Angular7 - Template
Angular 7 menggunakan <ng-template> sebagai tag, bukan <template> yang digunakan di Angular2. <ng-template> telah digunakan sejak rilis Angular 4, dan versi sebelumnya yaitu Angular 2 menggunakan <template> untuk tujuan yang sama. Alasan ia mulai menggunakan <ng-template> daripada <template> dari Angular 4 dan seterusnya adalah karena ada konflik nama antara tag <template> dan tag standar <template> html. Ini akan benar-benar tidak berlaku lagi. Ini adalah salah satu perubahan besar yang dibuat pada versi Angular 4.
Sekarang mari kita gunakan template bersama dengan if else condition dan lihat hasilnya.
app.component.html
<!--The content below is only a placeholder and can be replaced.-->
<div style = "text-align:center">
<h1>Welcome to {{title}}.</h1>
</div>
<div> Months :
<select (change) = "changemonths($event)" name = "month">
<option *ngFor = "let i of months">{{i}}</option>
</select>
</div>
<br/>
<div>
<span *ngIf = "isavailable;then condition1 else condition2">
Condition is valid.
</span>
<ng-template #condition1>Condition is valid from template</ng-template>
<ng-template #condition2>Condition is invalid from template</ng-template>
</div>
<button (click) = "myClickFunction($event)">Click Me</button>
Untuk tag Span, kami telah menambahkan if pernyataan dengan else condition dan akan memanggil template condition1, else condition2.
Templatenya akan dipanggil sebagai berikut -
<ng-template #condition1>Condition is valid from template</ng-template>
<ng-template #condition2>Condition is invalid from template</ng-template>
Jika kondisinya benar, maka condition1 template dipanggil, jika tidak condition2.
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular 7';
// declared array of months.
months = ["January", "February", "March", "April", "May", "June", "July",
"August", "September", "October", "November", "December"];
isavailable = false; // variable is set to true
myClickFunction(event) {
//just added console.log which will display the event details in browser on click of the button.
alert("Button is clicked");
console.log(event);
}
changemonths(event) {
alert("Changed month from the Dropdown");
}
}
Output di browser adalah sebagai berikut -
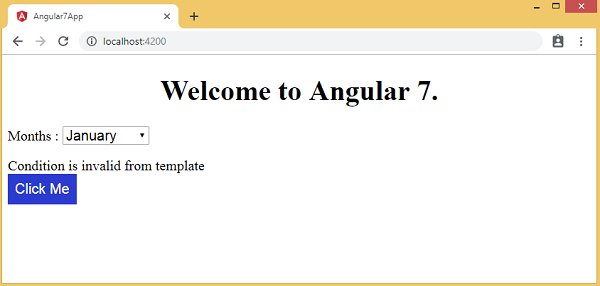
Variabel isavailablesalah sehingga template condition2 dicetak. Jika Anda mengklik tombol tersebut, template terkait akan dipanggil.
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular 7';
// declared array of months.
months = ["January", "Feburary", "March", "April", "May", "June", "July",
"August", "September", "October", "November", "December"];
isavailable = false; //variable is set to true
myClickFunction(event) {
this.isavailable = !this.isavailable;
// variable is toggled onclick of the button
}
changemonths(event) {
alert("Changed month from the Dropdown");
}
}
Itu isavailable variabel diaktifkan dengan mengklik tombol seperti yang ditunjukkan di bawah ini -
myClickFunction(event) {
this.isavailable = !this.isavailable;
}
Ketika Anda mengklik tombol berdasarkan nilai dari isavailable variabel template masing-masing akan ditampilkan -
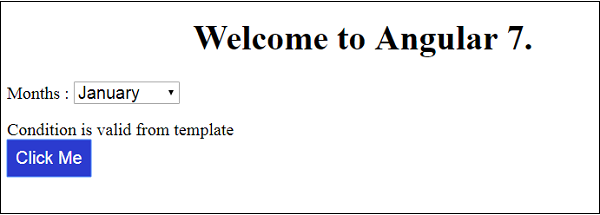

Jika Anda memeriksa browser, Anda akan melihat bahwa Anda tidak pernah mendapatkan tag span di dom. Contoh berikut akan membantu Anda memahami hal yang sama.

Meskipun dalam app.component.html kami telah menambahkan tag span dan <ng-template> untuk kondisi seperti gambar dibawah ini -
<span *ngIf = "isavailable;then condition1 else condition2">
Condition is valid.
</span>
<ng-template #condition1>Condition is valid from template</ng-template>
<ng-template #condition2>Condition is invalid from template</ng-template>
Kami tidak melihat tag span dan juga <ng-template> dalam struktur dom saat kami memeriksa hal yang sama di browser.
Baris kode berikut di html akan membantu kita mendapatkan tag span di dom -
<!--The content below is only a placeholder and can be replaced.-->
<div style = "text-align:center">
<h1> Welcome to {{title}}. </h1>
</div>
<div> Months :
<select (change) = "changemonths($event)" name = "month">
<option *ngFor = "let i of months">{{i}}</option>
</select>
</div>
<br/>
<div>
<span *ngIf = "isavailable; else condition2">
Condition is valid.
</span>
<ng-template #condition1>Condition is valid from template </ng-template>
<ng-template #condition2>Condition is invalid from template</ng-template>
</div>
<button (click) = "myClickFunction($event)">Click Me</button>
Jika kami menghapus thenkondisi, kita mendapatkan pesan "Kondisi valid" di browser dan tag span juga tersedia di dom. Misalnya, diapp.component.ts, kami telah membuat isavailable variabel sebagai benar.
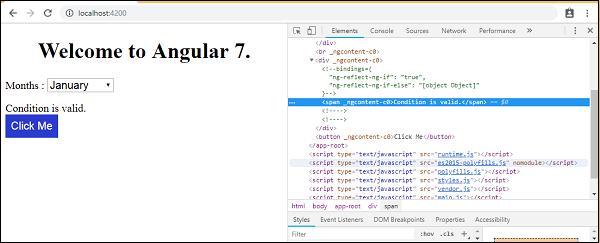