Windows10開発-データバインディング
データバインディングはXAMLアプリケーションのメカニズムであり、部分クラスを使用してWindowsランタイムアプリがデータを表示および操作するためのシンプルで簡単な方法を提供します。データの管理は、このメカニズムでのデータの表示方法から完全に分離されています。
データバインディングにより、UI要素とユーザーインターフェイス上のデータオブジェクト間のデータフローが可能になります。バインディングが確立され、データまたはビジネスモデルが変更されると、更新がUI要素に自動的に反映されます。その逆も同様です。標準のデータソースではなく、ページ上の別の要素にバインドすることもできます。データバインディングは次のようになります-
- 一方向のデータバインディング
- 双方向のデータバインディング
- 要素のバインド
一方向のデータバインディング
一方向バインディングでは、データはソース(データを保持するオブジェクト)からターゲット(データを表示するオブジェクト)にバインドされます。
一方向のデータバインディングの簡単な例を見てみましょう。以下に示すのは、いくつかのプロパティを使用して4つのテキストブロックが作成されるXAMLコードです。
<Page
x:Class = "OneWayDataBinding.MainPage"
xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local = "using:OneWayDataBinding"
xmlns:d = "http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable = "d">
<Grid Background = "{ThemeResource ApplicationPageBackgroundThemeBrush}">
<StackPanel Name = "Display">
<StackPanel Orientation = "Horizontal" Margin = "50, 50, 0, 0">
<TextBlock Text = "Name: " Margin = "10" Width = "100"/>
<TextBlock Margin = "10" Width = "100" Text = "{Binding Name}"/>
</StackPanel>
<StackPanel Orientation = "Horizontal" Margin = "50,0,50,0">
<TextBlock Text = "Title: " Margin = "10" Width = "100"/>
<TextBlock Margin = "10" Width = "200" Text = "{Binding Title}" />
</StackPanel>
</StackPanel>
</Grid>
</Page>
2つのテキストブロックのテキストプロパティは次のように設定されます “Name” そして “Title” 静的に、テキストブロックの他の2つのTextプロパティは、以下に示すように、Employeeクラスのクラス変数である「Name」と「Title」にバインドされます。
using Windows.UI.Xaml.Controls;
// The Blank Page item template is documented at
http://go.microsoft.com/fwlink/?LinkId=402352&clcid=0x409
namespace OneWayDataBinding {
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class MainPage : Page {
public MainPage(){
this.InitializeComponent();
DataContext = Employee.GetEmployee();
}
}
public class Employee {
public string Name { get; set; }
public string Title { get; set; }
public static Employee GetEmployee() {
var emp = new Employee() {
Name = "Waqar Ahmed",
Title = "Development Manager"
};
return emp;
}
}
}
の中に Employee class、変数があります Name そして Title そして、1つの静的メソッド employee object初期化され、その従業員オブジェクトを返します。したがって、プロパティ、名前、およびタイトルにバインドしていますが、プロパティが属するオブジェクトはまだ選択されていません。簡単な方法は、オブジェクトをに割り当てることですDataContext、そのプロパティをバインドしています MainPage コンストラクタ。
このアプリケーションを実行すると、すぐにあなたので見ることができます MainWindow そのEmployeeオブジェクトのNameとTitleに正常にバインドしたこと。
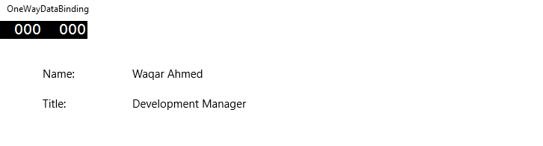
双方向データバインディング
双方向バインディングでは、ユーザーはユーザーインターフェイスを介してデータを変更し、そのデータをソースで更新することができます。たとえば、ユーザーがビューを見ているときにソースが変更された場合、ビューを更新する必要があります。
2つのラベル、2つのテキストボックス、および1つのボタンがいくつかのプロパティとイベントで作成されている以下の例を見てみましょう。
<Page
x:Class = "TwoWayDataBinding.MainPage"
xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local = "using:TwoWayDataBinding"
xmlns:d = "http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable = "d">
<Grid Background = "{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Grid.RowDefinitions>
<RowDefinition Height = "Auto" />
<RowDefinition Height = "Auto" />
<RowDefinition Height = "*" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width = "Auto" />
<ColumnDefinition Width = "200" />
</Grid.ColumnDefinitions>
<TextBlock Name = "nameLabel" Margin = "200,20,0,0">Name:</TextBlock>
<TextBox Name = "nameText" Grid.Column = "1" Margin = "10,20,0,0"
Text = "{Binding Name, Mode = TwoWay}"/>
<TextBlock Name = "ageLabel" Margin = "200,20,0,0"
Grid.Row = "1">Age:</TextBlock>
<TextBox Name = "ageText" Grid.Column = "1" Grid.Row = "1" Margin = "10,20,0,0"
Text = "{Binding Age, Mode = TwoWay}"/>
<StackPanel Grid.Row = "2" Grid.ColumnSpan = "2">
<Button Content = "Display" Click = "Button_Click"
Margin = "200,20,0,0"/>
<TextBlock x:Name = "txtblock" Margin = "200,20,0,0"/>
</StackPanel>
</Grid>
</Page>
以下を観察することができます-
両方のテキストボックスのテキストプロパティは、 "Name" そして "Age" のクラス変数です Person class 以下に示すように。
に Person class、NameとAgeの2つの変数があり、そのオブジェクトはで初期化されます。 MainWindow クラス。
XAMLコードでは、プロパティにバインドしています- Name そして Age、ただし、プロパティが属するオブジェクトは選択していません。
より簡単な方法は、オブジェクトをに割り当てることです。 DataContext、以下に示すように、C#コードでプロパティをバインドします。 MainWindowconstructor。
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
// The Blank Page item template is documented at
http://go.microsoft.com/fwlink/?LinkId=402352&clcid=0x409
namespace TwoWayDataBinding {
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class MainPage : Page {
Person person = new Person { Name = "Salman", Age = 26 };
public MainPage() {
this.InitializeComponent();
this.DataContext = person;
}
private void Button_Click(object sender, RoutedEventArgs e) {
string message = person.Name + " is " + person.Age + " years old";
txtblock.Text = message;
}
}
public class Person {
private string nameValue;
public string Name {
get { return nameValue; }
set { nameValue = value; }
}
private double ageValue;
public double Age {
get { return ageValue; }
set {
if (value != ageValue) {
ageValue = value;
}
}
}
}
}
上記のコードをコンパイルして実行すると、次のウィンドウが表示されます。クリックDisplay ボタン。

