Windows10 Dev - обмен данными между приложениями
Связь между приложениями означает, что ваше приложение может общаться или взаимодействовать с другим приложением, установленным на том же устройстве. Это не новая функция в приложении универсальной платформы Windows (UWP), она также была доступна в Windows 8.1.
В Windows 10 представлены некоторые новые и улучшенные способы упрощения взаимодействия между приложениями на одном устройстве. Связь между двумя приложениями может быть следующими:
- Одно приложение запускает другое приложение с некоторыми данными.
- Приложения просто обмениваются данными, ничего не запуская.
Основное преимущество связи между приложениями состоит в том, что вы можете разбивать приложения на более мелкие части, которые можно легко поддерживать, обновлять и использовать.
Подготовка вашего приложения
Если вы выполните следующие действия, другие приложения смогут запустить ваше приложение.
Добавьте объявление протокола в манифест пакета приложения.
Дважды щелкните значок Package.appxmanifest файл, который доступен в обозревателе решений, как показано ниже.
Перейти к Declaration вкладка и напишите имя протокола, как показано ниже.
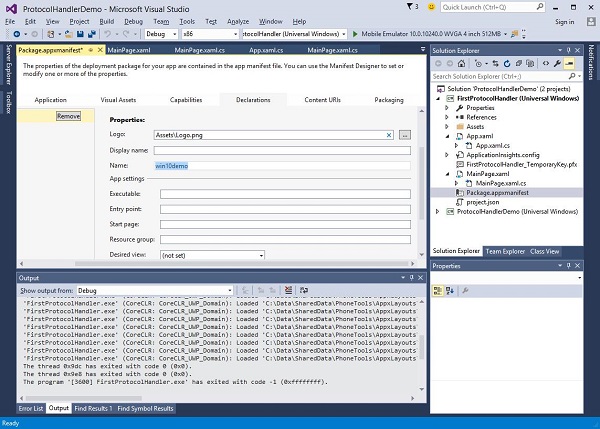
Следующим шагом будет добавление activation код, чтобы приложение могло должным образом реагировать при запуске другим приложением.
Чтобы ответить на активацию протокола, нам нужно переопределить OnActivatedметод класса активации. Итак, добавьте следующий код вApp.xaml.cs файл.
protected override void OnActivated(IActivatedEventArgs args) {
ProtocolActivatedEventArgs protocolArgs = args as ProtocolActivatedEventArgs;
if (args != null){
Frame rootFrame = Window.Current.Content as Frame;
// Do not repeat app initialization when the Window already has content,
// just ensure that the window is active
if (rootFrame == null){
// Create a Frame to act as the navigation context and navigate to the first page
rootFrame = new Frame();
// Set the default language
rootFrame.Language = Windows.Globalization.ApplicationLanguages.Languages[0];
rootFrame.NavigationFailed += OnNavigationFailed;
// Place the frame in the current Window
Window.Current.Content = rootFrame;
}
if (rootFrame.Content == null){
// When the navigation stack isn't restored, navigate to the
// first page, configuring the new page by passing required
// information as a navigation parameter
rootFrame.Navigate(typeof(MainPage), null);
}
// Ensure the current window is active
Window.Current.Activate();
}
}
Для запуска приложения вы можете просто использовать Launcher.LaunchUriAsync , который запустит приложение с протоколом, указанным в этом методе.
await Windows.System.Launcher.LaunchUriAsync(new Uri("win10demo:?SomeData=123"));
Давайте разберемся в этом на простом примере, в котором у нас есть два приложения UWP с ProtocolHandlerDemo и FirstProtocolHandler.
В этом примере ProtocolHandlerDemo приложение содержит одну кнопку, и при нажатии на нее откроется FirstProtocolHandler применение.
Код XAML в приложении ProtocolHandlerDemo, который содержит одну кнопку, приведен ниже.
<Page
x:Class = "ProtocolHandlerDemo.MainPage"
xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local = "using:ProtocolHandlerDemo"
xmlns:d = "http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable = "d">
<Grid Background = "{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Button x:Name = "LaunchButton" Content = " Launch First Protocol App"
FontSize = "24" HorizontalAlignment = "Center"
Click = "LaunchButton_Click"/>
</Grid>
</Page>
Ниже приведен код C #, в котором реализовано событие нажатия кнопки.
using System;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
// The Blank Page item template is documented at
http://go.microsoft.com/fwlink/?LinkId=402352&clcid=0x409
namespace ProtocolHandlerDemo {
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class MainPage : Page {
public MainPage(){
this.InitializeComponent();
}
private async void LaunchButton_Click(object sender, RoutedEventArgs e) {
await Windows.System.Launcher.LaunchUriAsync(new
Uri("win10demo:?SomeData=123"));
}
}
}
Теперь давайте посмотрим на FirstProtocolHandlerтаблица приложений. Ниже приведен код XAML, в котором текстовый блок создается с некоторыми свойствами.
<Page
x:Class = "FirstProtocolHandler.MainPage"
xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local = "using:FirstProtocolHandler"
xmlns:d = "http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable = "d">
<Grid Background = "{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock Text = "You have successfully launch First Protocol Application"
TextWrapping = "Wrap" Style = "{StaticResource SubtitleTextBlockStyle}"
Margin = "30,39,0,0" VerticalAlignment = "Top" HorizontalAlignment = "Left"
Height = "100" Width = "325"/>
</Grid>
</Page>
Реализация C # App.xaml.cs файл, в котором OnActicatedпереопределено показано ниже. Добавьте следующий код внутри класса App вApp.xaml.cs файл.
protected override void OnActivated(IActivatedEventArgs args) {
ProtocolActivatedEventArgs protocolArgs = args as ProtocolActivatedEventArgs;
if (args != null) {
Frame rootFrame = Window.Current.Content as Frame;
// Do not repeat app initialization when the Window already has content,
// just ensure that the window is active
if (rootFrame == null) {
// Create a Frame to act as the navigation context and navigate to
the first page
rootFrame = new Frame();
// Set the default language
rootFrame.Language = Windows.Globalization.ApplicationLanguages.Languages[0];
rootFrame.NavigationFailed += OnNavigationFailed;
// Place the frame in the current Window
Window.Current.Content = rootFrame;
}
if (rootFrame.Content == null) {
// When the navigation stack isn't restored navigate to the
// first page, configuring the new page by passing required
// information as a navigation parameter
rootFrame.Navigate(typeof(MainPage), null);
}
// Ensure the current window is active
Window.Current.Activate();
}
}
Когда вы компилируете и выполняете ProtocolHandlerDemo приложения на эмуляторе, вы увидите следующее окно.
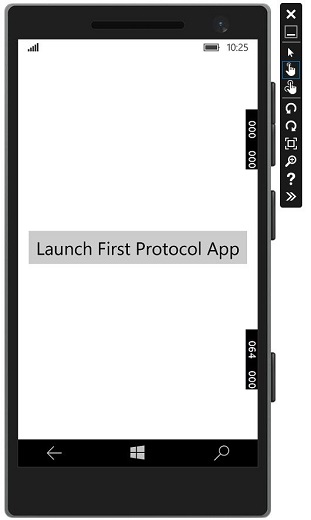
Теперь, когда вы нажмете на кнопку, откроется FirstProtocolHandler приложение, как показано ниже.
