Spring MVC - ตัวอย่างการอัปโหลดไฟล์
ตัวอย่างต่อไปนี้แสดงวิธีใช้ File Upload Control ในฟอร์มโดยใช้ Spring Web MVC framework ในการเริ่มต้นให้เรามี Eclipse IDE ที่ใช้งานได้และปฏิบัติตามขั้นตอนต่อไปนี้เพื่อพัฒนา Web Application ที่ใช้ Dynamic Form โดยใช้ Spring Web Framework
ขั้นตอน | คำอธิบาย |
---|---|
1 | สร้างโปรเจ็กต์ด้วยชื่อ HelloWeb ภายใต้แพ็คเกจ com.tutorialspoint ตามที่อธิบายไว้ในบท Spring MVC - Hello World |
2 | สร้างคลาส Java FileModel, FileUploadController ภายใต้แพ็กเกจ com.tutorialspoint |
3 | สร้างไฟล์ดู fileUpload.jsp, success.jsp ภายใต้โฟลเดอร์ย่อย jsp |
4 | สร้างโฟลเดอร์ temp ภายใต้โฟลเดอร์ย่อย WebContent |
5 | ดาวน์โหลด Apache Commons FileUpload ห้องสมุดคอมมอน-fileupload.jarและ Apache Commons IO ห้องสมุดคอมมอน-io.jar ใส่ไว้ใน CLASSPATH ของคุณ |
6 | ขั้นตอนสุดท้ายคือการสร้างเนื้อหาของไฟล์ต้นทางและการกำหนดค่าและส่งออกแอปพลิเคชันตามที่อธิบายด้านล่าง |
FileModel.java
package com.tutorialspoint;
import org.springframework.web.multipart.MultipartFile;
public class FileModel {
private MultipartFile file;
public MultipartFile getFile() {
return file;
}
public void setFile(MultipartFile file) {
this.file = file;
}
}
FileUploadController.java
package com.tutorialspoint;
import java.io.File;
import java.io.IOException;
import javax.servlet.ServletContext;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.util.FileCopyUtils;
import org.springframework.validation.BindingResult;
import org.springframework.validation.annotation.Validated;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.servlet.ModelAndView;
@Controller
public class FileUploadController {
@Autowired
ServletContext context;
@RequestMapping(value = "/fileUploadPage", method = RequestMethod.GET)
public ModelAndView fileUploadPage() {
FileModel file = new FileModel();
ModelAndView modelAndView = new ModelAndView("fileUpload", "command", file);
return modelAndView;
}
@RequestMapping(value="/fileUploadPage", method = RequestMethod.POST)
public String fileUpload(@Validated FileModel file, BindingResult result, ModelMap model) throws IOException {
if (result.hasErrors()) {
System.out.println("validation errors");
return "fileUploadPage";
} else {
System.out.println("Fetching file");
MultipartFile multipartFile = file.getFile();
String uploadPath = context.getRealPath("") + File.separator + "temp" + File.separator;
//Now do something with file...
FileCopyUtils.copy(file.getFile().getBytes(), new File(uploadPath+file.getFile().getOriginalFilename()));
String fileName = multipartFile.getOriginalFilename();
model.addAttribute("fileName", fileName);
return "success";
}
}
}
HelloWeb-servlet.xml
<beans xmlns = "http://www.springframework.org/schema/beans"
xmlns:context = "http://www.springframework.org/schema/context"
xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation = "
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd">
<context:component-scan base-package = "com.tutorialspoint" />
<bean class = "org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name = "prefix" value = "/WEB-INF/jsp/" />
<property name = "suffix" value = ".jsp" />
</bean>
<bean id = "multipartResolver"
class = "org.springframework.web.multipart.commons.CommonsMultipartResolver" />
</beans>
นี่คือวิธีการบริการแรก fileUploadPage()เราได้ผ่านช่องว่าง FileModelออบเจ็กต์ในออบเจ็กต์ ModelAndView ที่มีชื่อ "command" เนื่องจากสปริงเฟรมเวิร์กต้องการอ็อบเจ็กต์ที่มีชื่อ "command" ถ้าคุณใช้แท็ก <form: form> ในไฟล์ JSP ดังนั้นเมื่อfileUploadPage() เรียกว่าเมธอดมันส่งกลับ fileUpload.jsp ดู.
