Angular7 - เทมเพลต
Angular 7 ใช้ <ng-template> เป็นแท็กแทน <template> ซึ่งใช้ใน Angular2 <ng-template> ถูกใช้งานตั้งแต่รุ่น Angular 4 และรุ่นก่อนหน้าเช่น Angular 2 ใช้ <template> เพื่อจุดประสงค์เดียวกัน เหตุผลที่เริ่มใช้ <ng-template> แทน <template> ตั้งแต่ Angular 4 เป็นต้นไปเนื่องจากมีความขัดแย้งของชื่อระหว่างแท็ก <template> และแท็กมาตรฐาน html <template> จะเลิกใช้งานอย่างสมบูรณ์ในอนาคต นี่เป็นการเปลี่ยนแปลงที่สำคัญอย่างหนึ่งที่เกิดขึ้นในเวอร์ชัน Angular 4
ให้เราใช้เทมเพลตพร้อมกับไฟล์ if else condition และดูผลลัพธ์
app.component.html
<!--The content below is only a placeholder and can be replaced.-->
<div style = "text-align:center">
<h1>Welcome to {{title}}.</h1>
</div>
<div> Months :
<select (change) = "changemonths($event)" name = "month">
<option *ngFor = "let i of months">{{i}}</option>
</select>
</div>
<br/>
<div>
<span *ngIf = "isavailable;then condition1 else condition2">
Condition is valid.
</span>
<ng-template #condition1>Condition is valid from template</ng-template>
<ng-template #condition2>Condition is invalid from template</ng-template>
</div>
<button (click) = "myClickFunction($event)">Click Me</button>
สำหรับแท็ก Span เราได้เพิ่มไฟล์ if คำสั่งกับ else condition และจะเรียก template condition1, else condition2
เทมเพลตจะถูกเรียกดังต่อไปนี้ -
<ng-template #condition1>Condition is valid from template</ng-template>
<ng-template #condition2>Condition is invalid from template</ng-template>
หากเงื่อนไขเป็นจริงแสดงว่า condition1 เรียกเทมเพลตมิฉะนั้น condition2.
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular 7';
// declared array of months.
months = ["January", "February", "March", "April", "May", "June", "July",
"August", "September", "October", "November", "December"];
isavailable = false; // variable is set to true
myClickFunction(event) {
//just added console.log which will display the event details in browser on click of the button.
alert("Button is clicked");
console.log(event);
}
changemonths(event) {
alert("Changed month from the Dropdown");
}
}
ผลลัพธ์ในเบราว์เซอร์มีดังนี้ -
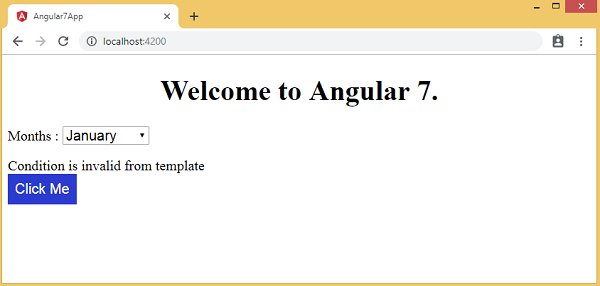
ตัวแปร isavailableเป็นเท็จดังนั้นเทมเพลต condition2 จึงถูกพิมพ์ หากคุณคลิกปุ่มจะมีการเรียกเทมเพลตตามลำดับ
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular 7';
// declared array of months.
months = ["January", "Feburary", "March", "April", "May", "June", "July",
"August", "September", "October", "November", "December"];
isavailable = false; //variable is set to true
myClickFunction(event) {
this.isavailable = !this.isavailable;
// variable is toggled onclick of the button
}
changemonths(event) {
alert("Changed month from the Dropdown");
}
}
isavailable ตัวแปรจะถูกสลับเมื่อคลิกปุ่มดังที่แสดงด้านล่าง -
myClickFunction(event) {
this.isavailable = !this.isavailable;
}
เมื่อคุณคลิกที่ปุ่มตามค่าของ isavailable ตัวแปรแม่แบบที่เกี่ยวข้องจะปรากฏขึ้น -
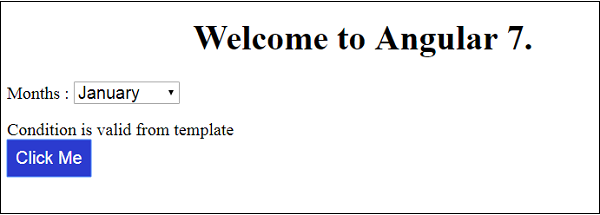

หากคุณตรวจสอบเบราว์เซอร์คุณจะเห็นว่าคุณไม่เคยได้รับแท็ก span ในโดเมน ตัวอย่างต่อไปนี้จะช่วยให้คุณเข้าใจสิ่งเดียวกัน

แม้ว่าใน app.component.html เราได้เพิ่มแท็กช่วงและไฟล์ <ng-template> สำหรับเงื่อนไขดังแสดงด้านล่าง -
<span *ngIf = "isavailable;then condition1 else condition2">
Condition is valid.
</span>
<ng-template #condition1>Condition is valid from template</ng-template>
<ng-template #condition2>Condition is invalid from template</ng-template>
เราไม่เห็นแท็ก span และ <ng-template> ในโครงสร้าง dom เมื่อเราตรวจสอบสิ่งเดียวกันในเบราว์เซอร์
โค้ดบรรทัดต่อไปนี้ใน html จะช่วยให้เราได้รับแท็ก span ในโดเมน -
<!--The content below is only a placeholder and can be replaced.-->
<div style = "text-align:center">
<h1> Welcome to {{title}}. </h1>
</div>
<div> Months :
<select (change) = "changemonths($event)" name = "month">
<option *ngFor = "let i of months">{{i}}</option>
</select>
</div>
<br/>
<div>
<span *ngIf = "isavailable; else condition2">
Condition is valid.
</span>
<ng-template #condition1>Condition is valid from template </ng-template>
<ng-template #condition2>Condition is invalid from template</ng-template>
</div>
<button (click) = "myClickFunction($event)">Click Me</button>
หากเราลบไฟล์ thenเงื่อนไขเราได้รับข้อความ "เงื่อนไขถูกต้อง" ในเบราว์เซอร์และแท็ก span ก็มีอยู่ในโดเมน ตัวอย่างเช่นในapp.component.tsเราได้สร้างไฟล์ isavailable ตัวแปรเป็นจริง
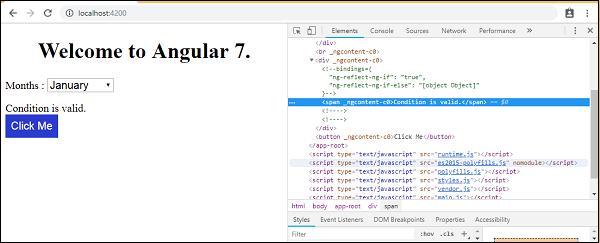