名前と年齢を変更して、 Display もう一度ボタンを押します。

あなたはクリックボタンでそれを見ることができます ‘Display’、テキストボックスのテキストは、上のデータを表示するために使用されません TextBlock ただし、クラス変数が使用されます。
理解を深めるために、両方のケースで上記のコードを実行することをお勧めします。
要素のバインド
標準のデータソースではなく、ページ上の別の要素にバインドすることもできます。というアプリケーションを作成しましょうElementBindingスライダーと長方形が作成され、スライダーを使用して、長方形の幅と高さがバインドされます。以下に、XAMLのコードを示します。
<Page
x:Class = "ElementBinding.MainPage"
xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local = "using:ElementBinding"
xmlns:d = "http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable = "d">
<Grid Background = "{ThemeResource ApplicationPageBackgroundThemeBrush}">
<StackPanel VerticalAlignment = "Center" HorizontalAlignment = "Center">
<Rectangle Height = "100" Width = "100" Fill = "SteelBlue"
RenderTransformOrigin = "0.5,0.5" Margin = "50">
<Rectangle.RenderTransform>
<CompositeTransform ScaleX = "{Binding Value, ElementName = MySlider}"
ScaleY = "{Binding Value, ElementName = MySlider}"/>
</Rectangle.RenderTransform>
</Rectangle>
<Slider Minimum = ".5" Maximum = "2.0" StepFrequency = ".1"
x:Name = "MySlider" />
</StackPanel>
</Grid>
</Page>
上記のコードをコンパイルして実行すると、次のウィンドウが表示されます。

スライダーを使用すると、以下に示すように長方形のサイズを変更できます。
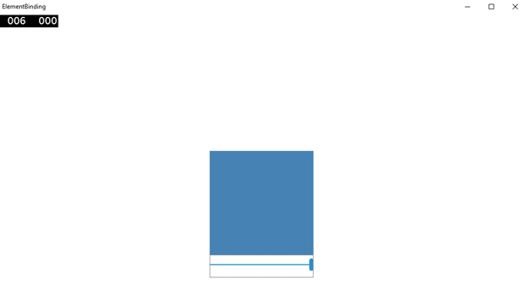