this->render('about');
}
public function actionSpeak($message = "default message") {
return $this->render("speak",['message' => $message]);
}
}
?>
PHP組み込みサーバーを使用して基本的なアプリケーションテンプレートを実行し、次のWebブラウザにアクセスします。 http://localhost:8080/index.php?r=site/contact。次のページが表示されます-

このページを開くと、 SiteController実行されます。コードは最初にContactFormモデル。次に、連絡先ビューをレンダリングし、モデルをそのビューに渡します。
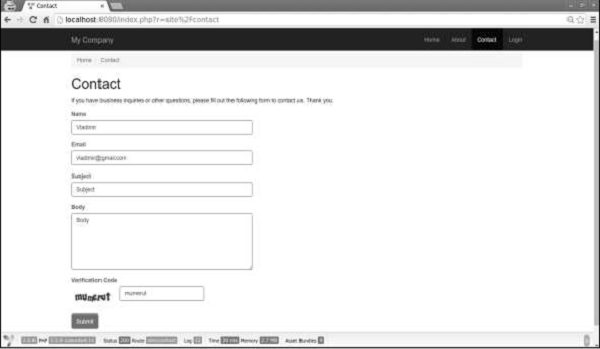
フォームに入力して送信ボタンをクリックすると、次のように表示されます。
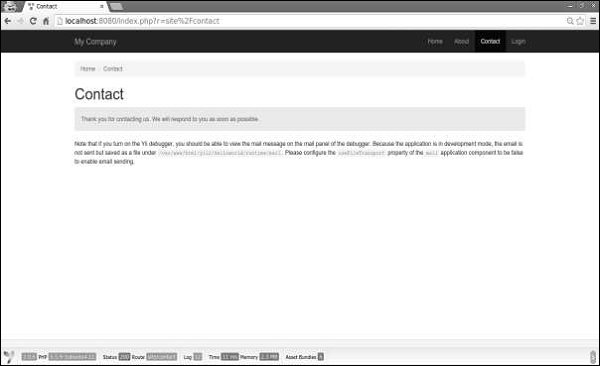
今回は次のコードが実行されることに注意してください-
if ($model->load(Yii::$app->request->post()) && $model->contact(Yii::$app>params ['adminEmail'])) {
Yii::$app->session->setFlash('contactFormSubmitted');
return $this->refresh();
}
POSTリクエストがあった場合は、POSTデータをモデルに割り当てて、メールを送信しようとします。成功した場合は、「お問い合わせいただきありがとうございます」というテキストを含むフラッシュメッセージを設定します。できるだけ早く対応させていただきます。」ページを更新します。
ルートを理解する
上記の例では、URLで、 http://localhost:8080/index.php?r=site/contact, ルートは site/contact。接触アクション(actionContact) の中に SiteController 実行されます。
ルートは次の部分で構成されています-
moduleID −コントローラがモジュールに属している場合、ルートのこの部分が存在します。
controllerID (上記の例のサイト)-同じモジュールまたはアプリケーション内のすべてのコントローラーの中でコントローラーを識別する一意の文字列。
actionID (上記の例の連絡先)-同じコントローラー内のすべてのアクションの中でアクションを識別する一意の文字列。
ルートの形式は次のとおりです。 controllerID/actionID。コントローラがモジュールに属している場合、その形式は次のとおりです。moduleID/controllerID/actionID。