Angular7 - Modelos
Angular 7 usa o <ng-template> como tag em vez de <template>, que é usado em Angular2. <ng-template> está em uso desde o lançamento do Angular 4, e a versão anterior, ou seja, Angular 2 usa <template> para o mesmo propósito. A razão pela qual começou a usar <ng-template> em vez de <template> a partir do Angular 4 é porque há um conflito de nomes entre a tag <template> e a tag padrão html <template>. Ele será completamente suspenso daqui para frente. Esta foi uma das principais mudanças feitas na versão Angular 4.
Vamos agora usar o modelo junto com o if else condition e veja a saída.
app.component.html
<!--The content below is only a placeholder and can be replaced.-->
<div style = "text-align:center">
<h1>Welcome to {{title}}.</h1>
</div>
<div> Months :
<select (change) = "changemonths($event)" name = "month">
<option *ngFor = "let i of months">{{i}}</option>
</select>
</div>
<br/>
<div>
<span *ngIf = "isavailable;then condition1 else condition2">
Condition is valid.
</span>
<ng-template #condition1>Condition is valid from template</ng-template>
<ng-template #condition2>Condition is invalid from template</ng-template>
</div>
<button (click) = "myClickFunction($event)">Click Me</button>
Para a tag Span, adicionamos o if declaração com o else condição e chamará o modelo condição1, caso contrário, condição2
Os modelos devem ser chamados da seguinte forma -
<ng-template #condition1>Condition is valid from template</ng-template>
<ng-template #condition2>Condition is invalid from template</ng-template>
Se a condição for verdadeira, então o condition1 template é chamado, caso contrário condition2.
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular 7';
// declared array of months.
months = ["January", "February", "March", "April", "May", "June", "July",
"August", "September", "October", "November", "December"];
isavailable = false; // variable is set to true
myClickFunction(event) {
//just added console.log which will display the event details in browser on click of the button.
alert("Button is clicked");
console.log(event);
}
changemonths(event) {
alert("Changed month from the Dropdown");
}
}
A saída no navegador é a seguinte -
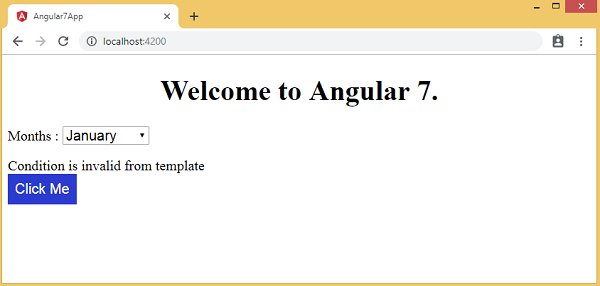
A variável isavailableé falso, então o modelo da condição2 é impresso. Se você clicar no botão, o respectivo modelo será chamado.
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular 7';
// declared array of months.
months = ["January", "Feburary", "March", "April", "May", "June", "July",
"August", "September", "October", "November", "December"];
isavailable = false; //variable is set to true
myClickFunction(event) {
this.isavailable = !this.isavailable;
// variable is toggled onclick of the button
}
changemonths(event) {
alert("Changed month from the Dropdown");
}
}
o isavailable a variável é alternada ao clicar no botão, conforme mostrado abaixo -
myClickFunction(event) {
this.isavailable = !this.isavailable;
}
Quando você clica no botão com base no valor do isavailable variável o respectivo modelo será exibido -
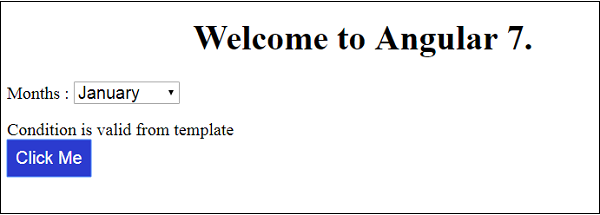

Se você inspecionar o navegador, verá que nunca obterá a tag span no dom. O exemplo a seguir ajudará você a entender o mesmo.

Embora em app.component.html adicionamos a tag span e o <ng-template> para a condição conforme mostrado abaixo -
<span *ngIf = "isavailable;then condition1 else condition2">
Condition is valid.
</span>
<ng-template #condition1>Condition is valid from template</ng-template>
<ng-template #condition2>Condition is invalid from template</ng-template>
Não vemos a tag span e também o <ng-template> na estrutura dom quando inspecionamos o mesmo no navegador.
A seguinte linha de código em html nos ajudará a obter a tag span no dom -
<!--The content below is only a placeholder and can be replaced.-->
<div style = "text-align:center">
<h1> Welcome to {{title}}. </h1>
</div>
<div> Months :
<select (change) = "changemonths($event)" name = "month">
<option *ngFor = "let i of months">{{i}}</option>
</select>
</div>
<br/>
<div>
<span *ngIf = "isavailable; else condition2">
Condition is valid.
</span>
<ng-template #condition1>Condition is valid from template </ng-template>
<ng-template #condition2>Condition is invalid from template</ng-template>
</div>
<button (click) = "myClickFunction($event)">Click Me</button>
Se removermos o thencondição, obtemos a mensagem “A condição é válida” no navegador e a tag span também está disponível no dom. Por exemplo, emapp.component.ts, nós fizemos o isavailable variável como verdadeira.
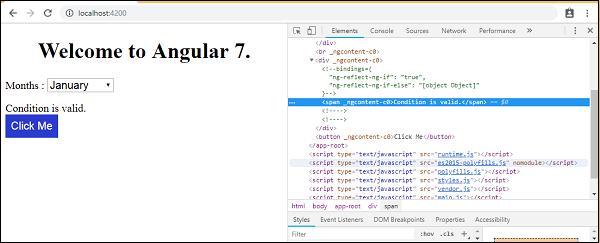