วิธีการบริการที่สอง fileUpload() จะถูกเรียกใช้เมธอด POST บนไฟล์ HelloWeb/fileUploadPageURL คุณจะเตรียมไฟล์ที่จะอัปโหลดตามข้อมูลที่ส่งมา สุดท้ายมุมมอง "ความสำเร็จ" จะถูกส่งกลับจากวิธีการบริการซึ่งจะส่งผลให้การแสดงผล success.jsp
fileUpload.jsp
<%@ page contentType="text/html; charset = UTF-8" %>
<%@ taglib prefix = "form" uri = "http://www.springframework.org/tags/form"%>
<html>
<head>
<title>File Upload Example</title>
</head>
<body>
<form:form method = "POST" modelAttribute = "fileUpload"
enctype = "multipart/form-data">
Please select a file to upload :
<input type = "file" name = "file" />
<input type = "submit" value = "upload" />
</form:form>
</body>
</html>
ที่นี่เรากำลังใช้ modelAttribute แอตทริบิวต์ที่มีค่า = "fileUpload" เพื่อแมปการควบคุมการอัปโหลดไฟล์กับโมเดลเซิร์ฟเวอร์
success.jsp
<%@ page contentType = "text/html; charset = UTF-8" %>
<html>
<head>
<title>File Upload Example</title>
</head>
<body>
FileName :
lt;b> ${fileName} </b> - Uploaded Successfully.
</body>
</html>
เมื่อคุณสร้างไฟล์ซอร์สและไฟล์คอนฟิกเสร็จเรียบร้อยแล้วให้ส่งออกแอปพลิเคชันของคุณ คลิกขวาที่แอปพลิเคชันของคุณใช้Export → WAR File และบันทึกไฟล์ HelloWeb.war ในโฟลเดอร์ webapps ของ Tomcat
ตอนนี้เริ่มเซิร์ฟเวอร์ Tomcat ของคุณและตรวจสอบให้แน่ใจว่าคุณสามารถเข้าถึงหน้าเว็บอื่น ๆ จากโฟลเดอร์ webapps โดยใช้เบราว์เซอร์มาตรฐาน ลองใช้ URL–http://localhost:8080/HelloWeb/fileUploadPage และเราจะเห็นหน้าจอต่อไปนี้หากทุกอย่างเรียบร้อยดีกับ Spring Web Application
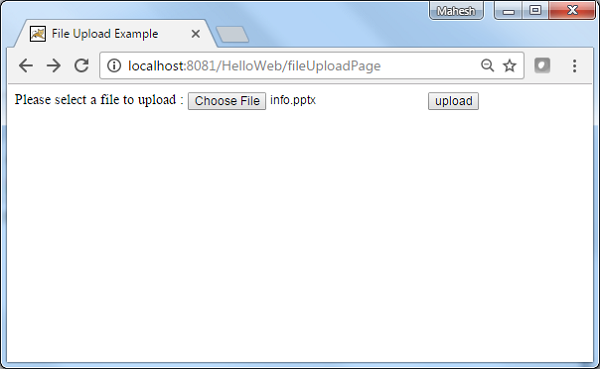
หลังจากส่งข้อมูลที่จำเป็นแล้วให้คลิกที่ปุ่มส่งเพื่อส่งแบบฟอร์ม คุณควรเห็นหน้าจอต่อไปนี้หากทุกอย่างเรียบร้อยดีกับ Spring Web Application
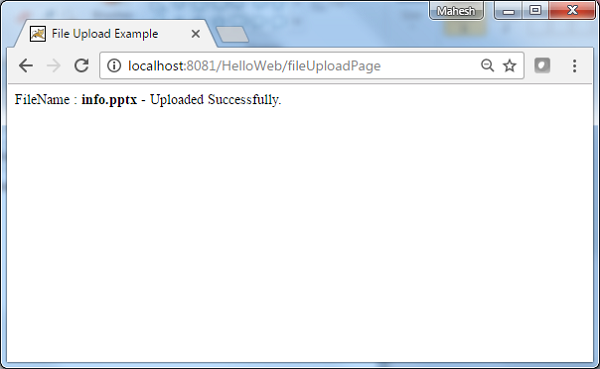